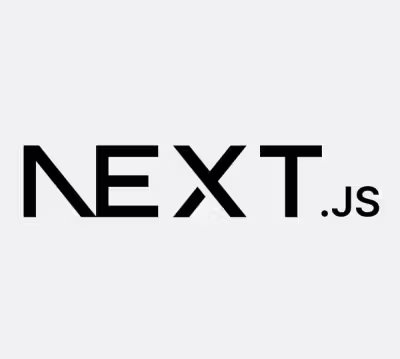
Security News
Next.js Patches Critical Middleware Vulnerability (CVE-2025-29927)
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
dui-active-record
Advanced tools
Implementation of the Active Record pattern for typescript
Works on Node.js and on browsers, with minimal dependecies
let FooValidator = new ModelValidator({
allowUnsafe: false,
strict : true,
rules : {
bar: ['int'],
},
});
let foo = {
bar : '123',
other: 'abc',
};
FooValidator.validate(foo);
// return false
FooValidator.allErrors(foo);
// return [
// new ValidationError(
// 'int',
// 'Value must be a integer',
// undefined,
// 'bar',
// ),
// new ValidationError(
// 'unsafe',
// 'Unsafe attributes is not allowed',
// undefined,
// 'other',
// ),
// ]
// FooValidator.errors(foo)
// {
// bar : [
// new ValidationError(
// 'int',
// 'Value must be a integer',
// undefined,
// 'bar',
// ),
// ],
// other: [
// new ValidationError(
// 'unsafe',
// 'Unsafe attributes is not allowed',
// undefined,
// 'other',
// ),
// ],
};
let foos = [
{
bar: 5,
}, {
bar: 10,
}
]
foos.map(FooValidator.validate);
foos.map(FooValidator.allErrors);
foos.map(FooValidator.errors);
let FooStorage = new ArrayStorage<IFoo>();
or
let FooStorage = MongoDB.storage<IFoo>('collection_name');
// single using
let foo = {
bar: 123,
};
FooStorage.save(foo);
FooStorage.save('someId', foo);
// array using
let foos: IFoo[] = [
{
bar: 5,
}, {
bar: 10,
}
]
foos.map(FooStorage.save);
@ActiveRecord({
storage: ArrayStorage
})
export class FooModel extends Model {
constructor(a?: string) {
super();
}
@Field({enumerable: false})
public a: string;
@Field()
public b: number = 0;
@Field()
public d: SubDoc;
@Field({enumerable: true})
get c(): string {
return 'c';
}
set c(value: string) {
console.log('set c value:', value);
}
rules() {
return [];
}
static stat() {
console.log('stat');
}
}
FAQs
Implementation of the Active Record pattern for typescript
The npm package dui-active-record receives a total of 19 weekly downloads. As such, dui-active-record popularity was classified as not popular.
We found that dui-active-record demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.