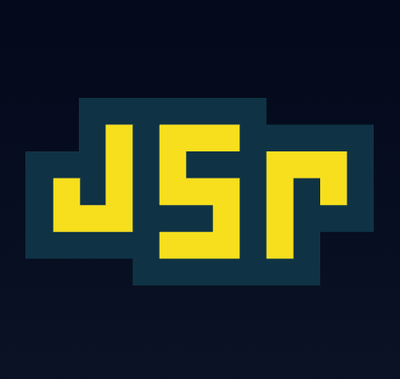
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
egg developer tool, extends common-bin.
npm i egg-bin --save-dev
Add egg-bin
to package.json
scripts:
{
"scripts": {
"dev": "egg-bin dev",
"debug": "egg-bin debug",
"test-local": "egg-bin test",
"test": "npm run lint -- --fix && npm run test-local",
"cov": "egg-bin cov",
"lint": "eslint .",
"pkgfiles": "egg-bin pkgfiles",
"autod": "egg-bin autod",
"ci": "npm run lint && npm run autod -- --check && npm run pkgfiles -- --check && npm run cov"
}
}
All the commands support these specific v8 options:
--debug
--inspect
--harmony*
--es_staging
egg-bin [command] --debug --es_staging
if process.env.NODE_DEBUG_OPTION
is provided (WebStorm etc), will use it as debug options.
Start dev cluster on local
env, it will start a master, an agent and a worker.
egg-bin dev
--framework
egg web framework root path.--baseDir
application's root path, default to process.cwd()
.--port
server port, default to 7001
.--workers
worker process number, default to 1
worker at local mode.--sticky
start a sticky cluster server, default to false
.--typescript
/ --ts
enable typescript support, default to false
. Also support read from package.json
's egg.typescript
.--declarations
/ --dts
enable egg-ts-helper support, default to false
. Also support read from package.json
's egg.declarations
.--require
will add to execArgv
, support multiple. Also support read from package.json
's egg.require
Debug egg app with V8 Inspector Integration.
automatically detect the protocol, use the new inspector
when the targeted runtime >=7.0.0 .
if running without VSCode
or WebStorm
, we will use inspector-proxy to proxy worker debug, so you don't need to worry about reload.
egg-bin debug --debug-port=9229 --proxy=9999
egg-bin dev
options is accepted.--proxy=9999
worker debug proxy port.Using mocha to run test.
egg-bin test [files] [options]
files
is optional, default to test/**/*.test.js
test/fixtures
, test/node_modules
is always exclude.test/.setup.js
If test/.setup.js
file exists, it will be auto require as the first test file.
test
├── .setup.js
└── foo.test.js
You can pass any mocha argv.
--require
require the given module--grep
only run tests matching <pattern>
--timeout
milliseconds, default to 60000--full-trace
display the full stack trace, default to false.--typescript
/ --ts
enable typescript support, default to false
.--changed
/ -c
only test changed test files(test files means files that match ${pwd}/test/**/*.test.(js|ts)
)--dry-run
/ -d
whether dry-run the test command, just show the command--parallel
enable mocha parallel mode, default to false
.--auto-agent
auto start agent in mocha master agent.--jobs
number of jobs to run in parallel, default to os.cpus().length - 1
.--mochawesome
enable mochawesome reporter, default to false
.Environment is also support, will use it if options not provide.
You can set TESTS
env to set the tests directory, it support glob grammar.
TESTS=test/a.test.js egg-bin test
And the reporter can set by the TEST_REPORTER
env, default is spec
.
TEST_REPORTER=doc egg-bin test
The test timeout can set by TEST_TIMEOUT
env, default is 60000
ms.
TEST_TIMEOUT=2000 egg-bin test
Using node:test to run test.
egg-bin node-test [files] [options]
files
is optional, default to test/**/*.test.js
test/fixtures
, test/node_modules
is always exclude.--test-only
configures the test runner to only execute top level tests that have the only option setTBD: TypeScript not support yet
Environment is also support, will use it if options not provide.
You can set TESTS
env to set the tests directory, it support glob grammar.
TESTS=test/a.test.js egg-bin node-test
And the reporter can set by the TEST_REPORTER
env, default is tap
.
TEST_REPORTER=doc egg-bin node-test
The test timeout can set by TEST_TIMEOUT
env, default is 60000
ms.
TEST_TIMEOUT=2000 egg-bin node-test
Using mocha and [c8] to run code coverage, it support all test params above.
Coverage reporter will output text-summary, json and lcov.
You can pass any mocha argv.
-x
add dir ignore coverage, support multiple argv
--prerequire
prerequire files for coverage instrument, you can use this options if load files slowly when call mm.app
or mm.cluster
--typescript
/ --ts
enable typescript support, default to false
, if true, will auto add .ts
extension and ignore typings
and d.ts
.
--c8
c8 instruments passthrough. you can use this to overwrite egg-bin's default c8 instruments and add additional ones.
- egg-bin have some default instruments passed to c8 like
-r
and--temp-directory
egg-bin cov --c8="-r teamcity -r text" --c8-report=true
--c8-report
use c8 to report coverage, c8 uses native V8 coverage, default to false
.
also support all test params above.
You can set COV_EXCLUDES
env to add dir ignore coverage.
COV_EXCLUDES="app/plugins/c*,app/autocreate/**" egg-bin cov
Using node:test and [c8] to run code coverage, it support all test params above.
Coverage reporter will output text-summary, json and lcov.
You can pass any node:test argv.
-x
add dir ignore coverage, support multiple argv
--prerequire
prerequire files for coverage instrument, you can use this options if load files slowly when call mm.app
or mm.cluster
--typescript
/ --ts
enable typescript support, default to false
, if true, will auto add .ts
extension and ignore typings
and d.ts
.
--c8
c8 instruments passthrough. you can use this to overwrite egg-bin's default c8 instruments and add additional ones.
- egg-bin have some default instruments passed to c8 like
-r
and--temp-directory
egg-bin cov --c8="-r teamcity -r text" --c8-report=true
--c8-report
use c8 to report coverage, c8 uses native V8 coverage, default to false
.
also support all node-test params above.
You can set COV_EXCLUDES
env to add dir ignore coverage.
COV_EXCLUDES="app/plugins/c*,app/autocreate/**" egg-bin node-test-cov
Generate pkg.files
automatically before npm publish, see ypkgfiles for detail
egg-bin pkgfiles
Generate pkg.dependencies
and pkg.devDependencies
automatically, see autod for detail
egg-bin autod
You maybe need a custom egg-bin to implement more custom features if your team has develop a framework base on egg.
Now you can implement a Command sub class to do that. Or you can just override the exists command.
See more at common-bin.
nsp has provide a useful security scan feature.
This example will show you how to add a new NspCommand
to create a new egg-bin
tool.
const EggBinCommand = require('egg-bin');
class MyEggBinCommand extends EggBinCommand {
constructor(rawArgv) {
super(rawArgv);
this.usage = 'Usage: egg-bin [command] [options]';
// load directory
this.load(path.join(__dirname, 'lib/cmd'));
}
}
module.exports = MyEggBinCommand;
const Command = require('egg-bin').Command;
class NspCommand extends Command {
async run({ cwd, argv }) {
console.log('run nsp check at %s with %j', cwd, argv);
}
description() {
return 'nsp check';
}
}
module.exports = NspCommand;
#!/usr/bin/env node
'use strict';
const Command = require('..');
new Command().start();
$ my-egg-bin nsp
run nsp check at /foo/bar with {}
atian25 | fengmk2 | popomore | whxaxes | dead-horse | hyj1991 |
---|---|---|---|---|---|
ngot | waitingsong | onlylovermb | snyk-bot | BiosSun | luckydrq |
stormslowly | snapre | ZYSzys | angleshe | ahungrynoob | yinseny |
mansonchor | okoala |
This project follows the git-contributor spec, auto updated at Sat Jun 04 2022 10:29:49 GMT+0800
.
5.12.2 (2023-01-11)
FAQs
egg developer tool
The npm package egg-bin receives a total of 16,395 weekly downloads. As such, egg-bin popularity was classified as popular.
We found that egg-bin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.