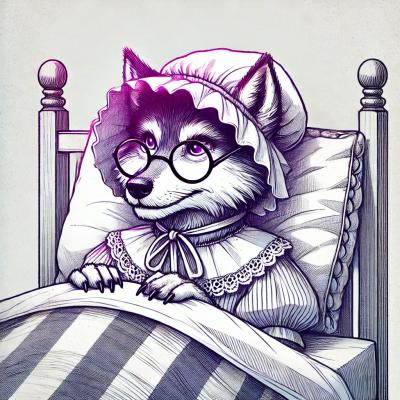
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The 'download' npm package is a utility that allows you to download files over HTTP or HTTPS. It is a high-level function that abstracts away the complexity of making HTTP requests and handling streams, making it easier to download files.
Downloading files
This feature allows you to download files from a given URL and save them to a specified directory. The function returns a promise that resolves when the download is complete.
const download = require('download');
download('https://example.com/somefile.png', 'dist').then(() => {
console.log('File downloaded!');
});
Downloading and extracting archives
This feature enables the downloading of archive files like ZIP or TAR and automatically extracts them to a specified directory.
const download = require('download');
download('https://example.com/somearchive.zip', 'dist', { extract: true }).then(() => {
console.log('Archive downloaded and extracted!');
});
Downloading files with options
This feature allows you to pass custom options such as headers, query parameters, and more, providing additional control over the HTTP request.
const download = require('download');
const options = {
headers: { 'User-Agent': 'my-custom-agent' }
};
download('https://example.com/somefile.png', 'dist', options).then(() => {
console.log('File downloaded with custom headers!');
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides more general HTTP request capabilities compared to 'download' and is often used for API interactions rather than file downloads.
Got is a human-friendly and powerful HTTP request library for Node.js. Similar to 'download', it supports streaming but offers a more extensive set of HTTP capabilities, making it suitable for a wider range of HTTP requests.
Request is a simplified HTTP request client for Node.js. Although it has been deprecated, it was once a popular choice for making HTTP requests and supports file downloads, but with less abstraction compared to 'download'.
Node-fetch is a light-weight module that brings the Fetch API to Node.js. It is similar to 'download' in that it can be used to download files, but it is designed to closely mimic the browser fetch API.
Download and extract files
See download-cli for the command-line version.
$ npm install --save download
const fs = require('fs');
const download = require('download');
download('http://unicorn.com/foo.jpg', 'dist').then(() => {
console.log('done!');
});
download('http://unicorn.com/foo.jpg').then(data => {
fs.writeFileSync('dist/foo.jpg', data);
});
download('unicorn.com/foo.jpg').pipe(fs.createWriteStream('dist/foo.jpg'));
Promise.all(['unicorn.com/foo.jpg', 'cats.com/dancing.gif'].map(x => download(x, 'dist'))).then(() => {
console.log('files downloaded!');
});
Returns both a Promise for a Buffer and a Duplex stream with additional events.
Type: string
URL to download.
Type: string
Path to where your file will be written.
Same options as got in addition to the ones below.
Type: boolean
Default: false
If set to true
, try extracting the file using decompress.
MIT © Kevin Mårtensson
FAQs
Download and extract files
The npm package download receives a total of 1,284,207 weekly downloads. As such, download popularity was classified as popular.
We found that download demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.