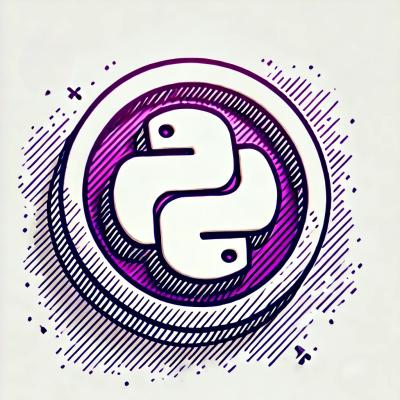
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The each-async npm package allows you to asynchronously iterate over an array, performing asynchronous operations on each item in the array. It is useful for scenarios where you need to handle asynchronous tasks in a sequential manner.
Asynchronous Iteration
This feature allows you to iterate over an array and perform asynchronous operations on each item. The `asyncOperation` function is called for each item in the array, and the `done` callback is called when the operation is complete. The final callback is called when all items have been processed.
const eachAsync = require('each-async');
const items = [1, 2, 3, 4, 5];
function asyncOperation(item, index, done) {
setTimeout(() => {
console.log('Processing item:', item);
done();
}, 1000);
}
eachAsync(items, asyncOperation, (err) => {
if (err) {
console.error('Error:', err);
} else {
console.log('All items have been processed.');
}
});
Error Handling
This feature demonstrates how to handle errors during the asynchronous iteration. If an error occurs during the processing of an item, the `done` callback is called with an error object, and the final callback receives the error.
const eachAsync = require('each-async');
const items = [1, 2, 3, 4, 5];
function asyncOperation(item, index, done) {
setTimeout(() => {
if (item === 3) {
done(new Error('An error occurred with item 3'));
} else {
console.log('Processing item:', item);
done();
}
}, 1000);
}
eachAsync(items, asyncOperation, (err) => {
if (err) {
console.error('Error:', err);
} else {
console.log('All items have been processed.');
}
});
The async package provides a wide range of utilities for working with asynchronous JavaScript. It includes functions for parallel and sequential execution, as well as error handling and control flow. Compared to each-async, async offers a more comprehensive set of tools for managing asynchronous operations.
Bluebird is a fully-featured Promise library for JavaScript. It provides powerful tools for managing asynchronous code, including methods for iterating over arrays with promises. Bluebird's `Promise.each` method offers similar functionality to each-async but with the added benefits of promise-based syntax and additional features.
The p-map package allows you to map over promises concurrently. It provides a simple and efficient way to handle asynchronous operations on arrays, with control over the concurrency level. Compared to each-async, p-map focuses on promise-based iteration and offers more control over concurrency.
Async parallel iterator
Like async.each, but smaller.
Download manually or with a package-manager.
npm install --save each-async
bower install --save each-async
component install sindresorhus/each-async
var eachAsync = require('each-async');
eachAsync(['foo','bar','baz'], function (item, index, done) {
console.log(item, index);
done();
}, function (error) {
console.log('finished');
});
//=> foo 0
//=> bar 1
//=> baz 2
//=> finished
<script src="bower_components/each-async/each-async.js"></script>
eachAsync(['foo','bar','baz'], function (item, index, done) {
console.log(item, index);
done();
}, function (error) {
console.log('finished');
});
//=> foo 0
//=> bar 1
//=> baz 2
//=> finished
The array you want to iterate.
A function which is called for each item in the array with the following arguments:
item
: the current item in the arrayindex
: the current indexdone([error])
: call this when you're done with an optional error. Supplying anything other than undefined
/null
will stop the iteration.Note that order is not guaranteed since each item is handled in parallel.
A function which is called when the iteration is finished or on the first error. First argument is the error passed from done()
in the callback
.
MIT © Sindre Sorhus
FAQs
Async concurrent iterator (async forEach)
The npm package each-async receives a total of 56,248 weekly downloads. As such, each-async popularity was classified as popular.
We found that each-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.