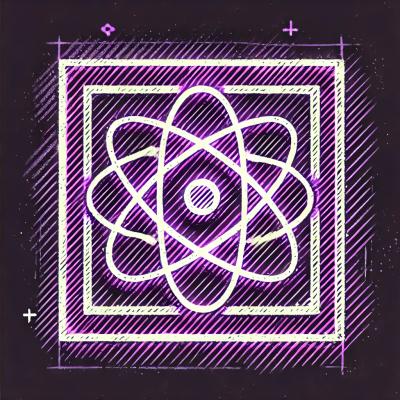
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
elf-hyperbee-persist
Advanced tools
elf-hyperbee-persist is a library for managing state persistence and synchronization between a Hyperbee instance and an RxJS Elf store. These utilities ensure that state changes are efficiently loaded and persisted using reactive programming principles.
[prefix, key]
formats and direct keys.Install the package via npm:
npm install elf-hyperbee-persist
elf-hyperbee-persist
is designed to work in both Node.js and Bare environments.
Node.js Compatibility:
import
).Bare Environment Compatibility:
bare:test
to ensure functionality in Bare-based runtimes.To ensure compatibility, both standard and Bare tests are included:
# Standard Node.js test
npm test
# Test in Bare runtime
npm run bare:test
loadStateFromHyperbee$
import { loadStateFromHyperbee$ } from "elf-hyperbee-persist";
Loads persisted state from Hyperbee into an RxJS Elf store.
hyperbee
: The Hyperbee instance to read the state from.elfStore
: The store where the loaded state will be applied.options.prefix
(default: "state"
): If provided, filters keys that start with this prefix.
prefix
is null
, keys are assumed to be stored as direct keys instead of [prefix, key]
.An RxJS observable that, when subscribed to, loads the state into the Elf store.
// Using prefixed keys
loadStateFromHyperbee$(hyperbeeInstance, myElfStore, { prefix: "gameState" }).subscribe();
// Using non-prefixed keys
loadStateFromHyperbee$(hyperbeeInstance, myElfStore, { prefix: null }).subscribe();
persistStateIntoHyperbee$
import { persistStateIntoHyperbee$ } from "elf-hyperbee-persist";
Watches for state changes in the Elf store and persists them into Hyperbee.
hyperbee
: The Hyperbee instance to persist the state into.elfStore
: The RxJS Elf store tracking state changes.options.prefix
(default: "state"
): If provided, persists state using [prefix, key]
format.
prefix
is null
, stores keys directly without structuring them as arrays.options.debounce
(default: 1000
): Debounce time in milliseconds before persisting updates.options.cas
(default: a deep equality check): A compare-and-swap (CAS) function that determines whether an entry should be updated in Hyperbee.
prev
(previous entry) and curr
(new entry).true
if the entry should be updated.options.distinct
(default: a deep equality check): A function that determines whether state updates are considered distinct.
distinctUntilChanged
to filter redundant updates.true
if they are the same (i.e., no need to update).An RxJS observable that processes state updates and saves them to Hyperbee.
// Using prefixed keys
persistStateIntoHyperbee$(hyperbeeInstance, myElfStore, { prefix: "gameState" }).subscribe();
// Using non-prefixed keys
persistStateIntoHyperbee$(hyperbeeInstance, myElfStore, { prefix: null }).subscribe();
// Using a custom CAS function
const customCas = (prev, curr) => prev.version !== curr.version;
persistStateIntoHyperbee$(hyperbeeInstance, myElfStore, { cas: customCas }).subscribe();
// Using a custom distinct function
const customDistinct = ([prev, curr]) => prev.timestamp === curr.timestamp;
persistStateIntoHyperbee$(hyperbeeInstance, myElfStore, { distinct: customDistinct }).subscribe();
loadStateThenPersistStateFromHyperbee$
import { loadStateThenPersistStateFromHyperbee$ } from "elf-hyperbee-persist";
Combines loadStateFromHyperbee$
and persistStateIntoHyperbee$
in sequence.
hyperbee
: The Hyperbee instance.elfStore
: The Elf store to synchronize.options.prefix
(default: "state"
): If provided, applies a prefix to stored and retrieved keys.options.debounce
(default: 1000
): Debounce time before persisting changes.options.cas
(default: a deep equality check): A compare-and-swap (CAS) function that determines whether an entry should be updated in Hyperbee.
prev
(previous entry) and curr
(new entry).true
if the entry should be updated.options.distinct
(default: a deep equality check): A function that determines whether state updates are considered distinct.
distinctUntilChanged
to filter redundant updates.true
if they are the same (i.e., no need to update).An RxJS observable that first loads the state and then starts persisting subsequent changes.
// Using prefixed keys
loadStateThenPersistStateFromHyperbee$(hyperbeeInstance, myElfStore, { prefix: "gameState" }).subscribe();
// Using non-prefixed keys
loadStateThenPersistStateFromHyperbee$(hyperbeeInstance, myElfStore, { prefix: null }).subscribe();
stringStringIndexEncoding
import { stringStringIndexEncoding } from "elf-hyperbee-persist";
A utility that provides encoding for string-based Hyperbee keys.
deepEqual
import { deepEqual } from "elf-hyperbee-persist";
Performs a deep equality check between two objects to detect changes efficiently.
deepEqual({ a: 1, b: 2 }, { a: 1, b: 2 }); // true
deepEqual({ a: 1 }, { a: 1, b: 2 }); // false
pairwiseStartWith
import { pairwiseStartWith } from "elf-hyperbee-persist";
An RxJS operator that emits previous and current values, starting with an initial state.
myObservable.pipe(pairwiseStartWith(initialState));
State Loading:
loadStateFromHyperbee$
reads stored state from Hyperbee.[prefix, key]
structures and direct keys.State Watching & Persistence:
persistStateIntoHyperbee$
listens for changes in the Elf store.deepEqual
to avoid unnecessary writes.prefix
is null
.Full Integration:
loadStateThenPersistStateFromHyperbee$
ensures that state is first loaded before persistence starts.This project is released under the MIT License.
FAQs
RxJS Elf state persistence and synchronization with Hyperbee
The npm package elf-hyperbee-persist receives a total of 182 weekly downloads. As such, elf-hyperbee-persist popularity was classified as not popular.
We found that elf-hyperbee-persist demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.