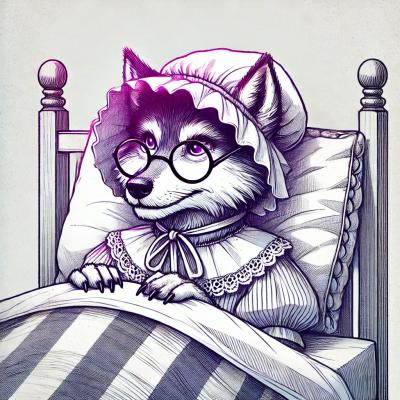
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Enhanced sequencing of asynchronous code with ES6 generators.
A tiny module created to solve one problem in a generalized way.
Write concise tests for an asynchronous story. Skip execution of side-effects. Instead, assert their call arguments, and mock their plain return value. See: "Easily test yielded calls, mock their return values"
Inspired by the test-friendly generator architecture of redux-saga
and the pure side-effects of Elm.
ES6/Generator support.
npm install episode-7 --save
See: CHANGELOG
const Episode7 = require('episode-7');
const fetch = require('node-fetch');
// Barebones helper to fetch & decode JSON.
function fetchJson(url) {
return fetch(url).then( response => response.json() );
}
// Compose a Generator for an async call sequence.
function* findFirstMovie(searchTerm) {
// Wrap side-effects with Episode 7's `call`
let results = yield Episode7.call(
fetchJson, `http://www.omdbapi.com/?s=${searchTerm}`
);
let movie = yield Episode7.call(
fetchJson, `http://www.omdbapi.com/?i=${results.Search[0].imdbID}`
);
return Promise.resolve(movie);
}
// Run the generator & get a Promise for the completed process.
Episode7.run(findFirstMovie, 'Episode 7')
.then( movie => console.log(movie.Title) )
How many times can a person write web service API calls in a dependent sequence, e.g. authenticate, fetch identity, query for records, then create or update those records? So many variations of this theme are created everyday, frequently involving multiple services.
I created this module to make it easy to code sequences of web service API requests interleaved with programmatic logic. I'm pulling the essence of redux-saga
into the smallest, plainest surface area conceivable.
FAQs
Sequence async side-effects with ES6 generators and easily test them.
The npm package episode-7 receives a total of 0 weekly downloads. As such, episode-7 popularity was classified as not popular.
We found that episode-7 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.