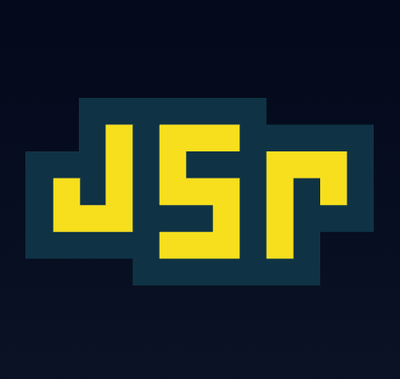
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The es5-ext package is a collection of ECMAScript 5 extensions. It provides polyfills for some of the newer ECMAScript features, as well as additional utility functions that enhance the JavaScript standard library.
Array polyfills and extensions
Adds new methods to the Array prototype, such as 'contains', which checks if an array includes a certain element.
[1, 2, 3].contains(2)
Object polyfills and extensions
Introduces new functions to work with objects, like 'isObject', which determines if a value is an object.
Object.isObject({})
String polyfills and extensions
Provides additional methods for strings, for instance, 'startsWith', which checks if a string starts with the specified characters.
'hello'.startsWith('he')
Function polyfills and extensions
Enhances functions with extra capabilities such as 'noop', which is an empty function that does nothing.
(function () {}).noop()
Number polyfills and extensions
Offers new properties and methods for numbers, like 'isFinite', which checks if a value is a finite number.
Number.isFinite(Infinity)
Core-js is a modular standard library for JavaScript, which includes polyfills for ECMAScript up to 2021. It's more comprehensive than es5-ext, covering a wider range of ECMAScript features.
Lodash is a utility library that provides a lot of the same functionality as es5-ext, such as working with arrays, objects, and functions. It is known for its performance and consistency across browsers.
Underscore.js is a utility library that offers similar functionality to es5-ext, with a focus on functional programming. It's often compared to lodash, and while it has many of the same functions, it has a smaller footprint.
Methods, functions and objects that are not part of the standard, written with EcmaScript conventions in mind.
Can be used in any environment that implements EcmaScript 5th edition.
Many extensions will also work with ECMAScript 3rd edition, if they're not let es5-shim be your aid.
To use it with node.js:
$ npm install es5-ext
For browser, you can create custom toolset with help of modules-webmake
es5-ext mostly offer methods (not functions) which can directly be assigned to native object's prototype:
Function.prototype.curry = require('es5-ext/lib/Function/prototype/curry');
Array.prototype.flatten = require('es5-ext/lib/Array/prototype/flatten');
String.prototype.startsWith = require('es5-ext/lib/String/prototype/starts-with');
However, extending native prototypes is controversial and in general discouraged,
most will agree that it's ok only if we own the context (see
extending-javascript-natives
for more views on that matter).
So when you don't want to extend native prototypes you can use methods as
functions:
var util = {};
var call = Function.prototype.call;
util.curry = call.bind(require('es5-ext/lib/Function/prototype/curry'));
util.flatten = call.bind(require('es5-ext/lib/Array/prototype/flatten'));
util.startsWith = call.bind(require('es5-ext/lib/String/prototype/starts-with'));
As with native ones most methods are generic and can be run on any object. In more detail:
Array.prototype
methods can be run on any object (any
value that's neither null nor undefined),Date.prototype
methods should be called only on Date
instances.Function.prototype
methods can be called on any callable objects (not
necessarily functions)Number.prototype
& String.prototype
methods can be called on any value, in
case of Number it’ll be degraded to number, in case of string it’ll be
degraded to string.API doesn't provide any methods for Object.prototype
as extending such in any case should be avoided. All Object
utils are provided as fuctions.
Object that represents global scope
List of EcmaScript 5th edition reserved keywords.
Additionally under keywords, future and futureStrict properties we have lists grouped thematically.
Generate an array of pregiven length built of repeated arguments.
In EcmaScript 6th Edition draft
Convert array-like object to an Array
In EcmaScript 6th Edition draft
Create an array from given arguments.
In sorted list search for index of item for which compareFn returns value closest to 0.
It's variant of binary search algorithm
Clears the array
Returns first index at which lists start to differ
Returns a copy of the list with all falsy values removed.
Whether list contains the given value.
Returns a copy of the list
Returns the array of elements that are present in context list but not present in other list.
egal version of indexOf
method
egal version of lastIndexOf
method
Returns the array of elements that are found only in context list or lists given in arguments.
Return first element for which given function returns true
Returns value for first declared index
Returns first declared index of the array
Returns flattened version of the array
forEach
starting from last element
Group list elements by value returned by cb function
Returns array of all indexes of given value
Computes the array of values that are the intersection of all lists (context list and lists given in arguments)
Returns value for last declared index
Returns last declared index of the array
Remove value from the array
some
starting from last element
Returns duplicate-free version of the array
Whether value is boolean
Whether value is date instance
If given object is not date throw TypeError in other case return it.
Returns a copy of the date object
Returns number of days of date's month
Sets the date time to 00:00:00.000
Sets date day to 1 and date time to 00:00:00.000
Sets date month to 0, day to 1 and date time to 00:00:00.000
Formats date up to given string. Supported patterns:
%Y
- Year with century, 1999, 2003%y
- Year without century, 99, 03%m
- Month, 01..12%d
- Day of the month 01..31%H
- Hour (24-hour clock), 00..23%M
- Minute, 00..59%S
- Second, 00..59%L
- Milliseconds, 000..999Whether value is error.
It returns true for all Error instances and Exception host instances (e.g. DOMException)
If given object is not error throw TypeError in other case return it.
Throws error
Some of the functions were inspired by Functional JavaScript project by Olivier Steele
Returns arguments object
arguments.call(…args) =def …args
Returns context in which function was called
context.call(x) =def x
Identity function. Returns first argument
i(x) =def x
Returns a function that will set name to value on given object
insert(name, value)(obj) =def object[name] = value
Returns a function that takes an object as an argument, and applies object's
name method to arguments.
name can be name of the method or method itself.
invoke(name, …args)(object, …args2) =def object[name](…args, …args2)
Whether value is arguments object
Wether value is instance of function
Returns a constant function that returns pregiven argument
k(x)(y) =def x
No operation function
Returns a function that takes an object, and returns the value of its name property
pluck(name)(obj) =def obj[name]
Returns a function that takes an object, and deletes object's name property
remove(name)(obj) =def delete obj[name]
Some of the methods were inspired by Functional JavaScript project by Olivier Steele
Applies the functions in argument-list order.
f1.chain(f2, f3, f4)(…args) =def f4(f3(f2(f1(…arg))))
Invoking the function returned by this function only n arguments are passed to the underlying function. If the underlying function is not saturated, the result is a function that passes all its arguments to the underlying function.
If n is not provided then it defaults to context function length
f.curry(4)(arg1, arg2)(arg3)(arg4) =def f(arg1, args2, arg3, arg4)
Returns a function that swaps its first two arguments before passing them to the underlying function.
f.flip()(a, b, c) =def f(b, a, c)
Returns a function that applies the underlying function to args, and ignores its own arguments.
f.lock(…args)(…args2) =def f(…args)
Named after it's counterpart in Google Closure
Returns a function that applies underlying function with first list argument
f.match()(args) =def f.apply(null, args)
Memoizes function results, works with any type of input arguments.
memoizedFn = memoize.call(fn);
memoizedFn('foo', 3);
memoizedFn('foo', 3); // Result taken from cache
By default fixed number of arguments that function takes is assumed (it's
read from fn.length
property) this behaviour can be overriden by providing
custom length setting e.g.:
memoizedFn = memoize(fn, 2);
or we may pass false
as length:
memoizeFn = memoize(fn, false);
which means that number of arguments is dynamic, and memoize will work with any number of them.
If we expect arguments of certain types it's good to coerce them before doing memoization. We can do that by passing additional resolvers array. Each item is function that would be run on given argument, value returned by function is accepted as coerced value.
memoizeFn = memoize(fn, 2, [String, Boolean]);
Collected cache can be cleared. To clear all collected data:
memoizedFn.clearAllCache();
or data for particall call:
memoizedFn.clearCache('foo', true);
Returns a function that returns boolean negation of value returned by underlying function.
f.not()(…args) =def !f(…args)
Returns a function that when called will behave like context function called with initially passed arguments. If more arguments are suplilied, they are appended to initial args.
f.partial(…args1)(…args2) =def f(…args1, …args2)
Returns a function that when called silents any error thrown by underlying function. If underlying function throws error, it is the result fo the function.
function () { throw err; }.silent()() ==def err
Wrap function with other function, it allows to specify before and after behavior, transform return value or prevent original function from being called.
Inspired by Prototype's wrap
In EcmaScript 6th Edition draft
Whether value is NaN. Differs from global isNaN that it doesn't do type coercion. See http://wiki.ecmascript.org/doku.php?id=harmony:number.isnan
Whether given value is number
In EcmaScript 6th Edition draft
Converts value to integer
Converts value to unsigned integer
Converts value to unsigned 32 bit integer. This type is used for array lengths. See: http://www.2ality.com/2012/02/js-integers.html
Pad given number with zeros. Returns string
Bind all object functions to given scope. If scope is not given then functions are bound to object they're assigned to. This emulates Python's bound instance methods. If source (second argument) is present then all functions from source are binded to scope and assigned to object.
Inspired by: http://mochi.github.com/mochikit/doc/html/MochiKit/Base.html#fn-bindmethods
Remove all enumerable own properties of the object
Returns clone that shares all properties of the object and have same prototype as the object
Returns copy of the object with all enumerable properties that have no falsy values
Universal cross-type compare function. To be used for e.g. array sort.
Returns copy of the object with all enumerable properties. Additionally nested objects can be copied aswell
Counts number of enumerable own properties on object
Descriptor factory.
mode is string, through we which we define whether value should be configurable, enumerable and/or writable, it's accepted as string of tokens, e.g.: c
, ce
, cew
, cw
, e
, ew
, w
If mode is not provided than cw
mode is assumed (it's how standard methods are defined on native objects).
To setup descriptor with getter and/or setter use descriptor.gs
, mode is configured same way as in value version, only difference is that settings for writable attribute are ignored.
Returns differences between two objects (taking into account only its own enumerable properties). Returned object is array of three arrays. Each array holds property names:
obj2
obj1
Analogous to Array.prototype.every. Returns true if every key-value pair in this object satisfies the provided testing function.
Optionally compareFn can be provided which assures that keys are tested in given order. If provided compareFn is equal to true
, then order is alphabetical (by key).
Extend dest by enumerable own properties of other objects. Properties found in both objects will be overwritten.
Extend dest by all own properties of other objects. Properties found in both objects will be overwritten (unless they're not configrable and cannot be overwritten).
Analogous to Array.prototype.filter. Returns new object with properites for which cb function returned truthy value.
Returns new object, with flatten properties of input object
flatten({ a: { b: 1 }, c: { d: 1 } }) =def { b: 1, d: 1 }
Analogous to Array.prototype.forEach. Calls a function for each key-value pair found in object
Optionally compareFn can be provided which assures that properties are iterated in given order. If provided compareFn is equal to true
, then order is alphabetical (by key).
Get all (not just own) property names of the object
In EcmaScript 6th Edition draft as is
operator
Whether two values are equal, takes into account NaN and -0/+0 cases
Whether object is callable
Compares two objects.
Object is considered a copy when its own enumerable properties match own enumerable properties of other object
True if object doesn't have any own enumerable property
Whether object is array-like object
Whether value is not primitive
Whether object is plain object, its protototype should be Object.prototype and it cannot be host object.
Search object for value
Analogous to Array.prototype.map. Creates a new object with properties which values are results of calling a provided function on every key-value pair in this object.
Create new object with same values, but remapped keys
Creates an array of results of calling a provided function on every key-value pair in this object.
Optionally compareFn can be provided which assures that results are added in given order. If provided compareFn is equal to true
, then order is alphabetical (by key).
Analogous to Array.prototype.some Returns true if any key-value pair satisfies the provided
testing function.
Optionally compareFn can be provided which assures that keys are tested in given order. If provided compareFn is equal to true
, then order is alphabetical (by key).
If given object is not callable throw TypeError in other case return it.
Throws error if given value is null
or undefined
, otherwise returns true
.
Return array of object own enumerable properties
Whether object is regular expression
Returns globally unique string identifier, it starts with a digit and is followed by any characters from 0-9 and a-z range. Length of string is 9 characters but may increase in future.
Simple and friendly implementation for common web application purpose (it's format is different from official GUID format)
Whether object is string
Case insensitive compare
In EcmaScript 6th Edition draft
Whether string contains given string.
Convert dash separated string to camelCase, e.g. one-two-three -> oneTwoThree
In EcmaScript 6th Edition draft
Whether strings ends with given string
Formats given template up to provided map, e.g.:
"%capital is a capital of %country".format({
capital: "Warsaw",
country: "Poland"
}); // -> "Warsaw is a capital of Poland"
Map may also provide not direct values but functions that resolve value, in that case optional thisArg determines the context in which functions are called.
Indents each line with provided str (if count given then str is repeated count times).
Return last character
Pad string with fill. If length si given than fill is reapated length times. If length is negative then pad is applied from right.
Repeat given string n times
In EcmaScript 6th Edition draft
Whether strings starts with given string
Returns string left trimmed by characters same for all strings
In EcmaScript 6th Edition draft
Returns sign of a number value
$ npm test
FAQs
ECMAScript extensions and shims
The npm package es5-ext receives a total of 6,518,799 weekly downloads. As such, es5-ext popularity was classified as popular.
We found that es5-ext demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.