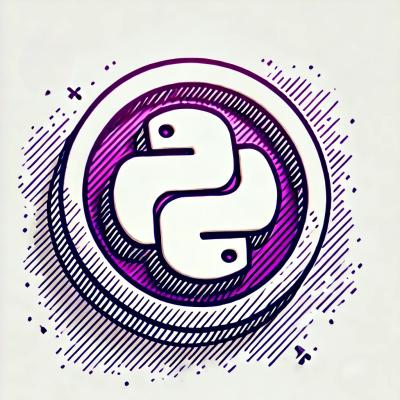
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
encoding-down
Advanced tools
The encoding-down npm package is a codec layer for levelup that provides encoding and decoding of keys and values. It allows you to use different encodings for keys and values, making it easier to work with various data types in a LevelDB store.
Basic Usage
This code demonstrates how to set up a LevelDB store with JSON encoding for values using encoding-down. It shows how to put and get a JSON object.
const levelup = require('levelup');
const leveldown = require('leveldown');
const encode = require('encoding-down');
const db = levelup(encode(leveldown('./mydb'), { valueEncoding: 'json' }));
db.put('key', { some: 'value' }, function (err) {
if (err) return console.log('Ooops!', err);
db.get('key', function (err, value) {
if (err) return console.log('Ooops!', err);
console.log('Got value:', value);
});
});
Custom Encoding
This code demonstrates how to use a custom encoding for values in a LevelDB store. The custom encoding converts values to and from Buffers.
const levelup = require('levelup');
const leveldown = require('leveldown');
const encode = require('encoding-down');
const customEncoding = {
encode: (val) => Buffer.from(val.toString()),
decode: (val) => val.toString(),
buffer: true,
type: 'custom'
};
const db = levelup(encode(leveldown('./mydb'), { valueEncoding: customEncoding }));
db.put('key', 'custom value', function (err) {
if (err) return console.log('Ooops!', err);
db.get('key', function (err, value) {
if (err) return console.log('Ooops!', err);
console.log('Got value:', value);
});
});
Binary Encoding
This code demonstrates how to use binary encoding for values in a LevelDB store. It shows how to put and get a binary value.
const levelup = require('levelup');
const leveldown = require('leveldown');
const encode = require('encoding-down');
const db = levelup(encode(leveldown('./mydb'), { valueEncoding: 'binary' }));
db.put('key', Buffer.from('binary value'), function (err) {
if (err) return console.log('Ooops!', err);
db.get('key', function (err, value) {
if (err) return console.log('Ooops!', err);
console.log('Got value:', value.toString());
});
});
The level-codec package provides encoding and decoding functionality for LevelDB. It allows you to define custom encodings for keys and values, similar to encoding-down. However, encoding-down is more integrated with the level ecosystem and provides a more streamlined API for setting up encodings.
The level-transcoder package offers a way to transform data as it is read from or written to a LevelDB store. It supports various encoding and decoding schemes, similar to encoding-down. However, encoding-down is more focused on providing a codec layer specifically for levelup, making it easier to use in that context.
WIP LevelDOWN wrapper supporting levelup@1 encodings. For motivation, see this issue.
TODO
MIT
[2.0.8] - 2017-01-26
Iterator.prototype.seek
(@juliangruber)FAQs
An abstract-leveldown implementation that wraps another store to encode keys and values
The npm package encoding-down receives a total of 111,578 weekly downloads. As such, encoding-down popularity was classified as popular.
We found that encoding-down demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.