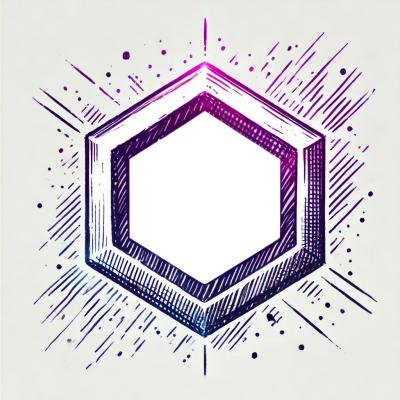
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
elastic-orm
Advanced tools
ElasticORM is an ORM tool that can run in javascript and helps developers to define a mapping of entities in one place by using decorators.
Install package from npm.
npm i elastic-orm @elastic/elasticsearch
import { EsEntity, EsProperty, EsId } from 'elastic-orm';
@EsEntity('elastic_index') // specify elastic index
export class MyEntity {
@EsId()
public id: string;
@EsProperty('integer')
public foo: number;
@EsProperty({ type: 'keyword' })
public bar: string;
@EsProperty('boolean')
public fingersCrossedForUkraine: boolean = true;
@EsProperty('date')
public createdAt: Date;
}
import { EsRepository } from 'elastic-orm';
import { Client } from '@elastic/elasticsearch';
const repository = new EsRepository(
MyEntity,
new Client(/* client configuration */),
);
Client configuration is provided here https://www.elastic.co/guide/en/elasticsearch/client/javascript-api/7.17/client-connecting.html
You can easily create index from you entity definition by running:
import { FactoryProvider } from 'elastic-orm';
const schema =
FactoryProvider.makeSchemaManager().generateIndexSchema(MyEntity);
await repository.createIndex(schema);
const myEntity = new MyEntity()
myEntity.foo = 99
myEntity.bar = 'hello elastic search'
myEntity.isTutorial = true
myEntity.createdAt = new Date()
const createdEntity = await repository.create(myEntity)
const {entities, raw} = await repository.find({
query: {
term: {
foo: 99,
},
},
})
Repository requires specific elastic structure object by default. Thanks to typescript query can be written really easily with autosuggestion. It will hint also properties from defined entity.
If you are more familiar with builders. Bodybuilder can be used easily.
npm i bodybuilder
import * as bodybuilder from 'bodybuilder';
const body = bodybuilder().query('match', 'foo', 1).build();
const res = await repository.findOne(body);
import { EsEntity, EsProperty, EsId } from 'elastic-orm';
@EsEntity('elastic_index')
export class MyEntity {
@EsId()
public id: string;
@EsProperty('integer')
public foo: number;
@EsProperty({ type: 'nested', entity: MyNestedEntity })
public nestedItem: MyNestedEntity;
@EsProperty({ type: 'nested', entity: MyNestedEntity })
public nestedItems: MyNestedEntity[];
}
export class MyNestedEntity {
@EsProperty('keyword')
public name: string;
}
// query for nested entities
const res = await repository.findOne({
query: {
nested: {
path: 'nestedItem',
query: {
bool: {
must: [{ match: { 'nestedItem.name': 'find this string' } }],
},
},
},
},
});
Identifier is automatically generated. But if you want to control generation of ids, you can use this approach:
@EsEntity('elastic_index')
export class MyEntity {
@EsId({
generator: () => {
// apply custom logic here
return 'customGeneratedId';
},
})
public id: string;
}
For additional property options use parameter additionalFieldOptions
.
import { EsEntity, EsProperty, EsId } from 'elastic-orm';
@EsEntity('elastic_index')
export class MyEntity {
@EsId()
public id: string;
@EsProperty('text', {
additionalFieldOptions: {
enabled: false,
fields: {
raw: {
type: 'keyword',
},
},
},
})
public foo: string;
}
@EsEntity('elastic_index', {
aliases: ['elastic_index_alias_read', 'elastic_index_alias_write'],
settings: {
number_of_replicas: 1,
number_of_shards: 5,
// and other settings definitions
},
mapping: {
dynamic: 'strict',
},
})
export class MyEntity{}
import { FactoryProvider } from 'elastic-orm';
const mapping = FactoryProvider.makeSchemaManager().buildMapping(
FactoryProvider.makeMetaLoader().getReflectMetaData(TestingNestedClass),
);
await repository.updateMapping(mapping);
await repository.deleteIndex();
Enhancing elastic search requests is sometimes useful in one place. To do so you can register custom function which will be executed before every request on elastic.
For example:
repository.on('beforeRequest', (action, esParams, args) => {
if (process.env.NODE_ENV !== 'production') {
esParams.explain = true;
}
});
repository.on('beforeRequest', (action, esParams, args) => {
if (action === 'find') {
esParams.index = 'elastic_index_alias_read';
}
});
FAQs
Elasticsearch object relational mapper.
The npm package elastic-orm receives a total of 0 weekly downloads. As such, elastic-orm popularity was classified as not popular.
We found that elastic-orm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.