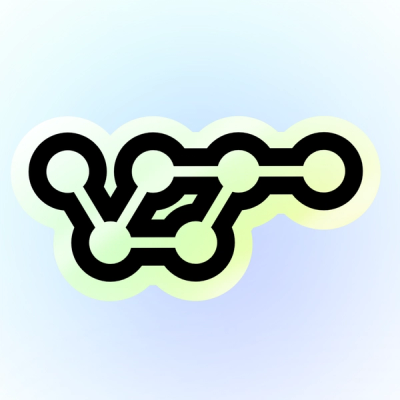
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
The dot-prop npm package allows for getting, setting, and deleting properties from objects using dot-path notation. This can be particularly useful for accessing deeply nested properties without having to check each level of the object structure.
Get a property
Retrieve a property from an object by specifying its path as a string.
const dotProp = require('dot-prop');
const object = {foo: {bar: 'a'}};
console.log(dotProp.get(object, 'foo.bar')); //=> 'a'
Set a property
Set a property on an object at a specified path, creating any necessary objects along the path.
const dotProp = require('dot-prop');
let object = {foo: {bar: 'a'}};
dotProp.set(object, 'foo.bar', 'b');
console.log(object.foo.bar); //=> 'b'
Delete a property
Delete a property from an object at a specified path.
const dotProp = require('dot-prop');
let object = {foo: {bar: 'a'}};
dotProp.delete(object, 'foo.bar');
console.log(object); //=> { foo: {} }
Check if an object has a property
Check if an object has a property at a specified path.
const dotProp = require('dot-prop');
const object = {foo: {bar: 'a'}};
console.log(dotProp.has(object, 'foo.bar')); //=> true
Similar to the 'get' functionality of dot-prop, lodash.get allows accessing a property value of an object using a dot-path. While lodash offers a broader utility toolkit, dot-prop focuses specifically on dot-path property manipulation.
object-path provides similar functionality to dot-prop for getting, setting, and deleting properties using a dot-path notation. It offers a similar API but might have different performance characteristics or additional minor features.
Get, set, or delete a property from a nested object using a dot path
$ npm install --save dot-prop
const dotProp = require('dot-prop');
// getter
dotProp.get({foo: {bar: 'unicorn'}}, 'foo.bar');
//=> 'unicorn'
dotProp.get({foo: {bar: 'a'}}, 'foo.notDefined.deep');
//=> undefined
dotProp.get({foo: {bar: 'a'}}, 'foo.notDefined.deep', 'default value');
//=> 'default value'
dotProp.get({foo: {'dot.dot': 'unicorn'}}, 'foo.dot\\.dot');
//=> 'unicorn'
// setter
const obj = {foo: {bar: 'a'}};
dotProp.set(obj, 'foo.bar', 'b');
console.log(obj);
//=> {foo: {bar: 'b'}}
const foo = dotProp.set({}, 'foo.bar', 'c');
console.log(foo);
//=> {foo: {bar: 'c'}}
dotProp.set(obj, 'foo.baz', 'x');
console.log(obj);
//=> {foo: {bar: 'b', baz: 'x'}}
// has
dotProp.has({foo: {bar: 'unicorn'}}, 'foo.bar');
//=> true
// deleter
const obj = {foo: {bar: 'a'}};
dotProp.delete(obj, 'foo.bar');
console.log(obj);
//=> {foo: {}}
obj.foo.bar = {x: 'y', y: 'x'};
dotProp.delete(obj, 'foo.bar.x');
console.log(obj);
//=> {foo: {bar: {y: 'x'}}}
Returns the object.
Type: Object
Object to get, set, or delete the path
value.
Type: string
Path of the property in the object, using .
to separate each nested key.
Use \\.
if you have a .
in the key.
Type: any
Value to set at path
.
Type: any
Default value.
MIT © Sindre Sorhus
FAQs
Get, set, or delete a property from a nested object using a dot path
We found that dot-prop demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.