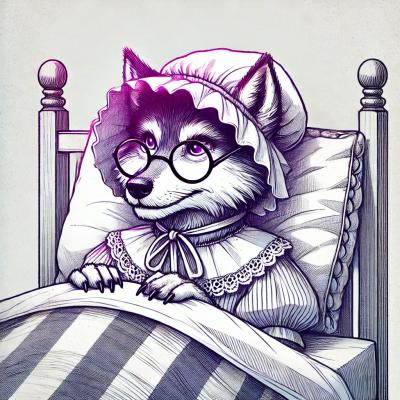
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
elasticsearch
Advanced tools
The elasticsearch npm package is a client library for interacting with Elasticsearch, a distributed search and analytics engine. This package allows developers to perform a variety of operations such as indexing documents, searching, and managing indices within an Elasticsearch cluster.
Indexing Documents
This feature allows you to index documents into an Elasticsearch index. The code sample demonstrates how to create a client, connect to an Elasticsearch node, and index a document into 'my-index'.
const { Client } = require('@elastic/elasticsearch');
const client = new Client({ node: 'http://localhost:9200' });
async function run() {
await client.index({
index: 'my-index',
document: {
title: 'Test Document',
content: 'This is a test document.'
}
});
console.log('Document indexed');
}
run().catch(console.log);
Searching Documents
This feature allows you to search for documents within an Elasticsearch index. The code sample demonstrates how to search for documents in 'my-index' where the title matches 'Test'.
const { Client } = require('@elastic/elasticsearch');
const client = new Client({ node: 'http://localhost:9200' });
async function run() {
const result = await client.search({
index: 'my-index',
query: {
match: { title: 'Test' }
}
});
console.log(result.hits.hits);
}
run().catch(console.log);
Managing Indices
This feature allows you to manage indices in Elasticsearch. The code sample demonstrates how to create a new index called 'my-new-index'.
const { Client } = require('@elastic/elasticsearch');
const client = new Client({ node: 'http://localhost:9200' });
async function run() {
await client.indices.create({
index: 'my-new-index'
});
console.log('Index created');
}
run().catch(console.log);
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It provides a schema-based solution to model your application data. While it is similar in that it interacts with a database, it is specific to MongoDB and offers features like schema validation and middleware, which are not provided by the elasticsearch package.
Redis is a fast, open-source, in-memory key-value data store. The redis npm package allows you to interact with a Redis database. While it offers some overlapping functionalities like data storage and retrieval, it is designed for different use cases such as caching and real-time analytics, unlike Elasticsearch which is optimized for full-text search and complex queries.
Couchbase is a distributed NoSQL cloud database. The couchbase npm package allows you to interact with a Couchbase server. It offers similar functionalities like indexing and querying documents but is designed for high-performance applications requiring low-latency data access, whereas Elasticsearch is optimized for search and analytics.
This is a Node.js module for the elasticsearch REST API.
npm install elasticsearch
var
elasticsearch = require('elasticsearch'),
config = {
_index : 'kittehs'
},
es = elasticsearch(config);
es.search({
query : {
field : {
animal : 'kitteh'
}
}
}, function (err, data) {
// work with data here
// response data is according to ElasticSearch spec
});
Unless otherwise stated, all callback signatures are function (err, data)
, with data
being the parsed JSON response from elasticsearch.
Calling elasticsearch.createClient(config)
is the same as elasticsearch(config)
.
var
elasticsearch = require('elasticsearch'),
es = elasticsearch.createClient(config);
You may also supply a logger for each request by passing in an optional second argument to elasticsearch.createClient(config, requestLogger)
. This will result in every http(s) request being logged via the callback supplied to the requestLogger
parameter.
var
elasticsearch = require('elasticsearch'),
es = elasticsearch.createClient(config, console.log);
When initializing the library, you may choose to specify an index and/or type to work with at the start to save from having to supply this information in the options for each operation request:
var config = {
_index : 'pet',
_type : 'kitteh'
};
Additionally, if working with multiple indexes or types, you may specify them as arrays:
var config = {
_indices : ['pet', 'family'],
_types : ['kitteh', 'canine']
};
Note: When index, indices, type or types are supplied via operation options, those settings will take precident over the base configuration for the library:
var
elasticsearch = require('elasticsearch'),
config = {
_index : 'kitteh'
},
es = elasticsearch.createClient(config);
es.indices.exist({ _index : 'canine' }, function (err, data) {
// will result in a HEAD request to /canine instead of /kitteh
});
If omitted from configuration, the server settings default to the following:
var config = {
// optional - when not supplied, defaults to the following:
server : {
host : 'localhost',
port : 9200
}
};
Anything specified within the server element of config is passed directly through to each HTTP/HTTPS request. You may configure additional options for connecting to Elasticsearch:
var config = {
server : {
agent : false,
auth : 'user:pass',
host : 'localhost',
port : 9243,
rejectUnauthorized : false,
secure : true // toggles between https and http
}
};
Elasticsearch is pretty much rad at clustering. If you want to specify multiple servers to failover to, you may do so by either supplying an array as the value for the property hosts
or hostnames
:
var elasticsearch = require('elasticsearch');
var config = {
_index : 'bawss',
server : {
hostnames : ['es1.myhost.com', 'es2.myhost.com', 'es3.myhost.com']
secure : true
}
};
var es = elasticsearch(config);
If you run on different ports for each server, use the hosts
property:
var elasticsearch = require('elasticsearch');
var config = {
_index : 'bawss',
server : {
hosts : ['localhost:9200', 'localhost:9201', 'localhost:9202']
}
};
var es = elasticsearch(config);
The default timeout for any operation against Elasticsearch is set at 30 seconds. You can override this value by specifying a timeout property in the options for the operation:
var options = {
timeout : 60000 // 60 seconds
};
es.bulk(options, commands, function (err, data) {
// teh datas
});
For each ES operation, options may be specified as the first argument to the function. In most cases, these are entirely optional, but when supplied, the values specified will take precident over the config values passed to the library constructor. Additionally, if there are extra option keys supplied beyond what is required for the operation, they are mapped directly to the querystring.
var options = {
_index : 'bawss',
_type : 'man',
refresh : true
};
var doc = {
field1 : 'test value'
};
es.index(options, doc, function (err, data) {
// this will result in a POST with path /bawss/man?refresh=true
});
For more specifics and details regarding the core API for ElasticSearch, please refer to the documentation at http://www.elasticsearch.org/guide/reference/api/.
Please Note: The default timeout is set at 30 seconds... if you are performing a large bulk insert you may need to increase this limit by specifying a higher value for timeout
in the options parameter.
This method doesn't take into account the underlying config that was used when instantiating the client. It requires index and type to be specified via the commands array or via the options parameter. Conflict will occur if one specifies a different index and type in the options than what is specified via the commands parameter.
At a high level, when performing a bulk update, you must supply an array with an action object followed by the object that the action will use during execution. In the following example, the first item in the array specifies the action is index
and the second item represents the data to index:
[
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'hamish', breed : 'manx', color : 'tortoise' }
]
In this example, two index
actions will be performed on the 'dieties' index and 'kitteh' type in ElasticSearch:
[
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'dugald', breed : 'siamese', color : 'white' },
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'keelin', breed : 'domestic long-hair', color : 'russian blue' }
]
For more information regarding bulk, please see the ElasticSearch documentation at http://www.elasticsearch.org/guide/reference/api/bulk/
es.bulk(options, commands, callback)
var
elasticsearch = require('elasticsearch'),
es = elasticsearch();
var commands = [
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'hamish', breed : 'manx', color : 'tortoise' },
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'dugald', breed : 'siamese', color : 'white' },
{ index : { _index : 'dieties', _type : 'kitteh' } },
{ name : 'keelin', breed : 'domestic long-hair', color : 'russian blue' }
];
es.bulk(commands, function (err, data) {
// teh datas
});
This is not a core action for ElasticSearch, but is a convenience method added to this ElasticSearch client to make bulk indexing more straight forward. Simply supply an array of documents you wish to bulk index in ElasticSearch and the method will take of the details for you.
es.bulkIndex(options, documents, callback)
var
elasticsearch = require('elasticsearch'),
es = elasticsearch();
var documents = [
{ name : 'hamish', breed : 'manx', color : 'tortoise' },
{ name : 'dugald', breed : 'siamese', color : 'white' },
{ name : 'keelin', breed : 'domestic long-hair', color : 'russian blue' }
];
var options = {
_index : 'dieties',
_type : 'kitteh'
}
es.bulkIndex(options, documents, function (err, data) {
// teh datas
});
es.count(options, callback)
var
elasticsearch = require('elasticsearch');
es = elasticsearch();
es.count(function (err, data) {
// teh datas
});
// count docs in a specific index/type
var options = {
_index : 'bawss',
_type : 'kitteh'
}
es.count(options, function (err, data) {
// counted... like a bawss
});
Requires _index
be specified either via lib config (as shown below) or via options when calling the operation.
es.delete(options, callback)
var
elasticsearch = require('elasticsearch'),
es = elasticsearch();
core.delete({ _id : 'mbQZc_XhQDWmNCQX5KwPeA' }, function (err, data) {
// teh datas
});
Requires _index
be specified either via lib config (as shown below) or via options when calling the operation.
es.deleteByQuery(options, query, callback)
var
elasticsearch = require('elasticsearch'),
es = elasticsearch({ _index : 'kitteh' });
var query = {
query : {
field : { breed : 'siamese' }
}
};
es.deleteByQuery(query, function (err, data) {
// teh datas
});
Requires _index
be specified either via lib config or via options when calling the operation.
es.exists(options, callback)
var
elasticsearch = require('elasticsearch'),
es = elasticsearch();
es.exists({ _index : 'kitteh' }, function (err, data) {
// teh datas
});
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
Also requires _id
, but this must be specified via options.
es.explain(options, query, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
Also requires _id
, but this must be specified via options.
es.get(options, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
es.index(options, doc, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
Also requires _id
, but this must be specified via options.
es.moreLikeThis(options, callback)
If _index
and/or _type
are supplied via options (or lib config), the will applied to the doc that is transmitted for the operation.
es.multiGet(options, docs, callback)
es.multiSearch(options, queries, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
es.percolate(options, doc, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
Also requires name
, but this must be specified via options.
es.registerPercolator(options, query, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
Also requires name
, but this must be specified via options.
es.unregisterPercolator(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.search(options, query, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
Also requires _id
, but this must be specified via options.
es.update(options, doc, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.validate(options, query, callback)
All operations here interact with the indices segment of the Elasticsearch API.
es.indices.alias(options, data, callback)
Requires alias
, but this must be specified via options.
es.indices.aliases(options, callback)
es.indices.analyze(options, data, callback)
es.indices.clearCache(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.closeIndex(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.createIndex(options, data, callback)
Requires name
, but this must be specified via options.
es.indices.createTemplate(options, template, callback)
Requires _index
and _alias
be specified either via lib config or via options when calling the operation.
es.indices.deleteAlias(opitons, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.deleteIndex(options, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
es.indices.deleteMapping(options, callback)
Requires name
, but this must be specified via options.
es.indices.deleteTemplate(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
Also requires name
, but this must be specified via options.
es.indices.deleteWarmer(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.exists(options, callback)
es.indices.flush(options, callback)
es.indices.mappings(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.openIndex(options, callback)
es.indices.optimize(options, callback)
Requires _index
and _type
be specified either via lib config or via options when calling the operation.
es.indices.putMapping(options, mapping, callback)
Requires name
, but this must be specified via options.
es.indices.putWarmer(options, warmer, callback)
es.indices.refresh(options, callback)
es.indices.segments(options, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.settings(options, callback)
es.indices.snapshot(options, callback)
es.indices.stats(options, callback)
es.indices.status(options, callback
Requires name
, but this must be specified via options.
es.indices.templates(options, callback)
es.indices.updateSettings(options, settings, callback)
Requires _index
be specified either via lib config or via options when calling the operation.
es.indices.warmers(options, callback)
All operations here interact with the Cluster portion of the Elasticsearch API.
Requires name
, but this must be specified via options.
es.cluster.deleteRiver(options, callback)
Requires field
or fields
, but this must be specified via options.
es.cluster.fieldStats(options, callback)
es.cluster.health(options, callback)
es.cluster.hotThreads(options, callback)
es.cluster.nodesInfo(options, callback)
es.cluster.nodesStatus(options, callback)
Requires name
, but this must be specified via options.
es.cluster.putRiver(options, meta, callback)
es.cluster.reroute(options, commands, callback)
Requires name
, but this must be specified via options.
es.cluster.rivers(options, callback)
es.cluster.settings(options, callback)
es.cluster.shutdown(options, callback)
es.cluster.state(options, callback)
es.cluster.updateSettings(options, updates, callback)
Note that a test coverage report is sent to coveralls.io during CI... running locally will result in a response similar to Bad response: 500 {"message":"Build processing error.","error":true,"url":""}
.
Code coverage data generated from npm test is located in ./lib-cov
and is not included in the git repo.
npm install
npm test
To run code coverage and generate local report at ./reports/coverage.html
:
npm run-script coverage
MIT
FAQs
The official low-level Elasticsearch client for Node.js and the browser.
The npm package elasticsearch receives a total of 164,056 weekly downloads. As such, elasticsearch popularity was classified as popular.
We found that elasticsearch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.