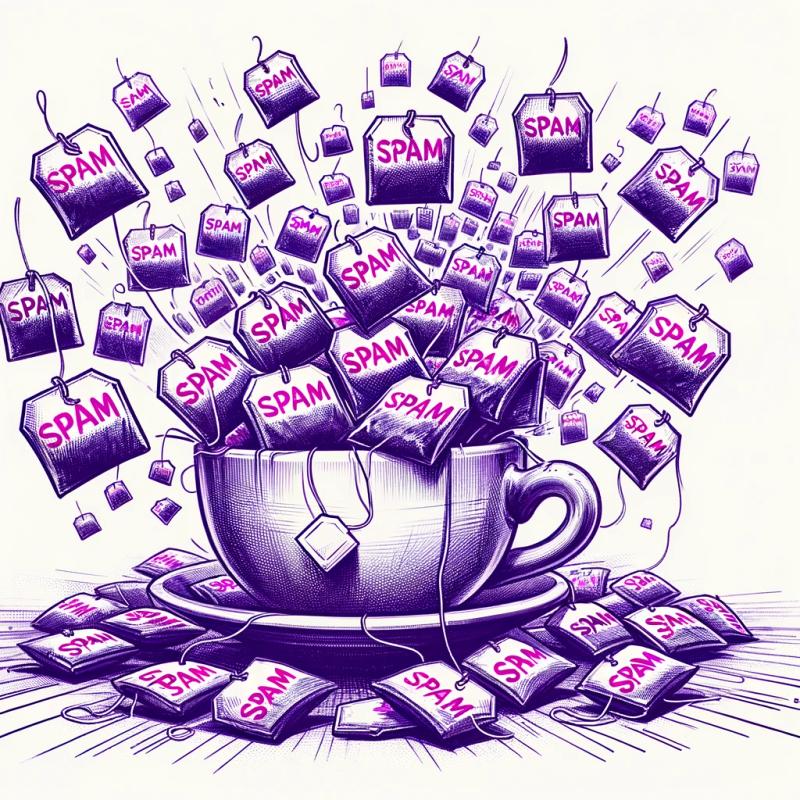
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ecommerce-basics
Advanced tools
Readme
Ecommerce Basics is a lightweight and easy-to-use npm library designed to simplify common e-commerce operations such as cart management, product calculations, and currency formatting. With a focus on simplicity and efficiency, this library provides essential functions to enhance the e-commerce experience in your applications.
To start using ecommerce-basics in your JavaScript projects, you can install it via npm:
npm install ecommerce-basics
Once you have installed ecommerce-basics, you can import the desired functions into your JavaScript code:
const ecommerce = require('ecommerce-basics');
ecommerce-basics offers a wide range of features:
const amount = 252.333334506
const formattedAmount = ecommerce.formatCurrency(amount);
console.log('Formatted Currency:', formattedAmount); // Output: $252.33
const cart = [{ id: 1, title: 'Product 1', price: 10, quantity: 2 }];
const total = ecommerce.calculateTotal(cart);
console.log('Total Price:', ecommerce.formatCurrency(total)); // Output: $20.00
const cart = [];
const productOne = { id: 1, title: 'Product 1', price: 10, quantity: 2 }
ecommerce.addToCart(cart, productOne);
console.log(cart); // Output: [{ id: 1, title: 'Product 1', price: 10, quantity: 2 }];
const productTwo = { id: 2, title: 'Product 2', price: 100, quantity: 1 }
ecommerce.addToCart(cart, productTwo);
console.log(cart);
/* Output:
[
{ id: 1, title: 'Product 1', price: 10, quantity: 2 },
{ id: 2, title: 'Product 2', price: 100, quantity: 1 }
];
/*
const productOne = { id: 1, title: 'Product 1', price: 10, quantity: 2 }
const productTwo = { id: 2, title: 'Product 2', price: 100, quantity: 1 }
const cart = [productOne, productTwo];
ecommerce.removeFromCart(cart, productOne);
console.log(cart[0]); // Output: [{ id: 1, title: 'Product 1', price: 10, quantity: 1 }];
console.log(cart[1]); // Output: [{ id: 2, title: 'Product 2', price: 100, quantity: 1 }];
ecommerce.removeFromCart(cart, productOne);
console.log(cart[0]); // Output: [{ id: 2, title: 'Product 2', price: 100, quantity: 1 }];
ecommerce.removeFromCart(cart, productTwo);
console.log(cart); // Output: []
const productOne = { id: 1, title: 'Product 1', price: 10, quantity: 4 }
const productTwo = { id: 2, title: 'Product 2', price: 100, quantity: 1 }
const cart = [productOne, productTwo];
ecommerce.removeProductFromCart(cart, productOne);
console.log(cart); // Output: [{ id: 2, title: 'Product 2', price: 100, quantity: 1 }];
console.log(cart.length); // Output: 1
ecommerce.removeProductFromCart(cart, productTwo);
console.log(cart.length); // Output: 0
const productOne = { id: 1, title: 'Product 1', price: 10, quantity: 4 }
const productTwo = { id: 2, title: 'Product 2', price: 100, quantity: 1 }
const cart = [productOne, productTwo];
const itemCount = ecommerce.calculateTotalItemCount(cart)
console.log(itemCount); // Output: 5
const productOne = { id: 1, title: 'Product 1', price: 10, quantity: 4 }
const productTwo = { id: 2, title: 'Product 2', price: 100, quantity: 1 }
const cart = [productOne, productTwo];
const updatedCart = ecommerce.clearCart(cart)
console.log(updatedCart); // Output: []
const apiUrl = 'https://example-api.com/products';
ecommerce.fetchProducts(apiUrl)
.then(products => {
console.log('Fetched Products:', products);
})
.catch(error => {
console.error('Error:', error);
});
I welcome contributions from the community to improve and expand the functionality of ecommerce-basics. If you have any suggestions, bug reports, or feature requests, please don't hesitate to open an issue or submit a pull request on the GitHub repository.
FAQs
This JS library is designed to simplify the development of e-commerce websites. It provides essential functionalities for building shopping carts, fetching products, and managing cart operations.
The npm package ecommerce-basics receives a total of 3 weekly downloads. As such, ecommerce-basics popularity was classified as not popular.
We found that ecommerce-basics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.