dot-path-value
Safely get and set deep nested properties using dot notation.
Features
- TypeScript first 🤙
- Support arrays
- Tiny
- No dependencies
- Utility types
Path
and PathValue
If you find this library useful, why not
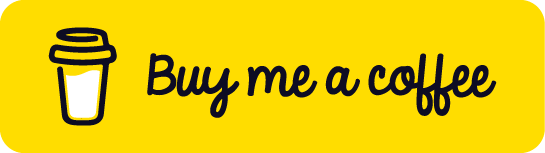
Installation
npm install dot-path-value
pnpm install dot-path-value
yarn add dot-path-value
Usage
import { getByPath, setByPath } from 'dot-path-value';
const obj = {
a: {
b: 'hello',
d: [
{
e: 'world',
}
],
},
};
getByPath(obj, 'a.b');
getByPath(obj, 'a.d.0.e');
getByPath(obj, 'a.d.0');
getByPath([{ a: 1 }], '0.a');
getByPath(obj, 'a.b.c');
setByPath(obj, 'a.b', 'hello there');
Types
dot-path-value
exports a few types to ensure the type safety:
Type | Description |
---|
Path<T> | converts nested structure T into a string representation of the paths to its properties |
PathValue<T, TPath> | returns the type of the value at the specified path |
Types usage
import { Path, PathValue } from 'dot-path-value';
const obj = {
a: {
b: 'hello',
d: [
{
e: 'world',
}
],
},
};
type Foo = Path<typeof obj>;
type Bar = PathValue<typeof obj, 'a.b'>;