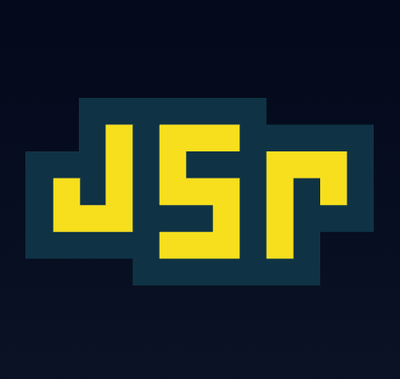
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
dom-helpers
Advanced tools
The dom-helpers package is a collection of utility functions for managing and interacting with the DOM. It provides a consistent and cross-browser way to perform common DOM manipulation tasks without relying on a larger library like jQuery.
Class Manipulation
Allows adding and removing CSS classes from DOM elements.
import { addClass, removeClass } from 'dom-helpers';
const element = document.getElementById('my-element');
addClass(element, 'new-class');
removeClass(element, 'old-class');
Events
Facilitates adding and removing event listeners to DOM elements.
import { on, off } from 'dom-helpers';
const handleClick = event => console.log('Clicked!', event);
const element = document.getElementById('my-button');
on(element, 'click', handleClick);
// Later on, to remove the event listener
off(element, 'click', handleClick);
Query
Provides a way to select DOM elements using CSS selectors.
import { querySelectorAll } from 'dom-helpers';
const items = querySelectorAll(document, '.list-item');
console.log(items);
Style
Enables getting and setting CSS styles on DOM elements.
import { css } from 'dom-helpers';
const element = document.getElementById('my-element');
css(element, { display: 'none' });
css(element, 'display', 'block');
Dimensions
Allows measuring and setting the dimensions of DOM elements.
import { height, width } from 'dom-helpers';
const element = document.getElementById('my-element');
console.log(height(element));
console.log(width(element));
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers. Compared to dom-helpers, jQuery is a more comprehensive tool but is also larger in size.
Zepto is a minimalist JavaScript library for modern browsers with a largely jQuery-compatible API. If you use jQuery, you will already be familiar with Zepto. While similar in API to dom-helpers, Zepto focuses on a jQuery-like experience in a smaller package.
Cash is an absurdly small jQuery alternative for modern browsers. It provides jQuery-style syntax for manipulating the DOM, handling events, and making AJAX requests. Cash-dom is more feature-rich than dom-helpers but still aims to be lightweight.
tiny modular DOM lib for ie8+
npm i -S dom-helpers
Mostly just naive wrappers around common DOM API inconsitencies, Cross browser work is minimal and mostly taken from jQuery. This library doesn't do a lot to normalize behavior across browsers, it mostly seeks to provide a common interface, and elminate the need to write the same damn if (ie8)
statements in every project.
For example events.on
works in all browsers ie8+ but it uses the native event system so actual event oddities will continue to exist. If you need robust cross-browser support use jQuery. if you are just tired of rewriting:
if (document.addEventListener)
return (node, eventName, handler, capture) =>
node.addEventListener(eventName, handler, capture || false);
else if (document.attachEvent)
return (node, eventName, handler) =>
node.attachEvent('on' + eventName, handler);
over and over again use this. Or you need a ok getComputedStyle
polyfill but don't want to include all of jquery, use this.
The real advantage to this collection is that any method can be required individually, meaning tools like Browserify or Webpack will only include the exact methods you use. This is great for environments where jQuery doesn't make sense, such as React
where you only occasionally need to do direct DOM manipulation.
Each level of the module can be required as a whole or you can drill down for a specific method or section:
var helpers = require('dom-helper')
var query = require('dom-helper/query')
var offset = require('dom-helper/query/offset')
// style is a function
require('dom-helper/style')(node, { width: '40px' })
//and a namespace
var gcs = require('dom-helper/style/getComputedStyle')
contains(container, element)
height(element, useClientHeight)
width(element, useClientWidth)
offset(element)
-> { top: Number, left: Number, top: height, width: Number}
scrollTop(element, [value])
scrollParent(element)
addClass(element, className)
removeClass(element, className)
hasClass(element, className)
style(element, propName, [value])
or style(element, objectOfPropValues)
removeStyle(element, styleName)
getComputedStyle(element)
-> getPropertyvalue(name)
end(node, handler, [duration])
listens for transition end, and ensures that the handler if called even if the transition fails to fire its end event. Will attempt to read duration from the element, otherwise one can be providedproperties
: Object containing the various vendor specifc transition and transform properties for your browser {
transform: // transform property: 'transform'
end: // transitionend
property: // transition-property
timing: // transition-timing
delay: // transition-delay
duration: // transition-duration
}
events
on(eventname, handler, [capture])
: capture is silently ignored in ie8off(eventname, handler, [capture])
: capture is silently ignored in ie8util
requestAnimationFrame
scrollTo
FAQs
tiny modular DOM lib for ie9+
We found that dom-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.