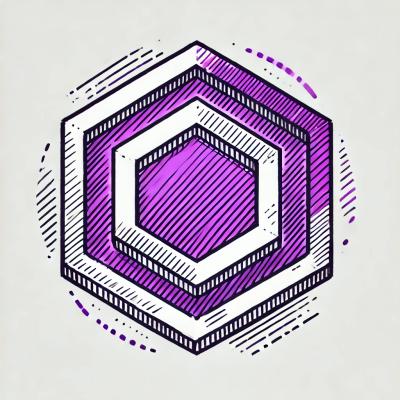
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
effects-as-data
Advanced tools
Effects-as-data is a micro abstraction layer for Javascript that makes writing, testing, and monitoring side-effects easy.
command
log allowing you to see every side-effect (HTTP, Disk IO, etc), its latency, and its result giving you detailed insight into your code while it runs in development and production.npm run perf
).This function creates a plain JSON command
object that effects-as-data will pass to a handler function which will perform the actual HTTP request. The type
field on the command matches the name of the handler to which it will be passed.
function httpGetCommand(url) {
return {
type: 'httpGet',
url
}
}
Effects-as-data uses a generator function's ability to give up execution flow and to pass a value to an outside process using the yield
keyword. You create command
objects in your business logic and yield
them to effects-as-data.
function* getPeople() {
const { results } = yield httpGetCommand('https://swapi.co/api/people')
const names = results.map(p => p.name)
return names
}
After the command
object is yield
ed, effects-as-data will pass it to a handler function that will perform the side-effect producing operation (in this case, an HTTP GET request).
function httpGetHandler(cmd) {
return fetch(cmd.url).then(r => r.json())
}
The effects-as-data config accepts an onCommandComplete
callback which will be called every time a command
completes, giving detailed information about the operation. This data can be logged to the console or sent to a logging service.
const config = {
onCommandComplete: telemetry => {
console.log('Telemetry (from onCommandComplete):', telemetry)
}
}
This will turn your effects-as-data functions into normal, promise-returning functions. In this case, functions
will be an object with one key, getPeople
, which will be a promise-returning function.
const functions = buildFunctions(
config,
{ httpGet: httpGetHandler },
{ getPeople }
)
Once you have built your functions, you can use them like normal promise-returning functions anywhere in your application.
functions
.getPeople()
.then(names => {
console.log('\n')
console.log('Function Results:')
console.log(names.join(', '))
})
.catch(console.error)
Turn your effects-as-data functions into normal promise-returning functions.
const { call, buildFunctions } = require('effects-as-data')
const fetch = require('isomorphic-fetch')
function httpGetCommand(url) {
return {
type: 'httpGet',
url
}
}
function httpGetHandler(cmd) {
return fetch(cmd.url).then(r => r.json())
}
function* getPeople() {
const { results } = yield httpGetCommand('https://swapi.co/api/people')
const names = results.map(p => p.name)
return names
}
const config = {
onCommandComplete: telemetry => {
console.log('Telemetry (from onCommandComplete):', telemetry)
}
}
const functions = buildFunctions(
config,
{ httpGet: httpGetHandler },
{ getPeople }
)
functions
.getPeople()
.then(names => {
console.log('\n')
console.log('Function Results:')
console.log(names.join(', '))
})
.catch(console.error)
const { call, buildFunctions } = require('../index')
const { cmds, handlers } = require('effects-as-data-universal')
// Pure business logic functions
function* getUsers() {
return yield cmds.httpGet('http://example.com/api/users')
}
function* getUserPosts(userId) {
return yield cmds.httpGet(`http://example.com/api/user/${userId}/posts`)
}
// Use onCommandComplete to gather telemetry
const config = {
onCommandComplete: telemetry => {
console.log('Telemetry:', telemetry)
}
}
// Turn effects-as-data functions into normal,
// promise-returning functions
const functions = buildFunctions(
config,
handlers, // command handlers
{ getUsers, getUserPosts } // effects-as-data functions
)
// Use the functions like you normally would
functions.getUsers().then(console.log)
FAQs
A micro abstraction layer for Javascript that makes writing, testing, and monitoring side-effects easy.
The npm package effects-as-data receives a total of 14 weekly downloads. As such, effects-as-data popularity was classified as not popular.
We found that effects-as-data demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.