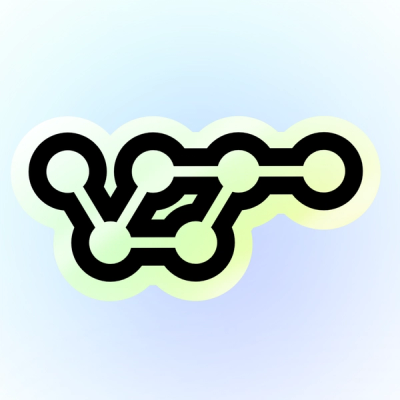
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
effects-as-data
Advanced tools
A micro abstraction layer for Javascript that makes writing, testing, and monitoring side-effects easy.
Effects-as-data is a micro abstraction layer for Javascript that makes writing and testing Javascript easier. Using effects-as-data dramatically reduces the time it takes to deliver highly tested code.
Consider this function that reads a config file based on a base path and NODE_ENV
. This simple function is hard to unit test and will probably take you a long time. It would require mocking process.env.NODE_ENV
, mocking/injecting readFile
, mocking logger.error
, and somehow forcing an error to test the error handling logic.
const { promisify } = require("util");
const fs = require('fs');
const readFile = promisify(fs.readFile)
const logger = require('logger')
async function readConfig(basePath) {
const { NODE_ENV } = process.env
const path = `${basePath}/${NODE_ENV}.json`;
const config = await readFile(path, { encoding: 'utf8' });
try {
return JSON.parse(config);
} catch (e) {
logger.error(e);
return {
default: 'config'
}
}
}
module.exports = {
readConfig
}
This is a drop-in replacement written using effects-as-data
:
const { globalVariable, call, fn, jsonParse, either, promisify } = require("effects-as-data");
const { readFile } = require('fs');
function* readConfig(basePath) {
const { env } = yield globalVariable('process');
const path = `${basePath}/${env.NODE_ENV}.json`;
const config = yield call.callback(readFile, path, { encoding: 'utf8' });
const parse = call.fn(JSON.parse, config);
return yield either(parse, { default: 'config' });
}
module.exports = {
readConfig: promisify(readConfig)
}
What normally requires mocks, spies, and other tricks for unit testing, effects-as-data
does simply and declaratively. The tests below tests all code branches in the function, tests the order in which side-effects occur and tests that everything is called the expected number of times and with the expected arguments:
const { testFn, args } = require('effects-as-data/test');
const { globalVariable, call, jsonParse } = require('effects-as-data');
const testReadConfig = testFn(readConfig);
test("readConfig() should return parsed config", testReadConfig(() => {
const basePath = '/foo'
const testProcess = { env: { NODE_ENV: 'development' } }
return args(basePath)
.cmd(globalVariable('process'))
.result(testProcess)
.cmd(callback(readFile, `${basePath}/development.json`, { encoding: 'utf8' }))
.result(`{"foo": "bar"}`)
.cmd(either(jsonParse(config), { default: 'config' }))
.result({ foo: 'bar' })
.returns({ foo: 'bar' })
}))
Generators are much more powerful than async/await
because generators allow developers to handle Javascript's most difficult problems all in one construct: asynchronous operations, non-determinism (ex: Date.now()
), and eliminate in most code the use of globals, singletons, closures for state, dependency injection, brittle promise chains, and mocks/spies. Generators, when used in effects-as-data
, allow developers to eliminate the anti-patterns that make Javascript hard to write, maintain and test.
FAQs
A micro abstraction layer for Javascript that makes writing, testing, and monitoring side-effects easy.
The npm package effects-as-data receives a total of 8 weekly downloads. As such, effects-as-data popularity was classified as not popular.
We found that effects-as-data demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.