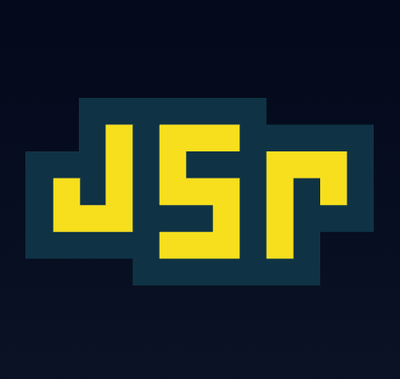
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
dom-serializer
Advanced tools
The dom-serializer package is used to serialize DOM nodes to a string representation, typically HTML or XML. It is useful for transforming the DOM tree into a textual format that can be saved, transmitted, or manipulated as a string.
Serializing DOM nodes to HTML
This feature allows you to serialize a DOM node into an HTML string. The code sample demonstrates how to serialize a simple DOM element using dom-serializer.
const serialize = require('dom-serializer');
const dom = require('domhandler');
const root = new dom.Element('div', { class: 'container' });
const serialized = serialize(root);
console.log(serialized); // Outputs: <div class="container"></div>
Custom formatting options
dom-serializer allows for custom formatting options such as xmlMode, decodeEntities, and selfClosingTags. This code sample shows how to serialize a DOM element with XML formatting.
const serialize = require('dom-serializer');
const dom = require('domhandler');
const root = new dom.Element('div', { class: 'container' });
const options = { xmlMode: true };
const serialized = serialize(root, options);
console.log(serialized); // Outputs: <div class="container"/>
parse5 is a fast full-featured HTML parsing and serialization library. It provides a variety of modules for parsing, serializing, and manipulating HTML documents. Compared to dom-serializer, parse5 offers a more comprehensive suite of HTML processing capabilities.
jsdom is a pure-JavaScript implementation of many web standards, notably the WHATWG DOM and HTML Standards. It creates a virtual DOM and is capable of serializing and manipulating it. While dom-serializer focuses on serialization, jsdom offers a broader range of features including DOM emulation, scripting, and event simulation.
htmlparser2 is a forgiving HTML and XML parser. It can also be used in conjunction with domhandler to create a DOM tree which can then be serialized. It is similar to dom-serializer in that it can be used to serialize DOM structures, but it also includes robust parsing capabilities.
Renders a domhandler DOM node or an array of domhandler DOM nodes to a string.
import render from "dom-serializer";
// OR
const render = require("dom-serializer").default;
render
▸ render(node
: Node | Node[], options?
: Options): string
Renders a DOM node or an array of DOM nodes to a string.
Can be thought of as the equivalent of the outerHTML
of the passed node(s).
Name | Type | Default value | Description |
---|---|---|---|
node | Node | Node[] | - | Node to be rendered. |
options | DomSerializerOptions | {} | Changes serialization behavior |
Returns: string
encodeEntities
• Optional
decodeEntities: boolean | "utf8"
Encode characters that are either reserved in HTML or XML.
If xmlMode
is true
or the value not 'utf8'
, characters outside of the utf8 range will be encoded as well.
default
decodeEntities
decodeEntities
• Optional
decodeEntities: boolean
Option inherited from parsing; will be used as the default value for encodeEntities
.
default
true
emptyAttrs
• Optional
emptyAttrs: boolean
Print an empty attribute's value.
default
xmlMode
example
With emptyAttrs: false
: <input checked>
example
With emptyAttrs: true
: <input checked="">
selfClosingTags
• Optional
selfClosingTags: boolean
Print self-closing tags for tags without contents.
default
xmlMode
example
With selfClosingTags: false
: <foo></foo>
example
With selfClosingTags: true
: <foo />
xmlMode
• Optional
xmlMode: boolean | "foreign"
Treat the input as an XML document; enables the emptyAttrs
and selfClosingTags
options.
If the value is "foreign"
, it will try to correct mixed-case attribute names.
default
false
Name | Description |
---|---|
htmlparser2 | Fast & forgiving HTML/XML parser |
domhandler | Handler for htmlparser2 that turns documents into a DOM |
domutils | Utilities for working with domhandler's DOM |
css-select | CSS selector engine, compatible with domhandler's DOM |
cheerio | The jQuery API for domhandler's DOM |
dom-serializer | Serializer for domhandler's DOM |
LICENSE: MIT
FAQs
render domhandler DOM nodes to a string
We found that dom-serializer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.