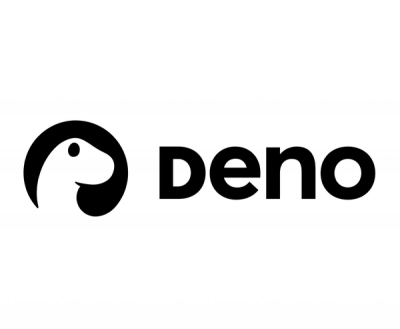
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
enum-class.js
Advanced tools
enum-class.js
gives JavaScript the power of strongly-typed enums, inspired by modern C++ enum class
es, Python namedtuples
and Java enum
s, with dynamic JavaScript sugar sprinkled on top.
Similar to the flexibility of the namedtuple
constructor in Python, you can pass the members as ['A', 'B', 'C']
, 'A, B, C'
or 'A B C'
:
const Color = EnumClass('Color', 'Red, Green, Blue');
const Food = EnumClass('Food', 'Ham Spam');
But also optionally associate values with the enum members:
const Favorites = EnumClass('Food', {
Red: function() { console.log('Every member has a value!'); },
Spaghetti: [1, 2, 3],
Google: "I'm immutable after configuration."
});
The special thing is that members of enum classes are ... instances of themselves :scream:
console.log(Color.Red instanceof Color); // true
As such, we get maximum type safety:
console.log(Color.Red === Favorites.Red); // false
But also all the good reflection stuff:
for (let member of Color) {
console.log(member.name); // Red, Green, Blue
console.log(member.toString()); // Red, Green, Blue
console.log(member.value); // undefined, because we didn't pass an object
}
And extensibility:
Color.add('Orange', 'value');
console.log(Color.length === 4); // true
console.log(Color.contains('Orange')); // true
console.log(Color.isMember(Color.Red)); // true
console.log(Color.isMember(Favorites.Google)); // false
$ npm install enum-class.js
Tests with ava, docs with ESDoc, transpilation with Babel and compilation/compression with Closure. Easy life, smiles and champagne with:
$ gulp
The source is small and modern, so feel free to hack around.
This project is released under the MIT License. For more information, see the LICENSE file.
This is my first ever JavaScript/Node project, so I have absolutely no idea what the hell I'm doing.
Peter Goldsborough + cat :heart:
FAQs
Strongly typed enums in JavaScript
The npm package enum-class.js receives a total of 1 weekly downloads. As such, enum-class.js popularity was classified as not popular.
We found that enum-class.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.