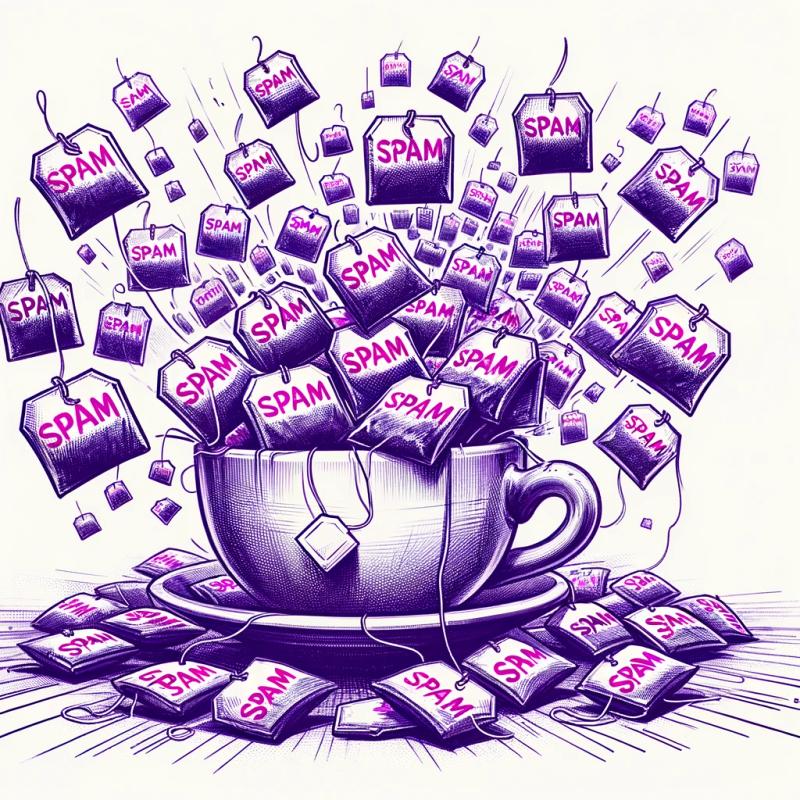
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
encrypted-env
Advanced tools
Readme
encrypted-env
is designed to make encrypting and decrypting sensitive .env
files a breeze. It's supports using different configuration files for different environments (e.g. development
, staging
, and production
) with different encryption keys for each, and can detect which to load based on existing environmental variavbles.
npm install encrypted-env
This package provides two commands, env-encrypt
and env-decrypt
. To encrypt your .env
file, run npx env-encrypt
. If encrypting for the first time, the user will be prompted to enter in a 32-character key. The key is then stored for future encryption / decryption.
Create a .env-encrypted.config.json
configuration file in the root of your project that maps environment names to the filename of the configuration file to use.
{
"development": ".env.dev",
"staging": ".env.staging",
"production": ".env.prod"
}
Once defined, you can encrypt and decrypt configuration files for each environment by appending the environment name to the command:
npx env-encrypt staging
This may also be used in the scripts
section of your package.json
file:
{
"name": "project-name",
"scripts": {
"encrypt:staging": "env-encrypt staging",
"decrypt:staging": "env-decrypt staging"
}
}
To enable dynamic decryption in CI, set a secret in the repository and inject it into the process ENV for decryption.
For example, here's a GitHub Actions job that decrypts the env files for a project prior to running integration tests that require them:
integration-test:
runs-on: ubuntu-latest
timeout-minutes: 5
steps:
- name: Checkout
uses: actions/checkout@v2
- name: Setup Node
uses: actions/setup-node@v2
with:
node-version: 14.x
- name: Install dependencies
run: npm install
- name: Decrypt env
run: npx env-decrypt
env:
ENV_KEY: ${{ secrets.ENV_KEY }}
CI: true
- name: Run integration tests
run: npm run test:integration
To load environmental variables into your project:
import loadENV from 'encrypted-env';
const env = loadENV();
If you have multiple environments defined, it will load the config file that corresponds to the environment set in the NODE_ENV
or ENVIRONMENT
variable.
For example, if the NODE_ENV
process env is set to development
and the .env-encrypted.config.json
file looks like this:
{
"development": ".env.dev",
"staging": ".env.staging",
"production": ".env.prod"
}
Then it will attempt to parse and load .env.dev
. If it cannot find .env.dev
, it will fail with an error prompting the user to run npx env-decrypt development
- which will attempt to create .env.dev
from .env.dev.encrypted
.
Make sure to add these lines to your .gitignore
file:
**/*.env
**/*.key
This way, only the encrypted .env
files will be pushed to version control.
MIT © Jesse Youngblood
FAQs
Encrypt and decrypt env config files quickly and easily
The npm package encrypted-env receives a total of 51 weekly downloads. As such, encrypted-env popularity was classified as not popular.
We found that encrypted-env demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.