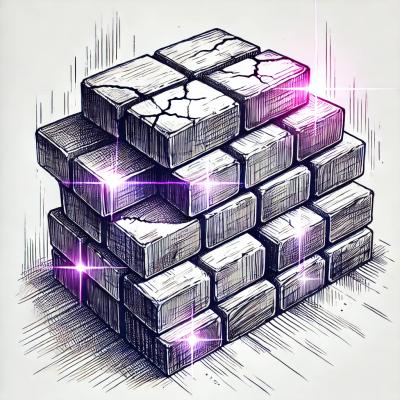
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
ember-keyboard
Advanced tools
ember-keyboard
, an Ember addon for the painless support of keyboard events.
ember-key-responder
for the inspiration)ember-keyboard-service
for the inspiration)keyup
and keydown
, as well as the modifier keys: ctrl
, alt
, shift
, and meta
.ember install ember-keyboard
First, inject ember-keyboard
into your component:
export default Ember.Component.extend({
keyboard: Ember.inject.service()
});
Once the keyboard
service is injected, you need to activate it.
activateKeyboard: Ember.on('didInsertElement', function() {
this.get('keyboard').activate(this);
})
This will place the component in the eventStack
, meaning it'll be able to respond to key events. Let's say you want your component to respond to the key a
as well as ctrl+shift+a
. You could do so with:
import { keyUp } from 'ember-keyboard';
. . . .
aFunction: Ember.on(keyUp('a'), function() {
console.log('`a` was pressed');
}),
anotherFunction: Ember.on(keyUp('ctrl+shift+a'), function() {
console.log('`ctrl+shift+a` was pressed');
})
The modifier keys include ctrl
, shift
, alt
, and meta
. For a full list of the primary keys (such as a
, 1
,
, Escape
, and ArrowLeft
), look here.
Finally, when you're ready for a component to leave the eventStack
you can deactivate it:
deactivateKeyboard: Ember.on('arbitraryTrigger', function() {
this.get('keyboard').deactivate(this);
})
Note that components will automatically be deactivated on willDestroyElement
.
keyboardPriority
By default, all activated components are treated as equal. If you have two components that respond to ctrl+a
, then both will get triggered when there's a ctrl+a
event. However, this behavior is undesirable in some scenarios. What if you have a modal open, and you only want it and its child components to respond to key events. You can get this behavior by assigning a priority to the modal and its children:
noPriorityComponent; // priority defaults to 0
lowPriorityComponent.set('keyboardPriority', 0);
modal.set('keyboardPriority', 1);
modalChild.set('keyboardPriority', 1);
In this scenario, when a key is pressed both modal
and modalChild
will have a chance to respond to it, while the remaining components will not. Once modal
and modalChild
are deactivated or their priority is removed, then lowPriorityComponent
and noPriorityComponent
will respond to key events.
Perhaps more conveniently, this property can be passed in through your template:
{{my-component keyboardPriority=1}}
{{my-dynamic-component keyboardPriority=dynamicPriority}}
Note that priority is descending, so higher numbers have precedence.
To reduce boilerplate, ember-keyboard
includes several mixins with common patterns.
import { EKOnInsertMixin } from 'ember-keyboard';
This mixin will activate the component on didInsertElement
, and as per normal, it will deactivate on willDestroyElement
.
import { EKOnFocusMixin } from 'ember-keyboard';
This mixin will activate the component whenever it receives focus and deactivate it when it loses focus.
Note that to ensure that the component is focusable, this mixin sets the component's tabindex
to 0.
import { EKFirstResponderMixin } from 'ember-keyboard';
This mixin does not activate or deactivate the component. Instead, it allows you to make a component the first and only responder, regardless of its initial keyboardPriority
. This can be useful if you want a low-priority component to temporarily gain precedence over everything else. When it resigns its first responder status, it automatically returns to its previous priority. Note that if you assign a second component first responder status, the first one will in turn lose first responder status.
To make this possible, this mixin adds two functions to the component:
// from within the component
this.becomeFirstResponder();
Makes the component the first responder. It will be activated (ember-keyboard.activate()
) if it has not yet been.
Note: This is accomplished by assigning the component a ridiculously high keyboardPriority
(9999999999999). If you manually change its priority after it becomes first responder, it will lose first responder status.
// from within the component
this.resignFirstResponder();
Resigns first responder status, in the process returning to its previous priority.
import { EKFirstResponderOnFocusMixin } from 'ember-keyboard';
This mixin grants the component first responder status while it is focused. When it loses focus, it resigns its status.
Note that to ensure that the component is focusable, this mixin sets the component's tabindex
to 0.
Ember.TextField
&& Ember.TextArea
To prevent ember-keyboard
from responding to key strokes while an input/textarea is focused, we've reopened Ember.TextField
and Ember.TextArea
and applied the EKOnInsertMixin
and EKFirstResponderOnFocusMixin
. This ensures that whenever an input is focused, other key responders will not fire. If you want to have responders associated with an input or textarea (such as a rich text editor with keyUp('ctrl+i')
bindings), you need to extend these components from Ember.TextField
or Ember.TextArea
rather than Ember.component
.
This applies to input
and textarea
helpers:
{{input}}
{{textarea}}
keyUp
and keyDown
ember-keyboard
listens to both keydown
and keyup
events, and has corresponding functions:
import { keyUp, keyDown } from 'ember-keyboard';
Note that keydown
events fire repeatedly while the key is pressed, while keyup
events fire only once, after the key has been released.
event
When ember-keyboard
triggers an event, it passes in the event
object as its first argument:
saveDocument: Ember.on(keyDown('ctrl+s'), function(event) {
this.performSave();
event.preventDefault();
})
Note that if you want preventDefault
to prevent window
level events, you'll need to use keyDown
, as the default event will fire before keyUp
.
getKey
Did you know that 65 was the keycode for 'a'? Or that 37 was the keycode for the right arrow? If you don't want to litter your code with keycode references, you can use getKey
, which ember-keyboard
uses internally:
import { getKey } from 'ember-keyboard';
. . . .
keyMapper: Ember.on(keyDown('a'), keyDown('b'), keyDown('c'), function(event) {
const key = getKey(event);
switch (key) {
match 'a': console.log('It\'s an a!'); break;
. . . .
}
})
Just pass in an event
, and it'll return a human readable key. Look here for a full mapping.
If you want an event to fire for every keypress, then simply don't provide a keystring to keyUp
or keyDown
. This can be a handy way to trigger events for large ranges of keys, such as on any alphanumeric keypress. For instance:
triggerOnAlphaNumeric: Ember.on(keyUp(), function(event) {
if (/^\w(?!.)/.test(getKey(event.keyCode)) {
this.startEditing();
}
})
meta
and alt
Macs diverge from other PCs in their naming of meta
and alt
. The alt
key is named options
, while the meta
key is named command
. You don't need to do anything special to get ember-keyboard
to recognize options
or command
keys, just use their generic names alt
and meta
. This will ensure that your keys fire across platforms.
If you'd like to dynamically add and remove key listeners on a component, you can do so with the standard on
and off
functions:
component.on(keyUp('ctrl+s'), someFunction);
component.off(keyUp('ctrl+s'), someFunction);
FAQs
An Ember.js addon for the painless support of keyboard events
The npm package ember-keyboard receives a total of 17,814 weekly downloads. As such, ember-keyboard popularity was classified as popular.
We found that ember-keyboard demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.