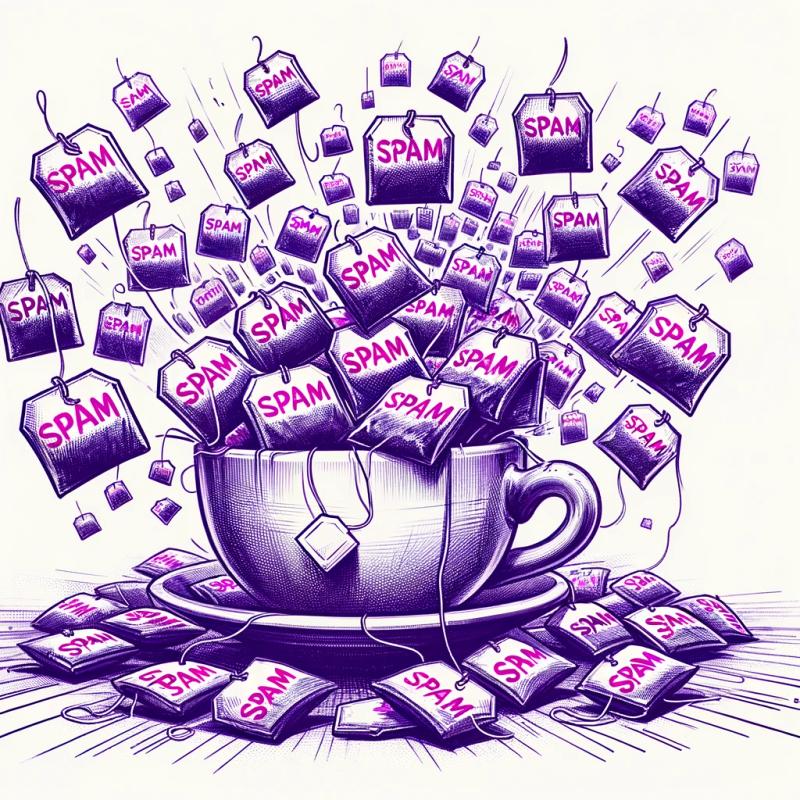
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
dynamoflow
Advanced tools
Readme
A practical & extendable DynamoDB client for TypeScript applications.
Unlike many other database technologies, DynamoDB expects your data management logic to live in the application layer. Rather than providing built-in features such as unique fields, foreign keys, or cascading deletes, DynamoDB provides the foundational technologies to implement these features yourself.
Rather than picking which of these features we do and don't include, DynamoFlow provides a set of tools to make it easy to implement these features yourself. We also provide a set of ready-to-go extensions for common patters, such as unique fields, foreign keys, secondary indexes & timestamping
There are several other Typescript DynamoDB clients available, and I encourage you to check them all out before committing to one.
My personal favourites are
npm install --save dynamoflow
or yarn add dynamoflow
npm install --save @aws-sdk/client-dynamodb @aws-sdk/lib-dynamodb
or yarn add @aws-sdk/client-dynamodb @aws-sdk/lib-dynamodb
docker run -p 8000:8000 amazon/dynamodb-local
(or copy our example docker-compose.yml file)import {DFTable} from "dynamoflow";
const table = new DFTable({
tableName: "my-application-table",
GSIs: ["GSI1", "GSI2"], // GSIs can be added later if needed
// using DynamoDBLocal
endpoint: "http://localhost:8000",
});
// not recommended for production use, but useful for local development
await table.createTableIfNotExists();
import {DFUniqueConstraintExt} from "dynamoflow";
interface User {
id: string;
name: string;
email: string;
}
const usersCollection = table.createCollection<User>({
name: "users",
partitionKey: "id",
extensions: [
new DFUniqueConstraintExt('email')
],
});
interface Project {
id: string;
userId: string;
name: string;
description: string;
status: "DRAFT" | "IN-PROGRESS" | "COMPLETED";
}
const projectsCollection = table.createCollection<Project>({
// the name of the collection is used to prefix the partition key for each item
name: "projects",
// any string, number or boolean fields of this entity can be used as keys
// different collections can have different keys
partitionKey: "userId",
sortKey: "id"
});
const user1 = await usersCollection.insert({
id: "user-1",
name: "John Smith",
email: "john.s@gmail.com"
});
const insertedProject = await projectsCollection.insert({
id: "project-1",
userId: user1.id,
name: "My First Project",
description: "This is my first project",
status: "DRAFT",
});
await projectsCollection.update({
id: "project-1"
}, {
status: "IN-PROGRESS"
});
const retrievedProject = await projectsCollection.retrieveOne({
where: {
userId: "user-1",
id: "project-1"
}
});
For a more comprehensive example, take a look at the included Messaging app demo.
Once you're ready to test against a real DynamoDB table, read Configuring DynamoDB Tables
FAQs
A practical & extendable DynamoDB client for TypeScript applications.
The npm package dynamoflow receives a total of 0 weekly downloads. As such, dynamoflow popularity was classified as not popular.
We found that dynamoflow demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.