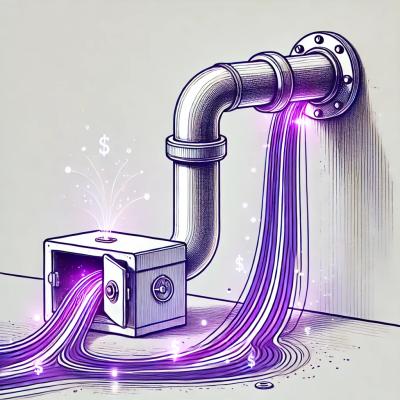
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
document-ts
Advanced tools
A very thin TypeScript-based async MongoDB helper with optional ODM convenience features
A lightweight TypeScript MongoDB ODM with standout convenience features like CollectionFactory
and findWithPagination
Read the excerpt from Angular for Enterprise on Understanding DocumentTS on the Wiki.
Looking to containerize MongoDB? Checkout excellalabs/mongo for a fully featured Mongo container (with Auth & SSL) inherited from the official Mongo Docker image and instructions on how to deploy it on AWS.
DocumentTS is an ODM (Object Document Mapper) for MongoDB.
connect()
MongoDB async connection harness
It can be a challenge to ensure that database connectivity exists, when writing a fully async web application. connect()
makes it easy to connect to a MongoDB instance and makes it safe to be called simultaneously from multiple threads starting up simultaneously.
Document
and IDocument
Base Class and Interface to help define your own models
CollectionFactory
Define collections, organize indexes, and aggregate queries alongside collection implementation. Below are the convenience features of a DocumentTS collection
get collection
returns the native MongoDB collection, so you can directly operate on itget collection(): ICollectionProvider<TDocument>
aggregate
allows you to run a MongoDB aggregation pipelineaggregate(pipeline: object[]): AggregationCursor<TDocument>
findOne
and findOneAndUpdate
simplifies the operation of commonly used database functionality, automatically hydrating the models it returnsasync findOne(filter: FilterQuery<TDocument>, options?: FindOneOptions)
async findOneAndUpdate(
filter: FilterQuery<TDocument>,
update: TDocument | UpdateQuery<TDocument>,
options?: FindOneAndReplaceOption
): Promise<TDocument | null>
findWithPagination
is by far the best feature of DocumentTS, allowing you to filter, sort, and paginate large collections of data. This function is geared towards use with data tables, so you specify searchable properties, turn off hydration, and use a debug feature to fine-tune your queries.async findWithPagination<TReturnType extends IDbRecord>(
queryParams: Partial<IQueryParameters> & object,
aggregationCursorFunc?: Func<AggregationCursor<TReturnType>>,
query?: string | object,
searchableProperties?: string[],
hydrate = true,
debugQuery = false
): Promise<IPaginationResult<TReturnType>>
Supports MongoDB v4+, Mongo Driver 3.3+ and TypeScript 3.7+
npm install document-ts mongodb
connect()
connectionRetryWait
(in seconds) and connectionRetryMax
to modify this behaviorisProd
and certFileUri
to connect using an SSL certificateimport { connect } from 'document-ts'
async function start() {
// If isProd is set to true and a .pem file is provided, SSL will be used to connect: i.e. connect(config.mongoUri, isProd, 'server/compose-ca.pem')
await connect(process.env.MONGO_URI)
}
start()
connect()
then you don't have to worry about having your Database Instance initialized during an asynchronous start-up sequence. getDbInstance
gives you access to the native MongoDB driver to perform custom functions like creating indexes.import { getDbInstance } from 'document-ts'
// assuming this is called within an async function
await dbInstance.collection('users').createIndexes([
{
key: {
displayName: 1,
},
},
{
key: {
email: 1,
},
unique: true,
},
])
See
tests\user.ts
for sample Model implementation
import { IDocument } from 'document-ts'
export interface IUser extends IDocument {
email: string;
firstName: string;
lastName: string;
role: string;
}
See
tests\user.ts
for sample Model implementation
import { Document } from 'document-ts'
export class User extends Document<IUser> implements IUser {
static collectionName = 'users'
private password: string
public email: string
public firstName: string
public lastName: string
public role: string
constructor(user?: IUser) {
super(User.collectionName, user)
}
...
}
getCalculatedPropertiesToInclude()
which will ensure that your get
properties that are "calculate" on the fly will be serialized when sending the model down to the client, but it will not be saved in the database. getCalculatedPropertiesToInclude(): string[]{
return ['fullName']
}
getPropertiesToExclude()
which will ensure that certain properties like passwords will not be serialized when sending the model down to the client, but it will still be saved in the database. getPropertiesToExclude(): string[]{
return ['password']
}
CollectionFactory
class, so that you can run Mongo queries without having to call getDbInstance
or specify the collection and TypeScript type name every time you run a query. CollectionFactory provides convenience functions like find
, findOne
, findOneAndUpdate
, findWithPagination
, and similar, while also handling hydration
tasks, such as serializing getters and child documents.import { CollectionFactory } from 'document-ts'
class UserCollectionFactory extends CollectionFactory<User> {
constructor(docType: typeof User) {
super(User.collectionName, docType, ['firstName', 'lastName', 'email'])
}
}
export let UserCollection = new UserCollectionFactory(User)
CollectionFactory
is powerful and flexible. In your custom class, you can implement MongoDB aggregate queries to run advanced join-like queries, geo queries, and whatever MongoDB supports. findWithPagination
itself is very powerful and will enable you to implement paginated dashboards with ease.
findWithPagination
leverage query parameters for pagination and configuration
export interface IQueryParameters {
filter?: string
skip?: number
limit?: number
sortKeyOrList?: string | Object[] | Object
projectionKeyOrList?: string | Object[] | Object
}
toJSON()
to customize serialization/hydration behavior or extend ISerializable
toJSON() {
let keys = Object.keys(this).concat(['fullAddress', 'localAddress'])
return Serialize(SerializationStrategy.JSON, this, keys)
}
toBSON()
to customize database serialization behavior or extend ISerializable
toBSON() {
let keys = Object.keys(this).concat(['fullAddress', 'localAddress'])
return Serialize(SerializationStrategy.BSON, this, keys)
}
toJSON() {
// drop a breakpoint here or console.log(this)
return super.toJSON()
}
toBSON() {
return super.toBSON()
}
See the Lemon Mart Server sample project for usage - https://github.com/duluca/lemon-mart-server
Not a full-fledged ODM or ORM replacement and doesn't aspire to be one like Mongoose or Camo. Databases are HARD. MongoDB took many years to mature, and Microsoft has been trying for a really long time to build a reliable ORM with Entity Framework, Mongoose and many other ODMs are ridden with bugs (no offense) when you push them beyond the basics. It takes great resources to deliver a solid data access experience, so with DocumentTS you can develop directly against MongoDB while enjoying some conveniences as you choose.
Although DocumentTS doesn't aspire to replace Mongoose or Camo, it most definitely is inspired by them in the way they've solved certain problems such as hydration. Check out the source code for those projects here:
npm install
npm test
FAQs
A very thin TypeScript-based async MongoDB helper with optional ODM convenience features
We found that document-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.