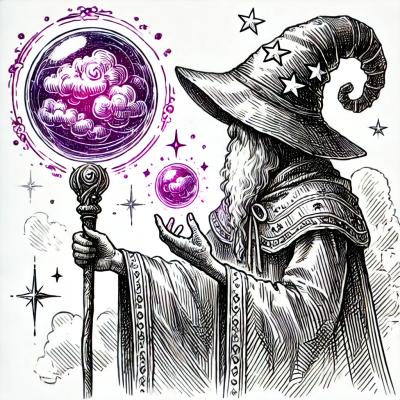
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
encrypt-uint8array
Advanced tools
Encrypt and decrypt an `Uint8Array` using another `Uint8Array` as password
Encrypt and decrypt an
Uint8Array
using anotherUint8Array
as password
$ npm install encrypt-uint8array
const {
encryptUint8Array,
decryptUint8Array,
encodeString, // For convenience, but can be done with native TextEncoder
decodeString // For convenience, but can be done with native TextDecoder
} = require('encrypt-uint8array');
(async () => {
const secret = encodeString('This is the secret');
const password = encodeString('P4s$w0Rd!');
const encrypted = await encryptUint8Array(secret, password);
console.log(encrypted);
//=> Uint8Array [ 0, 1, 1, 65, /* ... */, 103, 26 ]
const decrypted = await decryptUint8Array(encrypted, password);
console.log(decrypted);
//=> Uint8Array [ 84, 104, 105, 115, /* ... */, 101, 116 ]
console.log(decodeString(decrypted));
//=> 'This is the secret'
await decryptUint8Array(encrypted, encodeString('wrong password'));
//=> DecryptionError: Unable to decrypt - password is incorrect or data is corrupted.
})();
Async function that encrypts data
with password
. Returns a new Uint8Array
.
Type: Uint8Array
The data to be encrypted.
Type: Uint8Array
The Uint8Array
to be used as password.
Async function that decrypts encryptedData
with password
. Returns a new Uint8Array
.
If encryptedData
is not valid or the password is incorrect, this function will throw a DecryptionError
.
Type: Uint8Array
The data to be decrypted.
Type: Uint8Array
The Uint8Array
to be used as password.
FAQs
Encrypt and decrypt an `Uint8Array` using another `Uint8Array` as password
The npm package encrypt-uint8array receives a total of 47 weekly downloads. As such, encrypt-uint8array popularity was classified as not popular.
We found that encrypt-uint8array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.