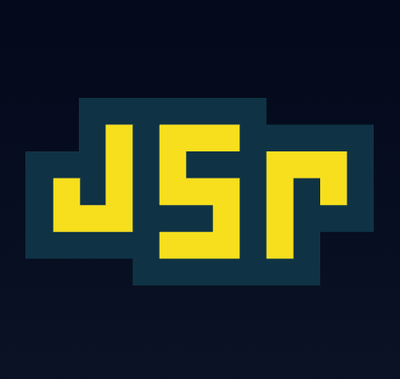
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The es5-ext package is a collection of ECMAScript 5 extensions. It provides polyfills for some of the newer ECMAScript features, as well as additional utility functions that enhance the JavaScript standard library.
Array polyfills and extensions
Adds new methods to the Array prototype, such as 'contains', which checks if an array includes a certain element.
[1, 2, 3].contains(2)
Object polyfills and extensions
Introduces new functions to work with objects, like 'isObject', which determines if a value is an object.
Object.isObject({})
String polyfills and extensions
Provides additional methods for strings, for instance, 'startsWith', which checks if a string starts with the specified characters.
'hello'.startsWith('he')
Function polyfills and extensions
Enhances functions with extra capabilities such as 'noop', which is an empty function that does nothing.
(function () {}).noop()
Number polyfills and extensions
Offers new properties and methods for numbers, like 'isFinite', which checks if a value is a finite number.
Number.isFinite(Infinity)
Core-js is a modular standard library for JavaScript, which includes polyfills for ECMAScript up to 2021. It's more comprehensive than es5-ext, covering a wider range of ECMAScript features.
Lodash is a utility library that provides a lot of the same functionality as es5-ext, such as working with arrays, objects, and functions. It is known for its performance and consistency across browsers.
Underscore.js is a utility library that offers similar functionality to es5-ext, with a focus on functional programming. It's often compared to lodash, and while it has many of the same functions, it has a smaller footprint.
Useful functions and objects that are not part of the standard.
It's work in progress, new methods are added when needed.
Can be used in any environment that implements EcmaScript 5th edition.
To make it work with EcmaScript 3, apply es5-shim.
To use it with node:
$ npm install es5-ext
Recommended way is to require stuff you need individually:
var sequence = require('es5-ext/lib/Function/sequence')
, merge = require('es5-ext/lib/Object/merge').call;
sequence(...);
merge(...);
but you can grab whole packs:
var fnExt = require('es5-ext/lib/Function');
fnExt.curry(...);
fnExt.sequence(...);
and if you prefer take them all:
var ext = require('es5-ext');
ext.Function.curry(...);
ext.Function.sequence(...);
ext.Object.merge.call(...);
Each extension is documented at begin of its source file.
global
reserved
Extensions for Array that can be used only on Array instances, these are mainly extensions that modify Array object in-place. For more generic extensions see List section.
Array.clone()
Array.compact()
Date.clone()
Date.duration(to)
Date.format()
Date.monthDaysCount()
Date.day.floor()
Date.month.floor()
Date.year.floor()
Many of the following are inspired by http://osteele.com/sources/javascript/functional/
Function.applyBind(f)
Function.aritize(n)
Function.bindBind(f)
Function.cache()
Function.callBind(f)
Function.clone()
Function.curry([...])
Function.dscope(scope)
Function.flip()
Function.functionalize(f)
Function.getApplyArg(f)
Function.hold([n, ...])
Function.i(x)
Function.invoke(methodName[, ...])
Function.isFunction(x)
Function.k(x)
Function.lock([...])
Function.log(log)
Function.ncurry(n[, ...])
Function.noop()
Function.pluck(name)
Function.rcurry([...])
Function.rncurry(n[, ...])
Function.s(f)
Function.sequence([...])
Extensions for Array-like objects.
List.compact()
List.concat([...])
List.every(f[, o])
List.filter(f[, o])
List.findSameStartLength(l[, ...])
List.flatten()
List.forEach(f[, o])
List.isListObject(x)
List.isList(x)
List.map(f[, o])
List.peek()
List.reduce(f[, x])
List.shiftSame(l[, ...])
List.slice([start[, end]])
List.some(f[, o])
List.toArray()
Functions for sorting list objects
List.sort.length(l1, l2)
Number.pad(precision)
Following takes into account all ES5 goodies (not enumerable properties, descriptors). For more tradtional ES3 like stuff see Object.plain below
Object.bindMethods([p[, q]])
Object.every(f[, p])
Object.extend(o)
Object.invoke(args)
Object.mapToArray(cb[, scope])
Object.mergeDeep(o)
Object.merge(p)
Object.pluck(name)
Object.sameType(x)
Object.same()
Object.toDescriptor()
Object.toDescriptors()
Object.toString()
Following are about hash'es (simple enumerable key value pairs)
Object.plain.clone()
Object.plain.compare(p)
Object.plain.elevate([p])
Object.plain.every(f[, p])
Object.plain.filter(f[, p])
Object.plain.forEach(f[, p])
Object.plain.isEmpty()
Object.plain.isPlainObject()
Object.plain.link(p)
Object.plain.map(f[, p])
Object.plain.merge(p)
Object.plain.pluck(name)
Object.plain.same()
Object.plain.setTrue()
Object.plain.setValue(value)
Object.plain.set()
Object.plain.values()
String.endsWith(s)
String.format(map)
String.indent()
String.pad(n)
String.repeat(n)
String.startsWith(s)
String.trimLeftStr(s)
String.trimRightStr(s)
String.convert.dashToCamelCase(str)
FAQs
ECMAScript extensions and shims
The npm package es5-ext receives a total of 6,518,799 weekly downloads. As such, es5-ext popularity was classified as popular.
We found that es5-ext demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.