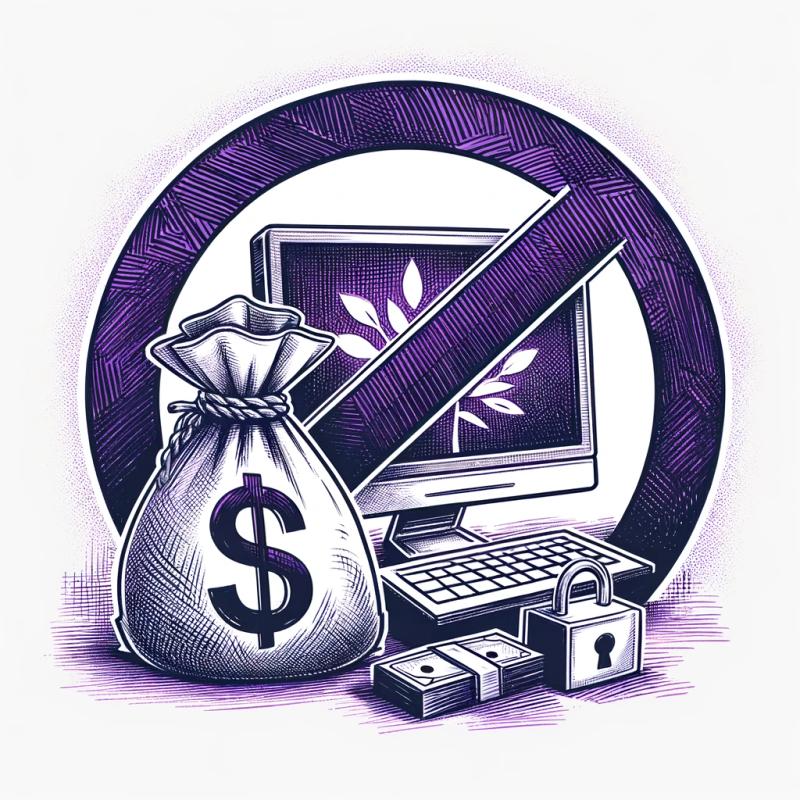
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
enquirer
Advanced tools
Package description
The enquirer npm package is a stylish, intuitive, and user-friendly way to interact with users via the command line. It provides a rich set of prompt types and utilities to make CLI development easier and more interactive.
Text Input
This feature allows you to ask users for text input. The example shows how to ask for a username.
const { prompt } = require('enquirer');
prompt({
type: 'input',
name: 'username',
message: 'What is your username?'
}).then(answer => console.log('Username:', answer.username));
Password Input
This feature enables you to securely ask users for a password, hiding their input on the screen.
const { prompt } = require('enquirer');
prompt({
type: 'password',
name: 'password',
message: 'What is your password?'
}).then(answer => console.log('Password:', answer.password));
Multiple Choice (Select)
This feature allows users to choose from a list of options. The example demonstrates asking users to pick a color from a list.
const { prompt } = require('enquirer');
prompt({
type: 'select',
name: 'color',
message: 'Pick a color',
choices: ['Red', 'Green', 'Blue']
}).then(answer => console.log('Selected color:', answer.color));
Checkbox
This feature lets users select multiple options from a list. The example asks users to select their favorite fruits.
const { prompt } = require('enquirer');
prompt({
type: 'multiselect',
name: 'fruits',
message: 'What are your favorite fruits?',
choices: ['Apple', 'Orange', 'Grape', 'Watermelon']
}).then(answer => console.log('Favorite fruits:', answer.fruits));
Inquirer.js is a common alternative to Enquirer with a similar feature set for creating interactive CLI prompts. It's known for its wide adoption and extensive plugin system but is generally considered to be heavier than Enquirer.
Prompts is a lightweight, minimal alternative that focuses on simplicity and performance. While it offers a similar range of prompt types to Enquirer, it might not be as feature-rich or extensible.
Readme
Intuitive, plugin-based prompt system for node.js. Much faster and lighter alternative to Inquirer, with all the same prompt types and more, but without the bloat.
(TOC generated by verb using markdown-toc)
Install with npm:
$ npm install --save enquirer
Create an instance of Enquirer
with the given options
.
Params
options
{Object}Example
var Enquirer = require('enquirer');
var enquirer = new Enquirer();
Register a new prompt type
with the given fn
.
Params
type
{String}: The name of the prompt typefn
{Function}: Prompt function that inherits from enquirer-prompt.returns
{Object}: Returns the Enquirer instance for chaining.Example
enquirer.register('confirm', require('enquirer-prompt-confirm'));
Invoke a plugin fn
Params
fn
{Function}: Function that takes an instance of Enquirer
returns
{Object}: Returns the instance for chaining.Example
enquirer.use(require('some-enquirer-plugin'));
Create question name
with the given message
and options
. Uses enquirer-question, visit that library for additional details.
Params
name
{String|Object}: Name or options objectmessage
{String|Object}: Message or options objectoptions
{Object}returns
{Object}: Returns the created question objectEvents
emits
: question
Example
enquirer.question('color', 'What is your favorite color?');
enquirer.question('color', 'What is your favorite color?', {
default: 'blue'
});
enquirer.question('color', {
message: 'What is your favorite color?',
default: 'blue'
});
enquirer.question({
name: 'color',
message: 'What is your favorite color?',
default: 'blue'
});
enquirer.question({
name: 'color',
type: 'input', // "input" is the default prompt type and doesn't need to be specified
message: 'What is your favorite color?',
default: 'blue'
});
Initialize a prompt session for one or more questions.
returns
{Array|Object} questions
: One or more question objects or names of registered questions.Events
emits
: ask
With the array of questions
to be askedExample
var Enquirer = require('enquirer');
var enquirer = new Enquirer();
enquirer.question('first', 'First name?');
enquirer.question('last', 'Last name?');
enquirer.ask('first')
.then(function(answers) {
console.log(answers)
});
// errors
enquirer.ask('first')
.then(function(answers) {
console.log(answers)
})
.catch(function(err) {
console.log(err)
});
Initialize a prompt session for a single question. Used by the ask method.
Params
name
{String}Events
emits
: prompt
with the default
value, key
, question
object, and answers
objectemits
: answer
with the answer
value, key
, question
object, and answers
objectExample
var Enquirer = require('enquirer');
var enquirer = new Enquirer();
enquirer.question('first', 'First name?');
enquirer.prompt('first')
.then(function(answers) {
console.log(answers)
});
Create a new Separator
to use in a choices array.
Create a new Separator
to use in a choices array.
What is a prompt "type"?
Prompt types determine the type of question, or prompt, to initiate. Currently, the only prompt type included in enquirer is input
.
The following types are all available as plugins (note that all of these modules are finished, I'm pushing them up one-by-one, and will check them off as I go):
checkbox
(enquirer-prompt-checkbox)confirm
(enquirer-prompt-confirm)editor
([enquirer-prompt-editor][])expand
([enquirer-prompt-expand][])input
(enquirer-prompt-input) (included in enquirer by default)list
([enquirer-prompt-list][])password
([enquirer-prompt-password][])radio
(enquirer-prompt-radio)rawlist
([enquirer-prompt-rawlist][])Or you can use [enquirer-prompts][], if you want a bundle with all of the listed prompt types.
Prompt modules are named using the convention enquirer-prompt-*
.
TBC
TODO
Plugin modules are named using the convention enquirer-*
.
TBC
filter
, when
etcWe use prompts extensively in our projects, and we wanted to improve the user experience and reduce dependencies associated with other libraries we tried, like Inquirer.
Our main goals were:
Initial load time
Enquirer takes ~11ms to load. This is about the same amount of time that it takes chalk to load.
By comparison, Inquirer takes ~120ms just to load!!! This is about how long it takes babel, or other massive libraries that you would never include in production code.
Regardless of whether or not a prompt is every actually used, your own application will be 120ms slower from having Inquirer in its dependency tree. This is caused by its own massive dependency tree, code redundancy, monolithic and slow reactive interface (which makes little sense for this use case anyway), poor API design (Inquirer actually executes code, even if you never call the library!), and so on.
120ms might not seem like a lot, but there is a critical threshold where performance of an application begins to feel laggy, and having inquirer in your dependency tree cuts into that threshold significantly, leaving less room for everything else.
Make prompts easier to add
Inquirer uses a reactive interface for flow control. Aside from being overkill and not offering and real code advantages, to work with the code you need to be familiar with microsoft's RX first. This makes it a pain to add new prompt types (e.g. you probably won't).
Regarding the specific "merits" of RX alone, we think it's overkill, makes the application slow, bloated, hard to maintain, hard to contribute to, and difficult to extend. Events are sufficient.
Code footprint
By moving prompt types into separate libraries, we're able to keep the core library small and fast. Moreover, implementors and authors can create their own prompt types without having to require enquirer itself (unlike inquirer). This also makes the individual prompt libraries easier to maintain.
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Please read the contributing guide for avice on opening issues, pull requests, and coding standards.
(This document was generated by verb-generate-readme (a verb generator), please don't edit the readme directly. Any changes to the readme must be made in .verb.md.)
To generate the readme and API documentation with verb:
$ npm install -g verb verb-generate-readme && verb
Install dev dependencies:
$ npm install -d && npm test
Jon Schlinkert
Copyright © 2016, Jon Schlinkert. Released under the MIT license.
This file was generated by verb-generate-readme, v0.1.30, on August 30, 2016.
FAQs
Stylish, intuitive and user-friendly prompt system. Fast and lightweight enough for small projects, powerful and extensible enough for the most advanced use cases.
We found that enquirer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).