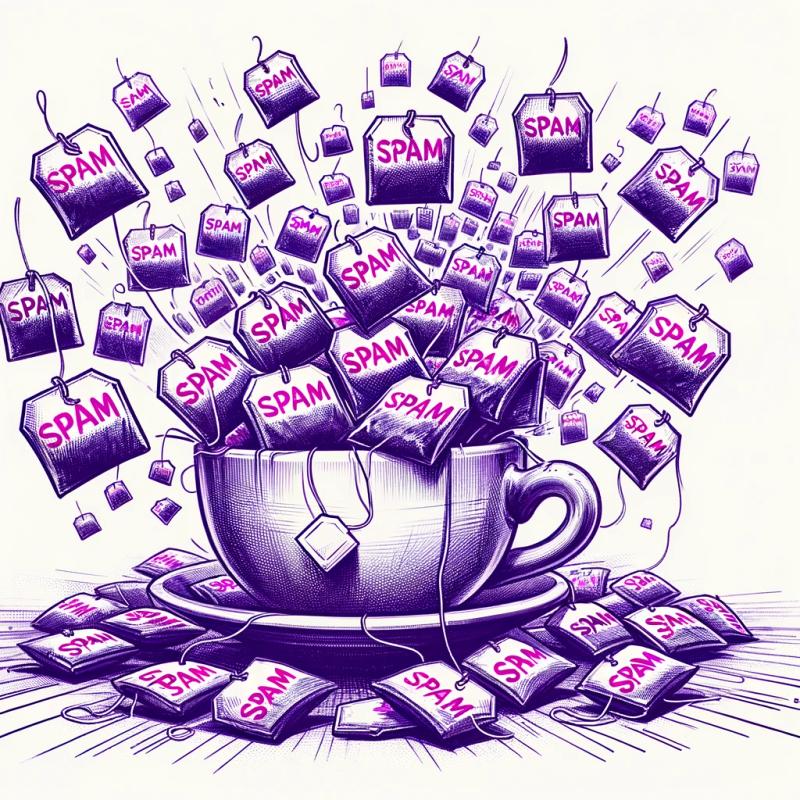
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
dpdm
Advanced tools
Readme
A robust static dependency analyzer for your JavaScript
and TypeScript
projects.
Highlights | Install | Usage | Options | API
CommonJS
, ESM
.JavaScript
and TypeScript
completely.
madge
, whose results are completely inconclusive when analyze TypeScript
.For command line
npm i -g dpdm
# or via yarn
yarn global add dpdm
As a module
npm i -D dpdm
# or via yarn
yarn add -D dpdm
Simple usage
dpdm ./src/index.ts
Print circular dependencies only
dpdm --no-warning --no-tree ./src/index.ts
Exit with a non-zero code if a circular dependency is found.
dpdm --exit-code circular:1 ./src/index.ts
Ignore type dependencies for TypeScript modules
dpdm -T ./src/index.ts
Find unused files by index.js
in src
directory:
dpdm --no-tree --no-warning --no-circular --detect-unused-files-from 'src/**/*.*' 'index.js'
Skip dynamic imports:
# The value circular will only ignore the dynamic imports
# when parse circular references.
# You can set it as tree to ignore the dynamic imports
# when parse source files.
dpdm --skip-dynamic-imports circular index.js
dpdm [options] <files...>
Analyze the files' dependencies.
Positionals:
files The file paths or globs [string]
Options:
--version Show version number [boolean]
--context the context directory to shorten path, default is current
directory [string]
--extensions, --ext comma separated extensions to resolve
[string] [default: ".ts,.tsx,.mjs,.js,.jsx,.json"]
--js comma separated extensions indicate the file is js like
[string] [default: ".ts,.tsx,.mjs,.js,.jsx"]
--include included filenames regexp in string, default includes all files
[string] [default: ".*"]
--exclude excluded filenames regexp in string, set as empty string to
include all files [string] [default: "node_modules"]
-o, --output output json to file [string]
--tree print tree to stdout [boolean] [default: true]
--circular print circular to stdout [boolean] [default: true]
--warning print warning to stdout [boolean] [default: true]
--tsconfig the tsconfig path, which is used for resolve path alias, default
is tsconfig.json if it exists in context directory [string]
-T, --transform transform typescript modules to javascript before analyze, it
allows you to omit types dependency in typescript
[boolean] [default: false]
--exit-code exit with specified code, the value format is CASE:CODE,
`circular` is the only supported CASE, CODE should be a integer
between 0 and 128. For example: `dpdm --exit-code circular:1` the
program will exit with code 1 if circular dependency found.
[string]
--progress show progress bar [boolean] [default: true]
--detect-unused-files-from this file is a glob, used for finding unused files. [string]
--skip-dynamic-imports Skip parse import(...) statement.
[string] [choices: "tree", "circular"]
-h, --help Show help [boolean]
import { parseDependencyTree, parseCircular, prettyCircular } from 'dpdm';
parseDependencyTree('./index', {
/* options, see below */
}).then((tree) => {
const circulars = parseCircular(tree);
console.log(prettyCircular(circulars));
});
parseDependencyTree(entries, option, output)
: parse dependencies for glob entries
/**
* @param entries - the glob entries to match
* @param options - the options, see below
*/
export declare function parseDependencyTree(
entries: string | string[],
options: ParserOptions,
): Promise<DependencyTree>;
/**
* the parse options
*/
export interface ParseOptions {
context: string; // context to shorten filename, default is process.cwd()
extensions: string[]; // the custom extensions to resolve file, default is [ '.ts', '.tsx', '.mjs', '.js', '.jsx', '.json' ]
include: RegExp; // the files to parse match regex, default is /\.m?[tj]sx?$/
exclude: RegExp; // the files to ignore parse, default is /\/node_modules\//
}
export enum DependencyKind {
CommonJS = 'CommonJS', // require
StaticImport = 'StaticImport', // import ... from "foo"
DynamicImport = 'DynamicImport', // import("foo")
StaticExport = 'StaticExport', // export ... from "foo"
}
export interface Dependency {
issuer: string;
request: string;
kind: DependencyKind;
id: string | null; // the shortened, resolved filename, if cannot resolve, it will be null
}
// the parse tree result, key is file id, value is its dependencies
// if file is ignored, it will be null
export type DependencyTree = Record<string, Dependency[] | null>;
parseCircular(tree)
: parse circulars in dependency tree
export declare function parseCircular(tree: DependencyTree): string[][];
FAQs
Analyze circular dependencies in your JavaScript/TypeScript projects.
The npm package dpdm receives a total of 177,907 weekly downloads. As such, dpdm popularity was classified as popular.
We found that dpdm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.