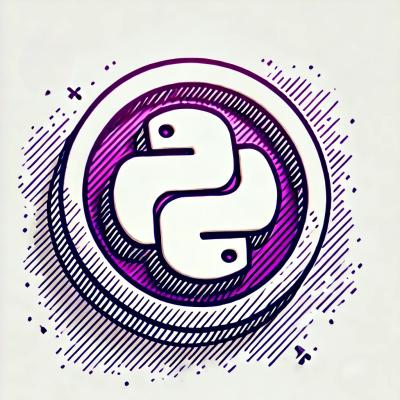
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Create views as components using DOM. Run the same code on the browser and on the server.
Create views as components using DOM. Run the same code on the browser and on the server.
This is a library that helps you create your html documents using plain javascript, instead of using a template language. No need to learn yet another language with new syntax. Using domv you can easily break up your page design into smaller components and reuse them in other projects.
In most templating languages reusability is hard because once the html is generated, you can no longer modify it easily. In domv, each component you create or use is a proper OOP class which can be subclassed or modified by calling methods or using setters. After you have set up all your components you can serialize them to html markup.
It should also help reduce coupling in your application. This is because the Components are more clearly separated from each other, there is less dependency between them.
Security is another benefit, user input is not parsed as HTML unless you explicitly want it to be.
This library has unit tests with 100% branch coverage. You can also run this test suite in the various browsers.
If you would like to use domv on your server using node.js or io.js you need to install domv for your project first:
npm install domv inherits --save
You will then need to obtain a DOM Document
, domv has a convenience function to create this for you using jsdom:
var domv = require('domv');
var document = domv.createHtmlDomDocument();
If you would like to use domv in a web browser, you can use one of two methods.
If you are already using browserify for your project, you can simply require domv:
var domv = require('domv');
Or you can generate an UMD bundle:
npm install domv
cd node_modules/domv
npm run bundle
This will generate a javascript file for you in build/domv.js
, here's an example on using it:
<!DOCTYPE html>
<html>
<head>
<script src="domv.js"></script>
<script>
(function(document, domv)
{
var image = domv.create(document, 'img', {src: 'foo.jpg'});
// ...
} (window.document, window.domv));
</script>
</head>
<body>
</body>
</html>
Now that you have obtained a DOM Document
and have access to the domv module, you can create new Components. When using the domv API you do not have to worry about which browser or server your code is running in.
The most important part of domv is the Component
class. This example shows how you can create one:
var domv = require('domv');
var image = domv.create(document, 'img', {src: 'foo.jpg', width: 200, height: 100});
console.log(image.stringifyAsHtml());
// <img src="foo.jpg" height="100" width="200">
Or you can use a convenient shorthand:
var domv = require('domv');
var div = domv.shorthand(document, 'div');
var img = domv.shorthand(document, 'img');
var component = div('columns',
div('left', 'This is the left column!'),
div('right',
'This is the right column...',
img({src: 'foo.jpg', width: 200, height: 100})
)
);
console.log(component.stringifyAsHtml());
// <div class="columns"><div class="left">This is the left column!</div>
// <div class="right">This is the right column...
// <img src="foo.jpg" height="100" width="200"></div></div>
Each Component
actually wraps a DOM Node
. This is useful to overcome differences between browsers and jsdom, however the real strength is that it lets you create subclasses of Component
(more on that later). Here is an example on wrapping:
var body = domv.wrap(document.body);
console.log(body !== document.body); // true
console.log(body.outerNode === document.body); // true
Note that domv.wrap
will always create a new Component
(this is by design):
var body = domv.wrap(document.body);
var body2 = domv.wrap(document.body);
console.log(body !== body2); // true
console.log(body.outerNode === body2.outerNode); // true
Subclassing Component
is what makes domv useful. A Component
wraps a DOM Node
and by doing so it gives you a stable API to modify the DOM with. When browsers introduce new features, your old Components will still use the same stable API without any clashes in attributes or method names. A lot of DOM methods are proxied with some extra flavoring (such asappendChild
, childNodes
, etc).
Here is an example:
// AuthorInfo.js
'use strict';
var domv = require('domv');
// the constructor for AuthorInfo
function AuthorInfo(node, author)
{
// call the constructor of our super class
domv.Component.call(this, node, 'div');
if (this.isCreationConstructor(node))
{
var img = this.shorthand('img');
var h2 = this.shorthand('h2');
var a = this.shorthand('a');
var p = this.shorthand('p');
var text = this.textShorthand();
this.cls('AuthorInfo');
this.attr('data-id', author.id);
this.appendChild(
this.avatar = img('avatar', { src: author.avatarUrl }),
this.name = h2('name',
this.nameLink = a(
{ href: '/author/' + encodeURIComponent(author.id) },
text(author.displayName)
)
),
this.introduction = p('introduction',
'about me: ',
text(author.introduction)
)
);
}
else // wrapping constructor
{
// assertHasClass and assertSelector are used to throw an
// Error in case invalid content is given to this constructor
this.assertHasClass('AuthorInfo');
this.avatar = this.assertSelector('> .avatar');
this.name = this.assertSelector('> .name');
this.nameLink = this.name.assertSelector('> a');
this.introduction = this.assertSelector('> .introduction');
}
// Add a DOM event listener
this.on('click', this._onclick);
}
module.exports = AuthorInfo;
// "inherits" module provides prototype chaining
require('inherits')(AuthorInfo, domv.Component);
Object.defineProperty(AuthorInfo.prototype, 'id', {
get: function()
{
return this.getAttr('data-id');
}
});
Object.defineProperty(AuthorInfo.prototype, 'displayName', {
get: function()
{
return this.nameLink.textContent;
},
set: function(val)
{
this.nameLink.textContent = val;
}
});
AuthorInfo.prototype._onclick = function(e)
{
this.toggleClass('highlighted');
};
(If you are using IntelliJ or WebStorm, there is a File Template you can import)
The constructor of a Component
subclass must always accept a node
argument as its first argument (a DOM Node
). If this argument is a Document
the Component must create new DOM elements and attributes.
As a rule of thumb, the outer element of a Component always has a class attribute that contains the name of the Component (beginning with a capital letter). Any other class name begins with a lowercase letter. This is import because it lets you easily debug where generated HTML came from, and it is also important when making sure your CSS rules only match your own Component.
var domv = require('domv');
var AuthorInfo = require('./AuthorInfo');
var body = domv.wrap(document.body);
var author = {
id: 500,
avatarUrl: 'someone.jpg',
displayName: 'Someone',
introduction: 'Hi! I am someone!!!'
};
var authorComponent = new AuthorInfo(document, author);
body.appendChild(authorComponent);
This results in the following HTML:
<div class="AuthorInfo" data-id="500">
<img src="someone.jpg" class="avatar">
<h2 class="name">
<a href="/author/500">Someone</a>
</h2>
<p class="introduction">about me: Hi! I am someone!!!</p>
</div>
If the node
argument is a different kind of Node
, usually an Element
, the Component must wrap existing content:
var domv = require('domv');
var AuthorInfo = require('./AuthorInfo');
var body = domv.wrap(document.body);
var element = body.selector('> .AuthorInfo');
var authorComponent = new AuthorInfo(element);
authorComponent.displayName = 'I just renamed myself!';
The wrapping constructor is especially useful if you would like to modify the DOM in the browser after having received HTML from the server.
Each Component
has an "outer" node and an "inner" node. You can access these nodes by using the outerNode
and innerNode
attributes. For most components innerNode
and outerNode
refer to the same Node
. But you can set them to something different anytime (usually in the constructor).
The outerNode
is used whenever you make a change to DOM attributes. Also, if a child Component is added to a parent Component, the outerNode
of the child is used.
The innerNode
is used to add any children and is also used for getters such as childNodes
, firstChild
, etc.
var domv = require('domv');
var document = domv.wrap(domv.createHtmlDomDocument());
var foo = document.create('div', 'outer');
var fooInner = document.create('div', 'inner');
foo.appendChild(fooInner);
foo.innerNode = fooInner;
foo.attr('data-example', 'This is set on the outer node!');
var bar = document.create('span', '', 'I am a span');
foo.appendChild(bar);
console.log(foo.stringifyAsHtml());
Result:
<div class="outer" data-example="This is set on the outer node!">
<div class="inner">
<span>I am a span</span>
</div>
</div>
HtmlDocument
is a Component
subclass which lets you create or wrap a html document. This includes the html, head, title and body elements, but it also provides a number of convenient methods to easily add style, script elements and JSON data. Unlike normal Components the node
argument in HtmlDocument is optional, if not given, a new Document
is constructed for you.
var domv = require('domv');
var author = {
id: 500,
avatarUrl: 'someone.jpg',
displayName: 'Someone',
introduction: 'Hi! I am someone!!!'
};
var html = new domv.HtmlDocument();
html.title = 'This is my page title';
html.baseURI = 'http://example.com/foo';
html.appendChild(html.create('p', 'myParagraph', 'This paragraph is added to the body'));
html.appendChild(new AuthorInfo(html.document, author));
html.addCSS('/my-bundle.css');
html.addJS('/my-bundle.js');
html.addJSONData('user-session', {userId: 1, name: 'root'});
console.log(html.stringifyAsHtml());
This gives you:
<!DOCTYPE html>
<html>
<head>
<base href="http://example.com/foo">
<title>This is my page title</title>
<link href="/my-bundle.css" rel="stylesheet" type="text/css">
<script async="async" src="/my-bundle.js" type="text/javascript"></script>
<script data-identifier="user-session" type="application/json">
{"userId":1,"name":"root"}
</script>
</head>
<body>
<p class="myParagraph">This paragraph is added to the body</p>
<div data-id="500" class="AuthorInfo">
<img src="someone.jpg" class="avatar">
<h2 class="name"><a href="/author/500">Someone</a></h2>
<p class="introduction">about me: Hi! I am someone!!!</p>
</div>
</body>
</html>
And this is an example with wrapping:
var domv = require('domv');
var html = new domv.HtmlDocument(document.documentElement);
html.title = 'A new title!';
var session = html.getJSONData('user-session');
console.log('My user id is', session.userId, 'And my name is', session.name);
var authorInfo = html.assertSelector('> .AuthorInfo', AuthorInfo);
console.log('display name of the author you are viewing:', authorInfo.displayName);
Because this is all just plain javascript, it is easy to publish your Component
as a node module on npm. This works especially well when you use browserify.
Most Components also need some CSS to function properly. For this purpose stylerefs is a good solution. It lets you list the CSS or LESS files your Component needs in the source code using a require call:
'use strict';
var domv = require('domv');
require('static-reference')('./AuthorInfo.less', 'optional', 'filter', 'keywords');
// the constructor for AuthorInfo
function AuthorInfo(node, author)
{
// call the constructor of our super class
domv.Component.call(this, node, 'div');
if (this.isCreationConstructor(node))
{
... SNIP ...
This works a lot like browserify. You simply point the stylerefs
command line tool to your code and it will recursively analyze all the require()
calls in your app in order to find the CSS / LESS files that you need. You can use the output of this tool to generate a single CSS bundle file. (instead of the command line tool you can also use grunt. Look at the stylerefs documentation for more details.
This has been generated by jsdoc, you can create your own fresh copy by executing npm run documentation
(which will also give you a html version).
Modules
Typedefs
#domv/lib/Component This is the super class for your components.
It contains a handful of methods that simplify using the DOM.
Author: Joris van der Wel joris@jorisvanderwel.com ##class: Component ⏏ Members
###new Component(node, [defaultNodeName], [wrapDocument]) Each Component has two constructors, one is used to wrap existing DOM nodes, the other is used to create new elements. Both constructors should result in the same DOM structure.
Which constructor is used depends on the type of the node argument, isCreationConstructor is used to test for this. A DOCUMENT_NODE will result in the creation constructor, any other node will result in the wrapping constructor.
Any subclass should accept a Node as their first argument in their constructors. This library does not care about any other argument in your constructors.
Params
Node
| domv/lib/Component
- Any kind of node or a component with an outerNode,this
parameter determines which constructor is used using isCreationConstructor.string
- If the creation constructor is used, this will be the tag that gets used to create
the default element. This is a convenience for subclasses.boolean
- Used by wrap
If false, passing a DOCUMENT_NODE as "node" will result in an empty tag being created instead of wrapping the DOCUMENT_NODE. This behaviour is more convenient when subclassing Component because it lets you treat subclasses and subsubclasses in the same way. (e.g. the subclass Menu adds the class 'Menu' and common menu items. The subsubclass EventMenu adds the class 'EventMenu' and further event menu items.)
If true, a document will always wrap.
Type: domv/lib/Exception
Example
new Component(document.createElement('p')); // wraps a "p" element
Example
new Component(document); // Creates an empty "div" element as a default
Example
new Component(document, true); // Wraps the Document Node instead of creating an empty "div"
###domv/lib/Component.document The Document Node that the nodes of this Component are associated with.
Type: Document ###domv/lib/Component.outerNode The "outer" DOM Node for this component.
This node is used to apply attributes or when adding this component as the child of another.
This property is usually set by the Component constructor
Type: Node ###domv/lib/Component.outerNodeWrapped The outer DOM node wrapped as a new component.
Type: domv/lib/Component ###domv/lib/Component.innerNode The "inner" DOM Node for this component.
This is used when adding nodes or other components as the child of this Component. E.g. when using methods such as appendChild() and prependChild() or properties such as childNodes or firstChild
This property is usually set by the Component constructor, or by your subclass constructor
If this property is set to null, children are not allowed for this component. Note that innerNode may also reference nodes that do not allow children because of their type (such as a TextNode)
Type: Node ###domv/lib/Component.innerNodeWrapped The inner DOM node wrapped as a new component.
Type: domv/lib/Component ###domv/lib/Component.style The inline style for the outer node.
Type: CSSStyleDeclaration ###domv/lib/Component.children The (wrapped) child elements of the inner node. The returned list is not live.
Type: Array.<domv/lib/Component> ###domv/lib/Component.childrenIndex The index of the outerNode in the "children" attribute of the parentNode.
Type: int
Example
myParent.children[3].childrenIndex === 3
###domv/lib/Component.childNodes The (wrapped) child nodes of the inner node. The returned list is not live.
Type: Array.<domv/lib/Component> ###domv/lib/Component.childNodesIndex The index of the outerNode in the "childNodes" attribute of the parentNode.
Type: int
Example
myParent.childNodes[3].childNodesIndex === 3
###domv/lib/Component.isEmpty Is the inner node empty? For Element nodes this means that there are 0 child nodes. For CharacterData nodes, the text content must be of 0 length. Other nodes are never considered empty
Type: boolean
###domv/lib/Component.firstChild
The first (wrapped) child node of the inner node.
Type: domv/lib/Component ###domv/lib/Component.lastChild The first (wrapped) child node of the inner node.
Type: domv/lib/Component ###domv/lib/Component.firstElementChild The first (wrapped) child node of the inner node that is an element.
Type: domv/lib/Component ###domv/lib/Component.lastElementChild The last (wrapped) child node of the inner node that is an element.
Type: domv/lib/Component ###domv/lib/Component.nextSibling The next (wrapped) sibling of the outer node.
Type: domv/lib/Component ###domv/lib/Component.previousSibling The previous (wrapped) sibling of the outer node.
Type: domv/lib/Component ###domv/lib/Component.nextElementSibling The next (wrapped) sibling of the outer node that is an element.
Type: domv/lib/Component ###domv/lib/Component.previousElementSibling The previous (wrapped) sibling of the outer node that is an element.
Type: domv/lib/Component ###domv/lib/Component.parentNode The (wrapped) parent node of the outer node.
Type: domv/lib/Component ###domv/lib/Component.textContent The textual content of an element and all its descendants. Or for Text, Comment, etc nodes it represents the nodeValue. Setting this property on an element removes all of its children and replaces them with a single text node with the given value.
Type: string
###domv/lib/Component.value
The value of this node. For most nodes this property is undefined, for input fields this
contains the current value. (The attribute "value" does not change by user input).
Type: string
###domv/lib/Component.checked
The checked state of this node. For most nodes this property is undefined, for input elements this
contains the checked state. (The attribute "checked" does not change by user input).
Type: boolean
###domv/lib/Component.selected
The selected state of this node. For most nodes this property is undefined, for option elements this
contains the current selected state. (The attribute "selected" does not change by user input).
Type: boolean
###domv/lib/Component.outerNodeType
The node type of the outer node.
Type: NodeType ###domv/lib/Component.innerNodeType The node type of the inner node.
Type: NodeType ###domv/lib/Component.outerNodeName The node name of the outer node. (element tag names always in lowercase)
Type: string
###domv/lib/Component.innerNodeName
The node name of the inner node.
Type: string
###domv/lib/Component.isCreationConstructor(node, [wrapDocument])
Params
Node
| domv/lib/Component
- A DOCUMENT_NODE will result in the
creation constructor, any other node will result in the wrapping constructor. A falsy value will also result in the
creation constructor and it used in Component subclasses that know how to create their own DOCUMENT_NODE
(e.g. domv/lib/HtmlDocument.boolean
- If false, passing a DOCUMENT_NODE as "node" will result in an empty tag being created instead of wrapping the DOCUMENT_NODE. This behaviour is more convenient when subclassing Component because it lets you treat subclasses and subsubclasses in the same way. (e.g. the subclass Menu adds the class 'Menu' and common menu items. The subsubclass EventMenu adds the class 'EventMenu' and further event menu items.)
If true, a document will always wrap.
Returns: boolean
- If thee creation constructor should be used instead of the wrapping constructor.
###domv/lib/Component.on(event, listener, [useCapture], [thisObject])
Adds a listener to the DOM.
Params
string
function
boolean
- Use the capture phase (for dom events)*
- The this object of "listener".
By default the "this" object of this method is usedType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.addListener(event, listener, [useCapture], [thisObject]) Adds a listener to the DOM or to the internal EventEmmiter, depending on the type of the event (see module:domv.isDOMEvent)
Params
string
function
boolean
- Use the capture phase (for dom events)*
- The this object of "listener".
By default the "this" object of this method is usedReturns: domv/lib/Component - this ###domv/lib/Component.removeListener(event, listener, [useCapture], [thisObject]) Removes a listener from the DOM. All of the parameters must equal the parameters that were used in addListener.
Params
string
function
boolean
*
Returns: domv/lib/Component - this ###domv/lib/Component.cleanup() Mark that this Component should perform any cleanup it wants to. Normally a Component do not need any cleanup, however this might be needed in special circumstances. This implementation clears all dom listeners set through this Component instance and it emits (local) the 'domv-cleanup' event.
###domv/lib/Component.clearListeners() Removes all (DOM) listeners from the outerNode.
###domv/lib/Component.emit(name, [data], [bubbles], [cancelable]) Emits a DOM custom Event on the outerNode with optional data. The listeners are passed an Event object as their first argument which has this data set
Params
String
- The event name, a prefix should be added to prevent name clashes with any new event names in
web browsers. (e.g. "domv-somethinghappened"Object
- Key value pairs to set on the Event objectboolean
boolean
Type: domv/lib/Exception
Returns: boolean
- False if any of the event listeners has called preventDefault(), otherwise true
###domv/lib/Component.isOuterNodeEqual(node)
Is the outer DOM node equal to the given node?.
If a Component is given the outer nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/Component.isInnerNodeEqual(node)
Is the inner DOM node equal to the given node?.
If a Component is given the inner nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/Component.isNodeEqual(node)
Are the outer and inner node equal to the given node?
If a Component is given the outer and inner nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/Component.create(nodeName, className, [...content])
Convenient function to create a wrapped Node including its attributes (for elements).
Params
string
string
string
| Node
| domv/lib/Component
| Object.<string, string>
- If a string is passed, a text node is appended.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes.
Type: domv/lib/Exception Returns: domv/lib/Component ###domv/lib/Component.text(...text_) Creates a new wrapped TextNode.
Params
string
- Extra arguments will be joined using a spaceReturns: domv/lib/Component
Example
var wrappedDiv = require('domv').create(document, 'div');
var wrappedText = require('domv').text(document, 'Hi!');
wrappedDiv.appendChild(wrappedText);
console.log(wrappedDiv.outerNode.outerHTML);
// <div>Hi!</div>
###domv/lib/Component.shorthand([tagName], ...initialAttributes) Generate a short hand function wich lets you quickly create new elements (wrapped) including attributes.
Params
string
string
| Object.<string, string>
- If a string is passed, a text node is appended.
If an object of key value pairs is passed, it sets those as attributes (see attr).
Type: domv/lib/Exception Returns: domv/lib/CreateShortHand
Example
var a = this.shorthand('a');
var link = a('readmore', {'href': something()}, 'Click here to readmore!');
// <a class="readmore" href="#example">Click here to readmore!</a>
###domv/lib/Component.textShorthand() Generate a short hand function which lets you quickly create new text nodes (wrapped).
Returns: function
Example
var text = this.textShorthand();
var wraped = text('bla');
wrapped = text('foo', 'bar'); // 'foo bar'
###domv/lib/Component.appendChild(...node_) Add a child node at the end of the inner node.
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.prependChild(...node_) Add a child node at the beginning of the inner node.
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.siblingBefore(...node_) Add a sibling node before the outer node. (which will become the outer node's previousSibling)
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.siblingAfter(...node_) Add a sibling node after the outer node. (which will become the outer node's nextSibling)
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.removeNode() Removes the outer node from its parentNode.
Returns: domv/lib/Component - this ###domv/lib/Component.removeChildren() Removes the all the children of the inner node
Returns: domv/lib/Component - this ###domv/lib/Component.cls(...cls) Add a className on the outer node.
Params
string
- The className to addType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.addClass(...cls) Add classNames on the outer node.
Params
string
- The classNames to addType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.removeClass(...cls) Remove classNames from the outer node.
Params
string
- The classNames to removeType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.hasClass(...cls) Does the outer node contain all of the given classNames?
Params
string
- The classNames to check.Returns: boolean
###domv/lib/Component.assertHasClass(...cls)
Does the outer node contain all of the given classNames?
Params
string
- The classNames to check.Type: domv/lib/Exception ###domv/lib/Component.toggleClass(cls, force) Toggle a className on the outer node.
Params
string
- The className to toggleboolean
- If set, force the class name to be added (true) or removed (false).Type: domv/lib/Exception
Returns: boolean
###domv/lib/Component.attr(name, value)
Set/unset an attribute on the outer node.
Params
string
| Object.<string, string>
- The attribute name to unset/set.
Or an object of key value pairs which sets multiple attributes at the same time,
in this case "value" should not be set.string
| boolean
- The value to set.
Use boolean false or null to unset the attribute. Use boolean true to set a boolean attribute (e.g. checked="checked").
If an object or array is passed, it is stringified using JSON.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/Component.getAttr(name, [json]) Get the value of a single attribute of the outer node.
Params
string
- The attribute name to get.boolean
- If true, the attribute value is parsed as jsonReturns: string
- The attribute value
###domv/lib/Component.selector(selector, [componentConstructor])
Returns the first element, or null, that matches the specified single selector.
(applied on the inner node)
Params
string
function
- The constructor to
use to wrap the result Node, by default the Node is wrapped in a plain Component,
but it is also possible to specify your own constructor.Returns: domv/lib/Component ###domv/lib/Component.assertSelector(selector, [componentConstructor]) Returns the first element that matches the specified single selector. (applied on the inner node)
Params
string
function
- The constructor to
use to wrap the result Node, by default the Node is wrapped in a plain Component,
but it is also possible to specify your own constructor.Type: domv/lib/Exception Returns: domv/lib/Component ###domv/lib/Component.selectorAll(selector, [componentConstructor]) Returns a list of all elements that matches the specified single selector. (applied on the inner node)
Params
string
function
- The constructor to
use to wrap the resulting Nodes, by default the Nodes are wrapped in a plain Component,
but it is also possible to specify your own constructor.Returns: Array.<domv/lib/Component> ###domv/lib/Component.adoptAllAttributes(from) Copy all attributes from the given element to our outer node.
Params
domv/lib/Component
| Element
- A DOM Element or if a Component is passed, the outerNode.Type: domv/lib/Exception ###domv/lib/Component.swapNode(node) Move over all child nodes of the inner node to the given "node" and replace the outer node with the given "node".
Params
Element
| domv/lib/Component
- The node to replace our outer node with.
If not set, the children of our inner node are added to the parent of the outer node.Type: domv/lib/Exception
Example
var container = document.createElement('div');
container.innerHTML = '<section>abc<p>def<strong>ghj</strong>klm</p>nop</section>';
domv.wrap(container).selector('p').swap(document.createElement('h1'));
console.log(container.innerHTML);
// '<section>abc<h1>def<strong>ghj</strong>klm</h1>nop</section>'
###domv/lib/Component.isAllWhiteSpace([checkChildElements]) Does the innerNode (and its (grand)children) of this component only consist of whitespace? Text nodes that only consist of spaces, newlines and horizontal tabs are whitespace. Comment nodes are whitespace. Empty text, comment, element nodes are whitespace. Certain content elements such as for example img, video, input, etc are not whitespace.
Params
boolean
- If false any element node (e.g. an empty div) that is
encountered will fail the whitespace check. If true those elements are checked recursively
for whitepspaceReturns: boolean
###domv/lib/Component.stringifyAsHtml()
Stringify the outerNode and all its children as html markup.
Returns: string
###domv/lib/Component.sendResponseAsHtml(response)
Stringify the outerNode and all its children as html markup, and send it
as a http response in node.js with the proper Content-Type and Content-Length.
Other headers can be set by calling setHeader() on the response before calling
this method. The status code can be set by setting response.statusCode in the same
fashion (the default is 200).
This method uses this.stringifyAsHtml() to generate the markup (which can be
overridden).
Params
ServerResponse
###domv/lib/Component.splice() This method does nothing, it is used so that firebug and chrome displays Component objects as an array. This method is not used by this library, feel free to override this method.
###domv/lib/Component.updateConsoleHack()
Called by whenever an inner/outer node changes. This enables pretty formatting of Component instances in the firebug and chrome console.
Firebug will display instances as:
"Object["BaseDocument", html.BaseDocument, div.content]"
Chrome will display instances as:
["BaseDocument", <html class="BaseDocument">...</html>, <div class="content">…</div>...</div>]
This hack works by setting the attributes "length", "0", "1" and "2" ("splice" is set on the prototype also). Override this method to do nothing in your subclass to disable this hack.
###const: domv/lib/Component.isDOMVComponent Always true for instances of this class.
Use this attribute to determine if an object is a Component. This would let you create an object compatible with this API, without having to use Component as a super type.
Type: boolean
#domv/lib/Exception
The base class for any exception that originates from this library
Author: Joris van der Wel joris@jorisvanderwel.com
##class: Exception ⏏
Extends: Error
Members
###new Exception(wrapped) Construct a simple domv.Exception
Params
Error
Extends: Error
Example
new domv.Exception(new Error('Hrm'));
###const: domv/lib/Exception.isDOMVException Always true for instances of this class.
Use this attribute to determine if an object is a Component. This would let you create an object compatible with this API, without having to use Component as a super type.
Type: boolean
#domv/lib/HtmlDocument
Represents a full document in html, including the root node html.
Author: Joris van der Wel joris@jorisvanderwel.com
##class: HtmlDocument ⏏
Extends: module:domv/lib/Component
Members
###new HtmlDocument(node) This constructor can be used to either create a new html document (html, head, body), or to wrap an existing html document into this class.
Params
Node
| domv/lib/Component
- Either null, a document node or an "html" element nodeExtends: module:domv/lib/Component
Type: domv/lib/Exception
Example
new HtmlDocument() // create a new Document Node, including html (as its child), head, body.
Example
new HtmlDocument(document); // Create html, head and body elements using the given Document Node,
but do not modify the given Document node (constructors should be side-effect free).
Example
new HtmlDocument(document.documentElement); // Associate an existing html document
###domv/lib/HtmlDocument.head The "head" Element of the document.
Type: domv/lib/Component ###domv/lib/HtmlDocument.body The "body" Element of the document.
Type: domv/lib/Component ###domv/lib/HtmlDocument.baseWrapped The "base" Element of the document. (within the "head" node)
Type: domv/lib/Component ###domv/lib/HtmlDocument.titleWrapped The "title" Element of the document. (within the head node)
Type: domv/lib/Component ###domv/lib/HtmlDocument.title The textContent of the "title" Element of this document. (within the "head" node)
Type: string
###domv/lib/HtmlDocument.baseURI
The base URI that is used to resolve all the relative uri's within this document.
(this is get/set using a "base" Element within the "head" element)
Type: string
###domv/lib/HtmlDocument.document
The Document Node that the nodes of this Component are associated with.
Type: Document ###domv/lib/HtmlDocument.outerNode The "outer" DOM Node for this component.
This node is used to apply attributes or when adding this component as the child of another.
This property is usually set by the Component constructor
Type: Node ###domv/lib/HtmlDocument.outerNodeWrapped The outer DOM node wrapped as a new component.
Type: domv/lib/Component ###domv/lib/HtmlDocument.innerNode The "inner" DOM Node for this component.
This is used when adding nodes or other components as the child of this Component. E.g. when using methods such as appendChild() and prependChild() or properties such as childNodes or firstChild
This property is usually set by the Component constructor, or by your subclass constructor
If this property is set to null, children are not allowed for this component. Note that innerNode may also reference nodes that do not allow children because of their type (such as a TextNode)
Type: Node ###domv/lib/HtmlDocument.innerNodeWrapped The inner DOM node wrapped as a new component.
Type: domv/lib/Component ###domv/lib/HtmlDocument.style The inline style for the outer node.
Type: CSSStyleDeclaration ###domv/lib/HtmlDocument.children The (wrapped) child elements of the inner node. The returned list is not live.
Type: Array.<domv/lib/Component> ###domv/lib/HtmlDocument.childrenIndex The index of the outerNode in the "children" attribute of the parentNode.
Type: int
Example
myParent.children[3].childrenIndex === 3
###domv/lib/HtmlDocument.childNodes The (wrapped) child nodes of the inner node. The returned list is not live.
Type: Array.<domv/lib/Component> ###domv/lib/HtmlDocument.childNodesIndex The index of the outerNode in the "childNodes" attribute of the parentNode.
Type: int
Example
myParent.childNodes[3].childNodesIndex === 3
###domv/lib/HtmlDocument.isEmpty Is the inner node empty? For Element nodes this means that there are 0 child nodes. For CharacterData nodes, the text content must be of 0 length. Other nodes are never considered empty
Type: boolean
###domv/lib/HtmlDocument.firstChild
The first (wrapped) child node of the inner node.
Type: domv/lib/Component ###domv/lib/HtmlDocument.lastChild The first (wrapped) child node of the inner node.
Type: domv/lib/Component ###domv/lib/HtmlDocument.firstElementChild The first (wrapped) child node of the inner node that is an element.
Type: domv/lib/Component ###domv/lib/HtmlDocument.lastElementChild The last (wrapped) child node of the inner node that is an element.
Type: domv/lib/Component ###domv/lib/HtmlDocument.nextSibling The next (wrapped) sibling of the outer node.
Type: domv/lib/Component ###domv/lib/HtmlDocument.previousSibling The previous (wrapped) sibling of the outer node.
Type: domv/lib/Component ###domv/lib/HtmlDocument.nextElementSibling The next (wrapped) sibling of the outer node that is an element.
Type: domv/lib/Component ###domv/lib/HtmlDocument.previousElementSibling The previous (wrapped) sibling of the outer node that is an element.
Type: domv/lib/Component ###domv/lib/HtmlDocument.parentNode The (wrapped) parent node of the outer node.
Type: domv/lib/Component ###domv/lib/HtmlDocument.textContent The textual content of an element and all its descendants. Or for Text, Comment, etc nodes it represents the nodeValue. Setting this property on an element removes all of its children and replaces them with a single text node with the given value.
Type: string
###domv/lib/HtmlDocument.value
The value of this node. For most nodes this property is undefined, for input fields this
contains the current value. (The attribute "value" does not change by user input).
Type: string
###domv/lib/HtmlDocument.checked
The checked state of this node. For most nodes this property is undefined, for input elements this
contains the checked state. (The attribute "checked" does not change by user input).
Type: boolean
###domv/lib/HtmlDocument.selected
The selected state of this node. For most nodes this property is undefined, for option elements this
contains the current selected state. (The attribute "selected" does not change by user input).
Type: boolean
###domv/lib/HtmlDocument.outerNodeType
The node type of the outer node.
Type: NodeType ###domv/lib/HtmlDocument.innerNodeType The node type of the inner node.
Type: NodeType ###domv/lib/HtmlDocument.outerNodeName The node name of the outer node. (element tag names always in lowercase)
Type: string
###domv/lib/HtmlDocument.innerNodeName
The node name of the inner node.
Type: string
###domv/lib/HtmlDocument.addCSS(href, media)
Link a css file to this document.
Params
string
- An absolute or relative URLstring
- The media query to associate with this css fileReturns: domv/lib/Component - The newly created "link" node ###domv/lib/HtmlDocument.addJS(href, [async]) Link a javascript file to this document.
Params
string
- An absolute or relative URLboolean
- If true, a webbrowser will continue parsing
the document even if the script file has not been fully loaded yet.
Use this whenever possible to decrease load times.Returns: domv/lib/Component - The newly created "script" node ###domv/lib/HtmlDocument.addJSONData(identifier, data) Expose JSON data to an interpreter of the HTML document using a script type="application/json" element. The data can be retrieved using getJSONData with the same identifier;
Params
string
- Must be unique to properly get your JSON data back*
- Any array, object, boolean, integer, et cetera that is able to be serialized into JSON.Returns: domv/lib/Component - The newly created "script" node
Example
myDoc.addJSONData('foo', {'abc': 'def'});
// <script type="application/json" data-identifier="foo">{"abc":"def"};</script>
###domv/lib/HtmlDocument.getJSONData(identifier) Retrieve JSON data previously exposed by addJSONData
Params
string
- Same identifier as was used in addJSONDataReturns: *
- parsed json data
###domv/lib/HtmlDocument.isCreationConstructor(node, [wrapDocument])
Params
Node
| domv/lib/Component
- A DOCUMENT_NODE will result in the
creation constructor, any other node will result in the wrapping constructor. A falsy value will also result in the
creation constructor and it used in Component subclasses that know how to create their own DOCUMENT_NODE
(e.g. domv/lib/HtmlDocument.boolean
- If false, passing a DOCUMENT_NODE as "node" will result in an empty tag being created instead of wrapping the DOCUMENT_NODE. This behaviour is more convenient when subclassing Component because it lets you treat subclasses and subsubclasses in the same way. (e.g. the subclass Menu adds the class 'Menu' and common menu items. The subsubclass EventMenu adds the class 'EventMenu' and further event menu items.)
If true, a document will always wrap.
Returns: boolean
- If thee creation constructor should be used instead of the wrapping constructor.
###domv/lib/HtmlDocument.on(event, listener, [useCapture], [thisObject])
Adds a listener to the DOM.
Params
string
function
boolean
- Use the capture phase (for dom events)*
- The this object of "listener".
By default the "this" object of this method is usedType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.addListener(event, listener, [useCapture], [thisObject]) Adds a listener to the DOM or to the internal EventEmmiter, depending on the type of the event (see module:domv.isDOMEvent)
Params
string
function
boolean
- Use the capture phase (for dom events)*
- The this object of "listener".
By default the "this" object of this method is usedReturns: domv/lib/Component - this ###domv/lib/HtmlDocument.removeListener(event, listener, [useCapture], [thisObject]) Removes a listener from the DOM. All of the parameters must equal the parameters that were used in addListener.
Params
string
function
boolean
*
Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.cleanup() Mark that this Component should perform any cleanup it wants to. Normally a Component do not need any cleanup, however this might be needed in special circumstances. This implementation clears all dom listeners set through this Component instance and it emits (local) the 'domv-cleanup' event.
###domv/lib/HtmlDocument.clearListeners() Removes all (DOM) listeners from the outerNode.
###domv/lib/HtmlDocument.emit(name, [data], [bubbles], [cancelable]) Emits a DOM custom Event on the outerNode with optional data. The listeners are passed an Event object as their first argument which has this data set
Params
String
- The event name, a prefix should be added to prevent name clashes with any new event names in
web browsers. (e.g. "domv-somethinghappened"Object
- Key value pairs to set on the Event objectboolean
boolean
Type: domv/lib/Exception
Returns: boolean
- False if any of the event listeners has called preventDefault(), otherwise true
###domv/lib/HtmlDocument.isOuterNodeEqual(node)
Is the outer DOM node equal to the given node?.
If a Component is given the outer nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/HtmlDocument.isInnerNodeEqual(node)
Is the inner DOM node equal to the given node?.
If a Component is given the inner nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/HtmlDocument.isNodeEqual(node)
Are the outer and inner node equal to the given node?
If a Component is given the outer and inner nodes of both components must match.
Params
Node
| domv/lib/Component
Returns: boolean
###domv/lib/HtmlDocument.create(nodeName, className, [...content])
Convenient function to create a wrapped Node including its attributes (for elements).
Params
string
string
string
| Node
| domv/lib/Component
| Object.<string, string>
- If a string is passed, a text node is appended.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes.
Type: domv/lib/Exception Returns: domv/lib/Component ###domv/lib/HtmlDocument.text(...text_) Creates a new wrapped TextNode.
Params
string
- Extra arguments will be joined using a spaceReturns: domv/lib/Component
Example
var wrappedDiv = require('domv').create(document, 'div');
var wrappedText = require('domv').text(document, 'Hi!');
wrappedDiv.appendChild(wrappedText);
console.log(wrappedDiv.outerNode.outerHTML);
// <div>Hi!</div>
###domv/lib/HtmlDocument.shorthand([tagName], ...initialAttributes) Generate a short hand function wich lets you quickly create new elements (wrapped) including attributes.
Params
string
string
| Object.<string, string>
- If a string is passed, a text node is appended.
If an object of key value pairs is passed, it sets those as attributes (see attr).
Type: domv/lib/Exception Returns: domv/lib/CreateShortHand
Example
var a = this.shorthand('a');
var link = a('readmore', {'href': something()}, 'Click here to readmore!');
// <a class="readmore" href="#example">Click here to readmore!</a>
###domv/lib/HtmlDocument.textShorthand() Generate a short hand function which lets you quickly create new text nodes (wrapped).
Returns: function
Example
var text = this.textShorthand();
var wraped = text('bla');
wrapped = text('foo', 'bar'); // 'foo bar'
###domv/lib/HtmlDocument.appendChild(...node_) Add a child node at the end of the inner node.
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.prependChild(...node_) Add a child node at the beginning of the inner node.
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.siblingBefore(...node_) Add a sibling node before the outer node. (which will become the outer node's previousSibling)
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.siblingAfter(...node_) Add a sibling node after the outer node. (which will become the outer node's nextSibling)
Params
domv/lib/Component
| Node
- A plain Node or if a Component is passed, the outerNode.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.removeNode() Removes the outer node from its parentNode.
Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.removeChildren() Removes the all the children of the inner node
Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.cls(...cls) Add a className on the outer node.
Params
string
- The className to addType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.addClass(...cls) Add classNames on the outer node.
Params
string
- The classNames to addType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.removeClass(...cls) Remove classNames from the outer node.
Params
string
- The classNames to removeType: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.hasClass(...cls) Does the outer node contain all of the given classNames?
Params
string
- The classNames to check.Returns: boolean
###domv/lib/HtmlDocument.assertHasClass(...cls)
Does the outer node contain all of the given classNames?
Params
string
- The classNames to check.Type: domv/lib/Exception ###domv/lib/HtmlDocument.toggleClass(cls, force) Toggle a className on the outer node.
Params
string
- The className to toggleboolean
- If set, force the class name to be added (true) or removed (false).Type: domv/lib/Exception
Returns: boolean
###domv/lib/HtmlDocument.attr(name, value)
Set/unset an attribute on the outer node.
Params
string
| Object.<string, string>
- The attribute name to unset/set.
Or an object of key value pairs which sets multiple attributes at the same time,
in this case "value" should not be set.string
| boolean
- The value to set.
Use boolean false or null to unset the attribute. Use boolean true to set a boolean attribute (e.g. checked="checked").
If an object or array is passed, it is stringified using JSON.Type: domv/lib/Exception Returns: domv/lib/Component - this ###domv/lib/HtmlDocument.getAttr(name, [json]) Get the value of a single attribute of the outer node.
Params
string
- The attribute name to get.boolean
- If true, the attribute value is parsed as jsonReturns: string
- The attribute value
###domv/lib/HtmlDocument.selector(selector, [componentConstructor])
Returns the first element, or null, that matches the specified single selector.
(applied on the inner node)
Params
string
function
- The constructor to
use to wrap the result Node, by default the Node is wrapped in a plain Component,
but it is also possible to specify your own constructor.Returns: domv/lib/Component ###domv/lib/HtmlDocument.assertSelector(selector, [componentConstructor]) Returns the first element that matches the specified single selector. (applied on the inner node)
Params
string
function
- The constructor to
use to wrap the result Node, by default the Node is wrapped in a plain Component,
but it is also possible to specify your own constructor.Type: domv/lib/Exception Returns: domv/lib/Component ###domv/lib/HtmlDocument.selectorAll(selector, [componentConstructor]) Returns a list of all elements that matches the specified single selector. (applied on the inner node)
Params
string
function
- The constructor to
use to wrap the resulting Nodes, by default the Nodes are wrapped in a plain Component,
but it is also possible to specify your own constructor.Returns: Array.<domv/lib/Component> ###domv/lib/HtmlDocument.adoptAllAttributes(from) Copy all attributes from the given element to our outer node.
Params
domv/lib/Component
| Element
- A DOM Element or if a Component is passed, the outerNode.Type: domv/lib/Exception ###domv/lib/HtmlDocument.swapNode(node) Move over all child nodes of the inner node to the given "node" and replace the outer node with the given "node".
Params
Element
| domv/lib/Component
- The node to replace our outer node with.
If not set, the children of our inner node are added to the parent of the outer node.Type: domv/lib/Exception
Example
var container = document.createElement('div');
container.innerHTML = '<section>abc<p>def<strong>ghj</strong>klm</p>nop</section>';
domv.wrap(container).selector('p').swap(document.createElement('h1'));
console.log(container.innerHTML);
// '<section>abc<h1>def<strong>ghj</strong>klm</h1>nop</section>'
###domv/lib/HtmlDocument.isAllWhiteSpace([checkChildElements]) Does the innerNode (and its (grand)children) of this component only consist of whitespace? Text nodes that only consist of spaces, newlines and horizontal tabs are whitespace. Comment nodes are whitespace. Empty text, comment, element nodes are whitespace. Certain content elements such as for example img, video, input, etc are not whitespace.
Params
boolean
- If false any element node (e.g. an empty div) that is
encountered will fail the whitespace check. If true those elements are checked recursively
for whitepspaceReturns: boolean
###domv/lib/HtmlDocument.stringifyAsHtml()
Stringify the outerNode and all its children as html markup.
Returns: string
###domv/lib/HtmlDocument.sendResponseAsHtml(response)
Stringify the outerNode and all its children as html markup, and send it
as a http response in node.js with the proper Content-Type and Content-Length.
Other headers can be set by calling setHeader() on the response before calling
this method. The status code can be set by setting response.statusCode in the same
fashion (the default is 200).
This method uses this.stringifyAsHtml() to generate the markup (which can be
overridden).
Params
ServerResponse
###domv/lib/HtmlDocument.splice() This method does nothing, it is used so that firebug and chrome displays Component objects as an array. This method is not used by this library, feel free to override this method.
###domv/lib/HtmlDocument.updateConsoleHack()
Called by whenever an inner/outer node changes. This enables pretty formatting of Component instances in the firebug and chrome console.
Firebug will display instances as:
"Object["BaseDocument", html.BaseDocument, div.content]"
Chrome will display instances as:
["BaseDocument", <html class="BaseDocument">...</html>, <div class="content">…</div>...</div>]
This hack works by setting the attributes "length", "0", "1" and "2" ("splice" is set on the prototype also). Override this method to do nothing in your subclass to disable this hack.
###const: domv/lib/HtmlDocument.isDOMVComponent Always true for instances of this class.
Use this attribute to determine if an object is a Component. This would let you create an object compatible with this API, without having to use Component as a super type.
Type: boolean
#domv
Author: Joris van der Wel joris@jorisvanderwel.com
Members
##enum: domv.NodeType All of the valid node types in DOM (excluding the ones that are deprecated).
Type: number
Properties: ELEMENT
, TEXT
, PROCESSING_INSTRUCTION
, COMMENT
, DOCUMENT
, DOCUMENT_TYPE
, DOCUMENT_FRAGMENT
##domv.isSupported(document, [checkAll])
Test if the current environment / browser / DOM library supports everything that is needed for the domv library.
Params
Document
boolean
- If true, also check isParseHTMLDocumentSupportedType: domv/lib/Exception
Returns: boolean
##domv.isParseHTMLDocumentSupported()
Test if the current environment / browser / DOM library supports parsing the markup of an
entire html document (including doctype, html, head, tags etc).
Returns: boolean
##domv.mayContainChildren(node, [doThrow])
Is the given node or component able to have children?.
Params
domv/lib/Component
| Node
boolean
- If true, throw instead of returning false upon failure.Type: domv/lib/Exception
Returns: boolean
##domv.wrap(node_, [ComponentConstructor], ...constructorArguments)
Wraps a plain DOM Node so that you can use the same API as you would on a Component.
If you pass a NodeList, an array (that is not live) with Component's will be returned.
Passing a falsy value will also return a falsy value instead of a Component
Params
Node
| Array.<Node>
function
- The constructor to
use to wrap the given Node, by default the Node is wrapped in a plain Component,
but it is also possible to specify your own constructor. The first argument is always
the node being wrapped.*
- Further arguments to be passed to the constructorType: domv/lib/Exception Returns: domv/lib/Component | Array.<domv/lib/Component> - Only null if "node" was also null
Example
domv.wrap(document.body).prependChild(...);
Example
domv.wrap(someNode, MyPictureGallery).addPicture(...);
##domv.unlive(nodeList) Returns an array copy of a NodeList so that it is no longer live. This makes it easier to properly modify the DOM while traversing a node list. The actual content of the array is identical (the nodes are not wrapped)
Params
*
- Any array like object.Returns: Array
Example
var list = require('domv').unlive(document.getElementsByTagName('*'));
##domv.create(document_, nodeName, className, ...content) Convenient function to create a wrapped Node including its attributes (for elements).
Params
Document
string
string
| Node
| domv/lib/Component
| Object.<string, string>
- If a string is passed, and the nodeName represents an element tag, the string is set as the class attribute. If not an element, the string is appended to the node data.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes (see [attr](#module_domv/lib/Component#attr)).
string
| Node
| domv/lib/Component
| Object.<string, string>
- If a string is passed, and the nodeName represents an element tag, the string is appended as a text node. If not an element, the string is appended to the node data.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes (see [attr](#module_domv/lib/Component#attr)).
Type: domv/lib/Exception Returns: domv/lib/Component
Example
var wrappedDiv = require('domv').create(document, 'div', 'myDiv', 'This is my div!', {'data-test': 'foo'});
console.log(wrappedDiv.outerNode.outerHTML);
// <div class="myDiv" data-test="foo">This is my div!</div>
##domv.shorthand(document_, [tagName_], ...initialAttributes) Generate a short hand function wich lets you quickly create a wrapped Element including its attributes.
Params
Document
string
string
| Object.<string, string>
- If a string is passed, a text node is appended.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes. (see attr)
Returns: domv/lib/CreateShortHand
Example
var a = require('domv').shorthand(document, 'a');
var link = a('readmore', {'href': something()}, 'Click here to readmore!');
// <a class="readmore" href="#example">Click here to readmore!</a>
##domv.text(document_, ...text) Creates a new wrapped TextNode.
Params
Document
string
- Extra arguments will be joined using a spaceReturns: domv/lib/Component
Example
var wrappedDiv = require('domv').create(document, 'div');
var wrappedText = require('domv').text(document, 'Hi!');
wrappedDiv.appendChild(wrappedText);
console.log(wrappedDiv.outerNode.outerHTML);
// <div>Hi!</div>
##domv.createHtmlDomDocument([minimal]) Create a new Document Node, including html, head, title and body tags.
Params
Boolean
- If true, only a doctype and a element is created.Returns: Document ##domv.parseHTMLDocument(markup, ownerDocument) Parse the given html markup text as a complete html docuemnt and return the outer "html" node. Optionally an ownerDocument may be given which will specify what Document the new nodes belong to.
Params
string
Document
Type: domv/lib/Exception Returns: domv/lib/HtmlDocument ##domv.parseHTMLSnippit(ownerDocument, markup) Parse the given html markup text and return a (wrapped) DocumentFragment containing the nodes the markup represents. The given markup must not be a full html document, otherwise the html, head and body nodes will not be present, only their content. An ownerDocument must be given which will specify what Document the new nodes belong to.
Params
Document
string
Type: domv/lib/Exception Returns: domv/lib/Component ##domv.cssStringEscape([str], [wrapInQuotes]) Escape a string so that you can use it as a CSS String, such as a selector.
Params
String
boolean
- If true, surround the result with quotes: "something"Returns: String
Example
myComponent.selectorAll('a[href=' + domv.cssStringEscape(somevar) + ']');
##domv.isLeftMouseButton(event) Given that 'event' is a mouse event, is the left mouse button being held down?.
Params
Event
Type: domv/lib/Exception
Returns: boolean
- True if the left mouse button is down
##const: domv.Component
Type: domv/lib/Component
##const: domv.HtmlDocument
Type: domv/lib/HtmlDocument
##const: domv.Exception
Type: domv/lib/Exception
#callback: domv/lib/CreateShortHand
Params
string
string
| Node
| domv/lib/Component
| Object.<string, string>
- If a string is passed, a text node is appended.
If a node or component is passed, it is simply appended.
If an object of key value pairs is passed, it sets those as attributes. (see attr)
Type: function
FAQs
Create views as components using DOM. Run the same code on the browser and on the server.
The npm package domv receives a total of 8 weekly downloads. As such, domv popularity was classified as not popular.
We found that domv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.