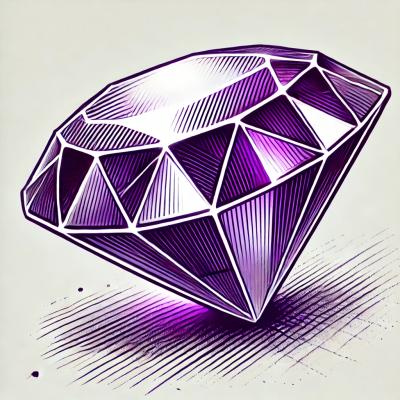
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
effector-final-form
Advanced tools
☄️ Effector bindings for Final Form 🏁
Forms values and validation rules are part of the business logic. This means that you need to be able to work with them without being bound to the UI-framework.
The goal of this project is to combine Final Form and Effector to achieve the above.
yarn add effector-final-form
# or
npm add effector-final-form
# or
pnpm add effector-final-form
Just import from the root module:
import { createForm } from 'effector-final-form';
import { createForm } from 'effector-final-form';
const form = createForm<{ login: string; password: string }>({
onSubmit: console.log,
subscribeOn: ['submitSucceeded', 'submitting'],
});
const loginField = form.api.registerField({
name: 'login',
subscribeOn: ['value', 'error', 'validating'],
validate: (x) => (x?.length >= 3 ? undefined : 'Incorrect login'),
});
loginField.$.watch(console.log);
// {name: "login", value: null, error: null, validating: true}
// {name: "login", error: "Incorrect login", value: null, validating: false}
const passwordField = form.api.registerField({
name: 'password',
subscribeOn: ['value', 'error', 'validating'],
validate: (x) => (x?.length >= 3 ? undefined : 'Incorrect password'),
});
passwordField.$.watch(console.log);
// {name: "password", value: null, error: null, validating: true}
// {name: "password", error: "Incorrect password", value: null, validating: false}
loginField.api.changeFx('John');
// {name: "login", error: null, value: "John", validating: true}
// {name: "login", error: null, value: "John", validating: false}
passwordField.api.changeFx('secret');
// {name: "password", error: null, value: "secret", validating: true}
// {name: "password", error: null, value: "secret", validating: false}
form.api.submitFx();
// {login: "John", password: "secret"}
0.10.0
value
typeFAQs
☄️ Effector bindings for Final Form
The npm package effector-final-form receives a total of 2 weekly downloads. As such, effector-final-form popularity was classified as not popular.
We found that effector-final-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.