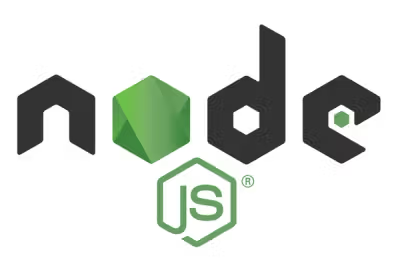
Security News
Node.js TSC Votes to Stop Distributing Corepack
Corepack will be phased out from future Node.js releases following a TSC vote.
enum-i18n
extends the excellent enum implementation adrai/enum. It exposes an Enum constructor that can be configured with a translate
function.
npm install -S enum-props
This document addresses the i18n features added here. For full documentation see adrai/enum.
The default export of this module is a factory that returns partially configured Enum
constructors. You can pass any config values supported by adrai/enum and they will be used for any enumerations instantiated with the returned constructor.
var enumI18n = require( "enum-i18n" );
var FrozenEnum = enumI18n( { freez: true } ); // Constructor for readonly enums
var InsensitiveEnum = enumI18n( { ignoreCase: true } ); // Constructor for case insensitive enums
Every enumeration must be provided a translate function. This function will be called to get a plain-text description of each enum member. A translate function will be passed an enum member and is expected to return a string.
You can pass a translate function to the constructor factory:
var Enum = require( "enum-i18n" )( {
translate: function( member ) {
return getTranslation( "enums." + member.enum.name + "." + member.key );
}
} );
// Same as this shorthand:
var Enum = require( "enum-i18n" )( function( member ) {
return getTranslation( "enums." + member.enum.name + "." + member.key );
} );
Or you can pass a translate function to the constructor:
var Enum = require( "enum-i18n" )();
var colors = new Enum( [ "red", "yellow", "green" ], {
name: "colors",
translate: function( member ) {
return member.key;
}
} );
If you pass both then the constructor's translate function will win.
Every enumeration must be provided with a name
and a translate
function. The name
must be provided at the time the enumeration is instantiated.
var Enum = require( "enum-i18n" )( translator );
var colors = Enum( [ "red", "yellow", "green" ], {
name: "colors"
} );
// Same as shorthand:
var colors = Enum( [ "red", "yellow", "green" ], "colors" );
You can also pass any options supported by adrai/enum.
If you have a single translation collection for your app, you can pass a translate function in the enum-i18n
options:
// Pass config and get back an Enum constructor
var Enum = require( "enum-i18n" )( function( member ) {
// Return a string to use as the enum member's description
return getTranslation( "enums." + member.enum.name + "." + member.key );
);
// Define a simple enum
var colors = new Enum( [ "red", "yellow", "green" ], "colors" );
// Render the member keys/values/descriptions
colors.enums.map( function( member ) {
return member.key + ", " + member.value + ", " + member.toDescription();
} );
/*
[
"red, 1, enums.colors.red",
"yellow, 2, enums.colors.yellow",
"green, 4, enums.colors.green"
]
*/
If you have a translation collection per enumeration, you can pass a translate function in the constructor options:
// Pass config and get back an Enum constructor
var Enum = require( "enum-i18n" )();
var COLOR_NAMES = {
red: "stop",
yellow: "caution",
green: "go"
};
// Define a simple enum
var colors = new Enum( [ "red", "yellow", "green" ], {
name: "colors",
translate: function( member ) {
// Return a string to use as the enum member's description
return COLOR_NAMES[ member.key ];
}
} );
// Render the member keys/values/descriptions
colors.enums.map( function( member ) {
return member.key + ", " + member.value + ", " + member.toDescription();
} );
/*
[
"red, 1, stop",
"yellow, 2, caution",
"green, 4, go"
]
*/
npm test # run tests
gulp coverage # run tests with linting and coverage
gulp show-coverage # show coverage full report
gulp # build compiled lib
FAQs
Enum with support for translated member descriptions
The npm package enum-i18n receives a total of 263 weekly downloads. As such, enum-i18n popularity was classified as not popular.
We found that enum-i18n demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.
Research
Security News
Research uncovers Black Basta's plans to exploit package registries for ransomware delivery alongside evidence of similar attacks already targeting open source ecosystems.
Security News
Oxlint's beta release introduces 500+ built-in linting rules while delivering twice the speed of previous versions, with future support planned for custom plugins and improved IDE integration.