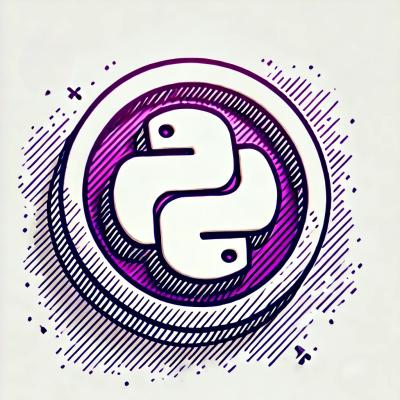
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
egg-mock
Advanced tools
Mock library for testing Egg applications, plugins and custom Egg frameworks with ease. egg-mock
inherits all APIs from node_modules/mm, offering more flexibility.
$ npm i egg-mock --save-dev
Launch a mock server with mm.app
// test/index.test.js
const path = require('path');
const mm = require('egg-mock');
describe('some test', () => {
let app;
before(() => {
app = mm.app({
baseDir: 'apps/foo'
});
return app.ready();
})
after(() => app.close());
it('should request /', () => {
return app.httpRequest()
.get('/')
.expect(200);
});
});
Retrieve Agent instance through app.agent
after mm.app
started.
Using mm.cluster
launch cluster server, you can use the same API as mm.app
;
baseDir
is optional that is process.cwd()
by default.
before(() => {
app = mm.app();
return app.ready();
});
framework is optional, it's node_modules/egg
by default.
before(() => {
app = mm.app({
baseDir: 'apps/demo',
framework: true,
});
return app.ready();
});
If eggPlugin.name
is defined in package.json
, it's a plugin that will be loaded to plugin list automatically.
before(() => {
app = mm.app({
baseDir: 'apps/demo',
});
return app.ready();
});
You can also test the plugin in different framework, e.g. test aliyun-egg and framework-b in one plugin.
describe('aliyun-egg', () => {
let app;
before(() => {
app = mm.app({
baseDir: 'apps/demo',
framework: path.join(__dirname, 'node_modules/aliyun-egg'),
});
return app.ready();
});
});
describe('framework-b', () => {
let app;
before(() => {
app = mm.app({
baseDir: 'apps/demo',
framework: path.join(__dirname, 'node_modules/framework-b'),
});
return app.ready();
});
});
If it's detected as an plugin, but you don't want it to be, you can use plugin = false
.
before(() => {
app = mm.app({
baseDir: 'apps/demo',
plugin: false,
});
return app.ready();
});
Create a mock application.
Create a mock cluster server, but you can't use API in application, you should test using supertest
.
const mm = require('egg-mock');
describe('test/app.js', () => {
let app, config;
before(() => {
app = mm.cluster();
return app.ready();
});
after(() => app.close());
it('some test', () => {
return app.httpRequest()
.get('/config')
.expect(200)
});
});
You can disable coverage, because it's slow.
mm.cluster({
coverage: false,
});
Mock env when starting
// production environment
mm.env('prod');
mm.app({
cache: false,
});
Environment list https://github.com/eggjs/egg-core/blob/master/lib/loader/egg_loader.js#L82
Mock level that print to stdout/stderr
// DON'T log to terminal
mm.consoleLevel('NONE');
level list: DEBUG
, INFO
, WARN
, ERROR
, NONE
mock home directory
restore all mock data, e.g. afterEach(mm.restore)
Options for mm.app
and mm.cluster
The directory of application, default is process.cwd()
.
mm.app({
baseDir: path.join(__dirname, 'fixtures/apps/demo'),
})
You can use a string based on $CWD/test/fixtures
for short
mm.app({
baseDir: 'apps/demo',
})
The directory of framework
mm.app({
baseDir: 'apps/demo',
framework: path.join(__dirname, 'fixtures/egg'),
})
It can be true when test an framework
The directory of plugin, it's detected automatically.
mm.app({
baseDir: 'apps/demo',
})
Define a list of plugins
Determine whether enable cache. it's cached by baseDir.
Clean all logs directory, default is true.
If you are using ava
, disable it.
Assert some string value in the logger instance.
It is recommended to pair app.mockLog()
with app.expectLog()
or app.notExpectLog()
.
Using app.expectLog()
or app.notExpectLog()
alone requires dependency on the write speed of the log. When the server disk is high IO, unstable results will occur.
it('should work', async () => {
app.mockLog();
await app.httpRequest()
.get('/')
.expect('hello world')
.expect(200);
app.expectLog('foo in logger');
app.expectLog('foo in coreLogger', 'coreLogger');
app.expectLog('foo in myCustomLogger', 'myCustomLogger');
app.notExpectLog('bar in logger');
app.notExpectLog('bar in coreLogger', 'coreLogger');
app.notExpectLog('bar in myCustomLogger', 'myCustomLogger');
});
Request current app http server.
it('should work', () => {
return app.httpRequest()
.get('/')
.expect('hello world')
.expect(200);
});
See supertest to get more APIs.
Assert current response not contains the specified header
it('should work', () => {
return app.httpRequest()
.get('/')
.unexpectHeader('set-cookie')
.expect(200);
});
Assert current response contains the specified header
it('should work', () => {
return app.httpRequest()
.get('/')
.expectHeader('set-cookie')
.expect(200);
});
const ctx = app.mockContext({
user: {
name: 'Jason'
}
});
console.log(ctx.user.name); // Jason
await app.mockContextScope(async ctx => {
console.log(ctx.user.name); // Jason
}, {
user: {
name: 'Jason'
}
});
app.mockCookies({
foo: 'bar'
});
const ctx = app.mockContext();
console.log(ctx.getCookie('foo'));
Mock request header
app.mockSession({
foo: 'bar'
});
const ctx = app.mockContext();
console.log(ctx.session.foo);
it('should mock user name', function* () {
app.mockService('user', 'getName', function* (ctx, methodName, args) {
return 'popomore';
});
const ctx = app.mockContext();
yield ctx.service.user.getName();
});
You can mock an error for service
app.mockServiceError('user', 'home', new Error('mock error'));
app.mockCsrf();
return app.httpRequest()
.post('/login')
.expect(302);
Mock httpclient request, e.g.: ctx.curl
app.get('/', function*() {
const ret = yield this.curl('https://eggjs.org');
this.body = ret.data.toString();
});
app.mockHttpclient('https://eggjs.org', {
// can be buffer / string / json / function
// will auto convert to buffer
// follow options.dataType to convert
data: 'mock egg',
});
// app.mockHttpclient('https://eggjs.org', 'get', mockResponse); // mock get
// app.mockHttpclient('https://eggjs.org', [ 'get' , 'head' ], mockResponse); // mock get and head
// app.mockHttpclient('https://eggjs.org', '*', mockResponse); // mock all methods
// app.mockHttpclient('https://eggjs.org', mockResponse); // mock all methods by default
// app.mockHttpclient('https://eggjs.org', 'get', function(url, opt) { return 'xxx' }); // support fn
return app.httpRequest()
.post('/')
.expect('mock egg');
You can also use Regular Expression for matching url.
app.mockHttpclient(/\/users\/[a-z]$/i, {
data: {
name: 'egg',
},
});
You can alse mock agent.httpclient
app.agent.mockHttpclient('https://eggjs.org', {
data: {
name: 'egg',
},
});
We also provide a bootstrap file for applications' unit test to reduce duplicated code:
const { app, mock, assert } = require('egg-mock/bootstrap');
describe('test app', () => {
it('should request success', () => {
// mock data will be restored each case
mock.data(app, 'method', { foo: 'bar' });
return app.httpRequest()
.get('/foo')
.expect(res => {
assert(!res.headers.foo);
})
.expect(/bar/);
});
});
EGG_BASE_DIR: the base dir of egg app EGG_FRAMEWORK: the framework of egg app
Please open an issue here.
popomore | fengmk2 | atian25 | dead-horse | shepherdwind | shaoshuai0102 |
---|---|---|---|---|---|
mansonchor | whxaxes | brickyang | zbinlin | GoodMeowing | ngot |
geekdada | shinux | zhang740 | caoer | lidianhao123 | limerickgds |
paranoidjk | gxcsoccer | okoala | ImHype | linjiajian999 |
This project follows the git-contributor spec, auto updated at Fri Apr 29 2022 22:49:14 GMT+0800
.
FAQs
mock server for egg
We found that egg-mock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.