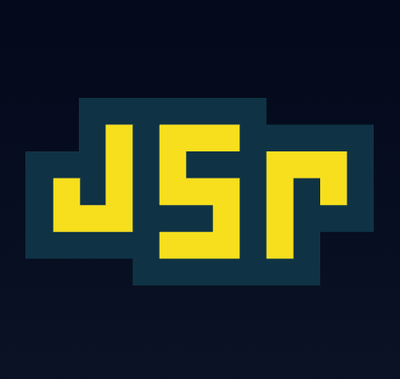
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
engine.io-parser
Advanced tools
The engine.io-parser npm package is designed for encoding and decoding packets used by engine.io, which is a transport layer for real-time data exchange between a client and a server. It handles the framing of messages for transport over various real-time protocols and supports binary data.
Encoding messages
This feature allows you to encode a message packet into a format suitable for transport over the network. The `encodePacket` function takes a packet object and a callback function that receives the encoded packet.
const { encodePacket } = require('engine.io-parser');
const packet = { type: 'message', data: 'hello' };
encodePacket(packet, false, (encodedPacket) => {
// handle the encoded packet
});
Decoding messages
This feature allows you to decode a message packet that was received from the network. The `decodePacket` function takes an encoded packet and the type of data ('string' or 'binary') and returns the decoded packet object.
const { decodePacket } = require('engine.io-parser');
const encodedPacket = '2hello'; // example of an encoded message packet
const packet = decodePacket(encodedPacket, 'string');
// handle the decoded packet
Support for binary data
This feature provides support for encoding and decoding binary data. The `encodePacket` and `decodePacket` functions can handle binary data when the second argument is set to `true` for binary.
const { encodePacket, decodePacket } = require('engine.io-parser');
const binaryData = new ArrayBuffer(100);
const packet = { type: 'message', data: binaryData };
encodePacket(packet, true, (encodedPacket) => {
// handle the encoded packet with binary data
});
const decodedPacket = decodePacket(encodedPacket, 'binary');
// handle the decoded packet with binary data
The 'ws' package provides a WebSocket client and server implementation. It allows for real-time communication similar to engine.io-parser but is focused on the WebSocket protocol specifically, whereas engine.io-parser is part of the engine.io ecosystem which can fall back to other transport mechanisms if WebSockets are not available.
The 'socket.io-parser' is used by Socket.IO to encode and decode messages. It is similar to engine.io-parser but is tailored for the Socket.IO framework, which builds on top of engine.io to provide additional features like rooms, namespaces, and middleware support.
The 'json-socket' package is a wrapper around the Node.js net library that adds a layer for seamless JSON message sending. It is similar to engine.io-parser in that it handles message framing, but it is specifically designed for TCP sockets and does not support the variety of transport layers that engine.io-parser does.
This is the JavaScript parser for the engine.io protocol encoding, shared by both engine.io-client and engine.io.
The parser can encode/decode packets, payloads, and payloads as binary
with the following methods: encodePacket
, decodePacket
, encodePayload
,
decodePayload
.
Example:
const parser = require("engine.io-parser");
const data = Buffer.from([ 1, 2, 3, 4 ]);
parser.encodePacket({ type: "message", data }, encoded => {
const decodedData = parser.decodePacket(encoded); // decodedData === data
});
Engine.IO Parser is a commonjs module, which means you can include it by using
require
on the browser and package using browserify:
install the parser package
npm install engine.io-parser
write your app code
const parser = require("engine.io-parser");
const testBuffer = new Int8Array(10);
for (let i = 0; i < testBuffer.length; i++) testBuffer[i] = i;
const packets = [{ type: "message", data: testBuffer.buffer }, { type: "message", data: "hello" }];
parser.encodePayload(packets, encoded => {
parser.decodePayload(encoded,
(packet, index, total) => {
const isLast = index + 1 == total;
if (!isLast) {
const buffer = new Int8Array(packet.data); // testBuffer
} else {
const message = packet.data; // "hello"
}
});
});
build your app bundle
$ browserify app.js > bundle.js
include on your page
<script src="/path/to/bundle.js"></script>
Note: cb(type)
means the type is a callback function that contains a parameter of type type
when called.
encodePacket
Object
: the packet to encode, has type
and data
.
data
: can be a String
, Number
, Buffer
, ArrayBuffer
Boolean
: binary supportFunction
: callback, returns the encoded packet (cb(String)
)decodePacket
String
or (Blob
on browser, ArrayBuffer
on Node)String
| ArrayBuffer
: the packet to decode, has type
and data
String
: optional, the binary typeencodePayload
Array
: an array of packetsFunction
: callback, returns the encoded payload (cb(String)
)decodePayload
String
: the payloadFunction
: callback, returns (cb(Object
: packet, Number
:packet index, Number
:packet total))Standalone tests can be run with npm test
which will run the node.js tests.
Browser tests are run using zuul. (You must have zuul setup with a saucelabs account.)
You can run the tests locally using the following command:
npm run test:browser
The support channels for engine.io-parser
are the same as socket.io
:
To contribute patches, run tests or benchmarks, make sure to clone the repository:
git clone git://github.com/socketio/engine.io-parser.git
Then:
cd engine.io-parser
npm ci
See the Tests
section above for how to run tests before submitting any patches.
MIT
FAQs
Parser for the client for the realtime Engine
The npm package engine.io-parser receives a total of 5,868,029 weekly downloads. As such, engine.io-parser popularity was classified as popular.
We found that engine.io-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.