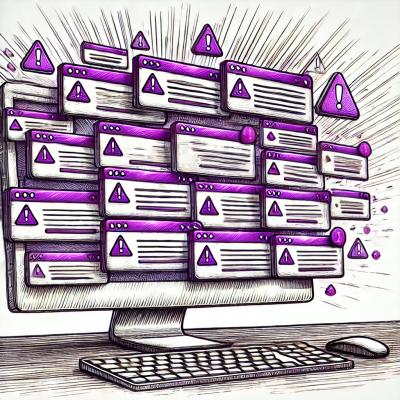
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
email-templates
Advanced tools
Node.js module for rendering beautiful emails with ejs templates and email-friendly inline CSS using juice.
The email-templates npm package is a powerful tool for creating, managing, and sending email templates. It supports various templating engines, inline CSS, and internationalization, making it a versatile choice for handling email communications in Node.js applications.
Template Rendering
This feature allows you to render email templates with dynamic data. In this example, the 'mars/html' template is rendered with the name 'Elon'.
const Email = require('email-templates');
const email = new Email();
email.render('mars/html', { name: 'Elon' })
.then(console.log)
.catch(console.error);
Sending Emails
This feature allows you to send emails using a specified transporter. In this example, an email is sent using Gmail's SMTP service with the 'mars' template and dynamic data.
const Email = require('email-templates');
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your-email@gmail.com',
pass: 'your-email-password'
}
});
const email = new Email({
message: {
from: 'your-email@gmail.com'
},
transport: transporter
});
email.send({
template: 'mars',
message: {
to: 'elon@musk.com'
},
locals: {
name: 'Elon'
}
}).then(console.log).catch(console.error);
Inline CSS
This feature allows you to inline CSS styles in your email templates. In this example, CSS styles are inlined into the 'mars/html' template.
const Email = require('email-templates');
const email = new Email({
juice: true,
juiceResources: {
preserveImportant: true,
webResources: {
relativeTo: path.resolve('path/to/css')
}
}
});
email.render('mars/html', { name: 'Elon' })
.then(console.log)
.catch(console.error);
Internationalization (i18n)
This feature allows you to support multiple languages in your email templates. In this example, the 'mars/html' template is rendered in French.
const Email = require('email-templates');
const email = new Email({
i18n: {
locales: ['en', 'fr'],
directory: path.join(__dirname, 'locales')
}
});
email.render('mars/html', { name: 'Elon', locale: 'fr' })
.then(console.log)
.catch(console.error);
Nodemailer is a module for Node.js applications to allow easy email sending. While it does not provide templating capabilities out of the box, it can be used in conjunction with other templating engines to achieve similar functionality to email-templates.
MJML is a markup language designed to reduce the pain of coding responsive emails. It focuses on the structure and design of emails, and can be used alongside email-templates for rendering MJML templates.
Handlebars is a popular templating engine that can be used to create dynamic email templates. It does not provide email sending capabilities, but can be integrated with Nodemailer or other email sending libraries.
Node.js module for rendering beautiful emails with ejs templates and email-friendly inline CSS using juice.
v0.0.7:
include
directive thanks to @nicjansmav0.0.6:
...has no method slice
) thanks to @vekexasiav0.0.5:
...
template('newsletter', locals, 'deflateRaw', function(err, html, text) {
// The `html` and `text` are buffers compressed using zlib.deflateRaw
// <http://nodejs.org/docs/latest/api/zlib.html#zlib_zlib_deflateraw_buf_callback>
// **NOTE**: You could also pass 'deflate' or 'gzip' if necessary, and it works with batch rendering as well
})
...
v0.0.4 (with bug fix for 0.0.3):
style.css
and text.ejs
files with compatibility in node
v0.6.x to v0.8.x (utilizes path.exists
vs. fs.exists
respectively).For professional and customizable email templates, please visit https://github.com/mailchimp/Email-Blueprints.
npm install email-templates
npm install email-templates-windows
npm install email-templates
.templates
inside your root directory (or elsewhere).templates
folder.include
directive from ejs (for example, to include a common header or footer). See the /examples
folder for details.Want to use different opening and closing tags instead of the EJS's default <%
and %>
?.
...
emailTemplates(templatesDir, { open: '{{', close: '}}' }, function(err, template) {
...
NOTE: You can also pass other options from EJS's documentation.
Render a template for a single email or render multiple (having only loaded the template once).
var path = require('path')
, templatesDir = path.join(__dirname, 'templates')
, emailTemplates = require('email-templates');
emailTemplates(templatesDir, function(err, template) {
// Render a single email with one template
var locals = { pasta: 'Spaghetti' };
template('pasta-dinner', locals, function(err, html, text) {
// ...
});
// Render multiple emails with one template
var locals = [
{ pasta: 'Spaghetti' },
{ pasta: 'Rigatoni' }
];
var Render = function(locals) {
this.locals = locals;
this.send = function(err, html, text) {
// ...
};
this.batch = function(batch) {
batch(this.locals, this.send);
};
};
template('pasta-dinner', true, function(err, batch) {
for(var user in users) {
var render = new Render(users[user]);
render.batch(batch);
}
});
});
var path = require('path')
, templatesDir = path.resolve(__dirname, '..', 'templates')
, emailTemplates = require('email-templates')
, nodemailer = require('nodemailer');
emailTemplates(templatesDir, function(err, template) {
if (err) {
console.log(err);
} else {
// ## Send a single email
// Prepare nodemailer transport object
var transport = nodemailer.createTransport("SMTP", {
service: "Gmail",
auth: {
user: "some-user@gmail.com",
pass: "some-password"
}
});
// An example users object with formatted email function
var locals = {
email: 'mamma.mia@spaghetti.com',
name: {
first: 'Mamma',
last: 'Mia'
}
};
// Send a single email
template('newsletter', locals, function(err, html, text) {
if (err) {
console.log(err);
} else {
transport.sendMail({
from: 'Spicy Meatball <spicy.meatball@spaghetti.com>',
to: locals.email,
subject: 'Mangia gli spaghetti con polpette!',
html: html,
// generateTextFromHTML: true,
text: text
}, function(err, responseStatus) {
if (err) {
console.log(err);
} else {
console.log(responseStatus.message);
}
});
}
});
// ## Send a batch of emails and only load the template once
// Prepare nodemailer transport object
var transportBatch = nodemailer.createTransport("SMTP", {
service: "Gmail",
auth: {
user: "some-user@gmail.com",
pass: "some-password"
}
});
// An example users object
var users = [
{
email: 'pappa.pizza@spaghetti.com',
name: {
first: 'Pappa',
last: 'Pizza'
}
},
{
email: 'mister.geppetto@spaghetti.com',
name: {
first: 'Mister',
last: 'Geppetto'
}
}
];
// Custom function for sending emails outside the loop
//
// NOTE:
// We need to patch postmark.js module to support the API call
// that will let us send a batch of up to 500 messages at once.
// (e.g. <https://github.com/diy/trebuchet/blob/master/lib/index.js#L160>)
var Render = function(locals) {
this.locals = locals;
this.send = function(err, html, text) {
if (err) {
console.log(err);
} else {
transportBatch.sendMail({
from: 'Spicy Meatball <spicy.meatball@spaghetti.com>',
to: locals.email,
subject: 'Mangia gli spaghetti con polpette!',
html: html,
// generateTextFromHTML: true,
text: text
}, function(err, responseStatus) {
if (err) {
console.log(err);
} else {
console.log(responseStatus.message);
}
});
}
};
this.batch = function(batch) {
batch(this.locals, this.send);
};
};
// Load the template and send the emails
template('newsletter', true, function(err, batch) {
for(var user in users) {
var render = new Render(users[user]);
render.batch(batch);
}
});
}
});
NOTE: Did you know nodemailer
can also be used to send SMTP email through Postmark? See this section of their Readme for more info.
For more message format options, see this section of Postmark's developer documentation section.
var path = require('path')
, templatesDir = path.resolve(__dirname, '..', 'templates')
, emailTemplates = require('email-templates')
, postmark = require('postmark')('your-api-key');
emailTemplates(templatesDir, function(err, template) {
if (err) {
console.log(err);
} else {
// ## Send a single email
// An example users object with formatted email function
var locals = {
email: 'mamma.mia@spaghetti.com',
name: {
first: 'Mamma',
last: 'Mia'
}
};
// Send a single email
template('newsletter', locals, function(err, html, text) {
if (err) {
console.log(err);
} else {
postmark.send({
From: 'Spicy Meatball <spicy.meatball@spaghetti.com>',
To: locals.email,
Subject: 'Mangia gli spaghetti con polpette!',
HtmlBody: html,
TextBody: text
}, function(err, response) {
if (err) {
console.log(err.status);
console.log(err.message);
} else {
console.log(response);
}
});
}
});
// ## Send a batch of emails and only load the template once
// An example users object
var users = [
{
email: 'pappa.pizza@spaghetti.com',
name: {
first: 'Pappa',
last: 'Pizza'
}
},
{
email: 'mister.geppetto@spaghetti.com',
name: {
first: 'Mister',
last: 'Geppetto'
}
}
];
// Custom function for sending emails outside the loop
//
// NOTE:
// We need to patch postmark.js module to support the API call
// that will let us send a batch of up to 500 messages at once.
// (e.g. <https://github.com/diy/trebuchet/blob/master/lib/index.js#L160>)
var Render = function(locals) {
this.locals = locals;
this.send = function(err, html, text) {
if (err) {
console.log(err);
} else {
postmark.send({
From: 'Spicy Meatball <spicy.meatball@spaghetti.com>',
To: locals.email,
Subject: 'Mangia gli spaghetti con polpette!',
HtmlBody: html,
TextBody: text
}, function(err, response) {
if (err) {
console.log(err.status);
console.log(err.message);
} else {
console.log(response);
}
});
}
};
this.batch = function(batch) {
batch(this.locals, this.send);
};
};
// Load the template and send the emails
template('newsletter', true, function(err, batch) {
for(user in users) {
var render = new Render(users[user]);
render.batch(batch);
}
});
}
});
MIT Licensed
FAQs
Create, preview (browser/iOS Simulator), and send custom email templates for Node.js. Made for Forward Email and Lad.
The npm package email-templates receives a total of 93,724 weekly downloads. As such, email-templates popularity was classified as popular.
We found that email-templates demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.