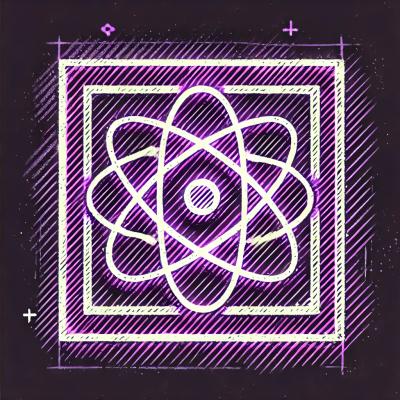
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
easy-mysql
Advanced tools
A small collection of simple functions that make using MySQL with node.js easier. Uses the node-mysql library.
Developed by MOG.com
npm install easy-mysql
EasyMySQL allows you to handle MySQL connection pooling, acquiring connections, and releasing them with ease. For example, where you might do this (using node-pool):
var get_widget = function(id, cb) {
pool.acquire(function (err, client) {
if (err) {
pool.release(client);
cb(err, null);
} else {
var sql = 'select * from widgets where id = ?';
client.query(sql, [id], function (err, results) {
pool.release(client);
if (err || results && results.length === 0) {
cb(err, null);
} else {
cb(null, results[0]);
}
});
}
});
};
...you can do this instead with EasyMySQL:
var get_widget = function(id, cb) {
var sql = 'select * from widgets where id = ?';
easy_mysql.get_one(sql, [id], cb);
};
// require the module
var EasyMySQL = require('easy-mysql');
You can connect three different ways with EasyMySQL:
Direct: Directly establish a single connection for each query. This is probably not a good idea for production code, but may be fine for code where you don't want to set up a pool, such as in unit tests.
Custom Pool: Pass in your own pool object. It must have functions named 'acquire' and 'release'.
Built-in Pool: Use the built-in pool, which uses node-pool
var settings = {
user : 'myuser',
password : 'mypass',
database : 'mydb'
};
var easy_mysql = EasyMySQL.connect(settings);
var my_pool = /* create your own pool here */;
var easy_mysql = EasyMySQL.connect_with_pool(pool);
var settings = {
user : 'myuser',
password : 'mypass',
database : 'mydb',
pool_size : 50
};
var easy_mysql = EasyMySQL.connect_with_easy_pool(settings);
You can specify a logger to log error events (more event types may be added later).
var settings = {
user : 'myuser',
password : 'mypass',
database : 'mydb',
logging: {
logger: my_logger,
events: {
error: {level: 'warn'}
}
}
};
You must pass in your own logger and it must have a function with the same
name as the level
you specify.
Returns only one result, and if no results are found, returns null.
var sql = 'select * from widgets where id = ?';
easy_mysql.get_one(sql, [123], function (err, result) {
cb(err, result);
});
Returns an array of results, and if no results are found, returns an empty array.
var sql = 'select * from widgets where id > ?';
easy_mysql.get_all(sql, [123], function (err, results) {
cb(err, results);
});
Executes an arbitrary SQL query and returns the results from node-mysql.
var sql = 'update widgets set foo = 'bar' where id = ?';
easy_mysql.execute(sql, [123], function (err, results) {
cb(err, results);
});
To run tests and generate docs, first run:
npm install -d
Run the tests:
make test
Generate JSDocs:
make doc
If you would like to contribute to the project, please fork it and send us a pull request. Please add tests
for any new features or bug fixes. Also run make lint
before submitting the pull request.
node-easy-mysql is licensed under the MIT license.
FAQs
Light MySQL query wrapper with connection pooling.
The npm package easy-mysql receives a total of 0 weekly downloads. As such, easy-mysql popularity was classified as not popular.
We found that easy-mysql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.