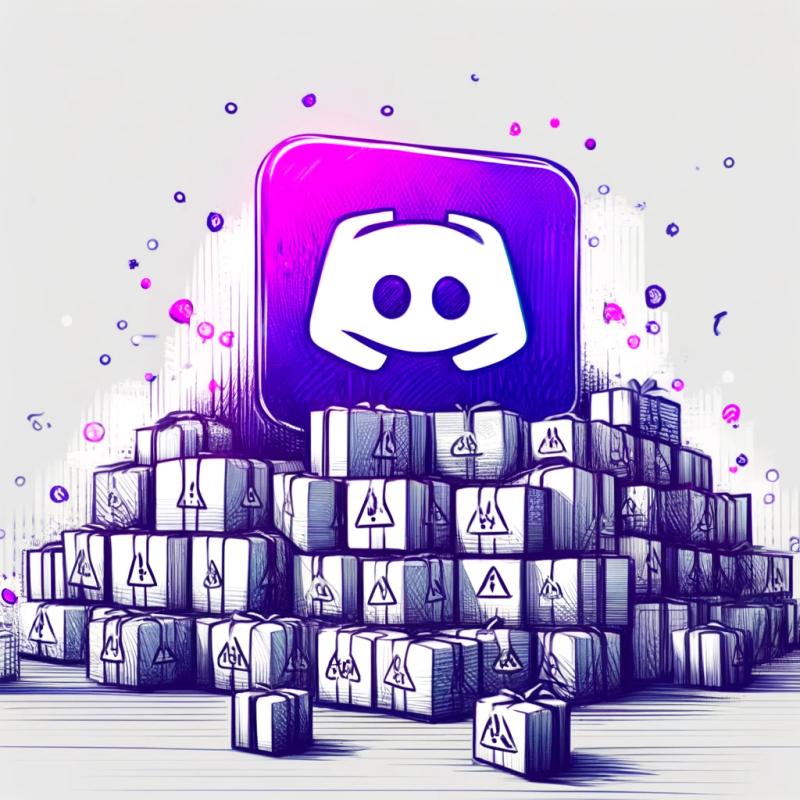
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
el-state
Advanced tools
Readme
Type safe state management for React.
combineReducers
to create root reducer. You can select multiple stores in single component. Action can dispatch other actions that belong to different store.Please see example.
import React from 'react';
import ReactDOM from 'react-dom';
import { StoreProvider } from 'el-state';
import App from './App';
ReactDOM.render(
<StoreProvider>
<App />
</StoreProvider>,
document.getElementById('root')
);
import { createStore } from 'el-state';
type CounterState = {
counter: number;
};
// Store
export const counterStore = createStore<CounterState>('counter', { counter: 0 });
// Update state by merging
export const setCounter = createAction(counterStore, ({ mergeState }, counter: number) => mergeState({ counter }));
// Dispatch other action. You can also dispatch action that belong to different store
export const resetCounter = createAction(counterStore, ({ dispatch }) => dispatch(setCounter, 0));
// Update state by returning new one. You can't mutate `state` because it's read only.
export const increaseCounter = createAction(counterStore, ({ state }) => ({ ...state, counter: state.counter + 1 }));
function MyComponent() {
const counter: number = useStore(counterStore, state => state.counter);
// use the counter
return <div>{counter}</div>;
}
const useSelector = createSelector(getStore => {
const { counter } = getStore(counterStore);
const { name } = getStore(accountStore);
return counter > 1 ? name : '';
});
function MyComponent() {
// this component will only rerender if the value of useSelector changed
// it will not rerender when the counterStore updated from 1 to 2
const name = useSelector();
// use the counter
return <div>{name}</div>;
}
function MyComponent() {
const onIncrease = useAction(increaseCounter);
// use this function as onClick handle
<button onClick={onIncrease}>Increase</button>;
}
Or
function MyComponent() {
const dispatch = useDispatcher();
const onIncrease = () => dispatch(increaseCounter);
// use this function as onClick handle
}
function MyComponent() {
const onChange = useActionCallback(setCounter, (e: React.ChangeEvent<HTMLInputElement>) => [
parseInt(e.target.value, 10), // you must parse the value, because first argument of setCounter is number
]);
// use this function as event handler
return <input value={counter} onChange={onChange} />;
}
FAQs
Type safe state management for React.
We found that el-state demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.