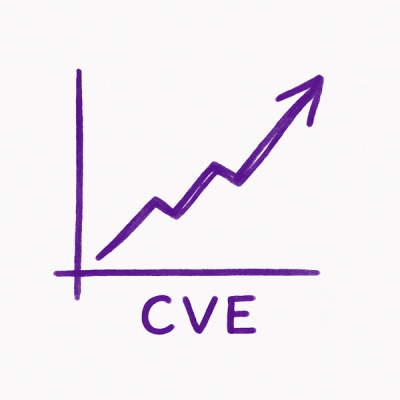
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
dynamic-xml-builder
Advanced tools
Dynamically create XML from JS objects.
const XMLObject = require('dynamic-xml-builder');
const data = new XMLObject('data');
data.user._type = 'person'
data.user.email = 'john@johnjohnnyandjohnson.com'
data.user.aliases.alias = ['John', 'Johnny', 'Johnson']
data.toXML()
<data>
<user type="person">
<email>john@johnjohnnyandjohnson.com</email>
<aliases>
<alias>John</alias>
<alias>Johnny</alias>
<alias>Johnson</alias>
</aliases>
</user>
</data>
PS. The examples use HTML because it's a well-known XML.
Object manipulation:
const XMLObject = require('dynamic-xml-builder');
var xml = new XMLObject('html');
// assign an attribute
xml._lang = 'en';
// assign a whole object
xml.head = {
meta: {
_charset: 'utf-8'
}
}
// go deeper
xml.head.title = 'example'
// or more complex
xml.body = {
div: {
_class: 'my-design',
p: [
'hello', 'how', 'are', 'you'
],
br: null
}
}
// or assign a property in a non-existing path
xml.body.div.div.p = 'great'
<html lang="en">
<head>
<meta charset="utf-8"/>
<title>example</title>
</head>
<body>
<div class="my-design">
<p>hello</p>
<p>how</p>
<p>are</p>
<p>you</p>
<br/>
<div>
<p>great</p>
</div>
</div>
</body>
</html>
Configure output formatting:
xml.toXML({
indent: 2, newLine: '\n'
})
<html lang="en">
<head>
<meta charset="utf-8"/>
<title>example</title>
</head>
<body>
<div class="my-design">
<p>hello</p>
<p>how</p>
<p>are</p>
<p>you</p>
<br/>
<div>
<p>great</p>
</div>
</div>
</body>
</html>
Every element in the object tree (except for assigned primitive values) is an XMLObject. Therefore the same functionality applies to those objects:
xml.head.toXML()
<head>
<meta charset="utf-8"/>
<title>example</title>
</head>
Output a plain object:
xml.head.toObject()
{ head: { meta: { _charset: 'utf-8' }, title: 'example' } }
When you need to directy assign value to element you can use a value selector (_value by default):
var xml = new XMLObject('a')
xml._href = 'https://www.example.com'
xml._value = 'Click me!'
<a href="https://www.example.com">Click me!</a>
Since this is based on ES6 proxies, then ES6 support is required:
new XMLObject('html')
new XMLObject('html', {head: {}, body: {}})
new XMLObject('html', {head: {}, body: {}}, ...options)
new XMLObject({html: {head: {}, body: {}}})
new XMLObject({html: {head: {}, body: {}}}, ...options)
Different options can be passed to the constructor or toXML(options) method
Name | Default | Usage | Description |
---|---|---|---|
attrSel | "_" | constructor | used to identify attributes (attrSel + attributeName, i.e. "_charset") |
valueSel | "_value" | constructor | used to set the element value directly |
defVal | "" | constructor | default value to use when element value has not been provided |
indent | "\t" | toXML | indent definition, can be any string |
newLine | "\r\n" | toXML | newline definition, can be any string |
attrKey | null | toXML | when provided, will group the attributes of an element under attrKey object |
declaration | null | toXML | provide true for the default declaration, or any string to override it |
selfClose | true | toXML | provice false to disable self-closing tags |
MIT
FAQs
dynamic XML builder
The npm package dynamic-xml-builder receives a total of 25 weekly downloads. As such, dynamic-xml-builder popularity was classified as not popular.
We found that dynamic-xml-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.