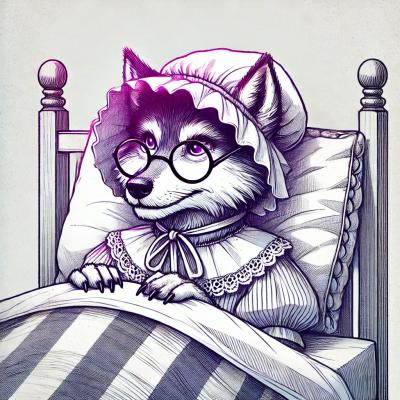
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
es6-module-loader
Advanced tools
ES6 Module Loader polyfill based on http://wiki.ecmascript.org/doku.php?id=harmony:module_loaders by Luke Hoban, Addy Osmani and Guy Bedford.
Not yet suitable for production use while the specification is still subject to change.
Download both es6-module-loader.js and traceur.js into the same folder.
Then include the es6-module-loader.js
file on its own in the page:
<script src="path/to/es6-module-loader.js"></script>
Traceur will be downloaded only when needed for ES6 syntax parsing.
If we have an ES6 module file located at /lib/app/main.js
, we can then load this with the system loader:
<script>
System.baseURL = '/lib';
System.import('app/main', function(app) {
new app.Application();
});
</script>
Any module dependencies of the file will be dynamically loaded and linked as per the ES6 module specification.
Modules are loaded by Module Name roughly using the rule:
URL = baseURL + '/' + ModuleName + '.js'
Relative module names can be written './local-module'
to load relative to the parent module name.
The contents of /lib/app/main.js
can be written:
import { Helpers } from './app-dep';
export class Application {
constructor() {
console.log('Initialized ES6 App Module');
},
foo() {
Helpers.foo();
}
}
With /lib/app/app-dep.js
containing:
export var Helpers = { ... };
When loaded, as with the System.import
call above, these module files are dynamically loaded and compiled to ES5 in the browser and executed.
When in production, one wouldn't want to load ES6 modules and syntax in the browser. Rather the modules would be built into ES5 and AMD to be loaded.
One can construct an AMD loader from this polyfill in under 30KB for such a scenario.
Think of this module as RequireJS, and Traceur as the r.js optimizer.
Bundling techniques for ES6 are an active area of development.
Modules can also be loaded with the module tag:
<script src="/path/to/es6-module-loader.js"></script>
<script>System.baseURL = '/lib'</script>
<script type="module">
import { Application } from 'app/main';
new Application();
</script>
The following module syntax is supported by this polyfill, which is to the latest specification (November 2013):
//import 'jquery'; // import a module ** awaiting support in Traceur
import $ from 'jquery'; // import the default export of a module
import { $ } from 'jquery'; // import a named export of a module
import { $ as jQuery } from 'jquery'; // import a named export to a different name
export var x = 42; // export a named variable
export function foo() {}; // export a named function
export q = {}; // export shorthand
export default 42; // export the default export
export default function foo() {}; // export the default export as a function
export { encrypt }; // export an existing variable
export { decrypt as dec }; // export a variable as a new name
export { encrypt as en } from 'crypto'; // export an export from another module
export * from 'crypto'; // export all exports from another module
module crypto from 'crypto'; // import an entire module instance object
For use in NodeJS, the Module
, Loader
and System
globals are provided as exports:
var System = require('es6-module-loader').System;
System.import('some-module', callback);
Tracuer support requires npm install traceur
, allowing ES6 syntax in NodeJS:
var System = require('es6-module-loader').System;
System.import('es6-file', function(module) {
module.classMethod();
});
To set a custom path to the Traceur parser, specify the data-traceur-src
attribute on the <script>
tag used to include the module loader.
The ES6 specification defines a loader through five hooks:
Variations of these hooks can allow creating many different styles of loader.
Evey hook is optional for a new loader, with default behaviours defined.
To create a new loader, use the Loader
constructor:
var MyLoader = new Loader({
global: window,
strict: false,
normalize: function (name, referer) {
return canonicalName;
},
resolve: function (normalized, options) {
return this.baseURL + '/' + normalized + '.js';
},
fetch: function (url, fulfill, reject, options) {
myXhr.get(url, fulfill, reject);
},
translate: function (source, options) {
return compile(source);
},
link: function (source, options) {
// use standard es6 linking
return;
// provide custom linking
// useful for providing AMD and CJS support
return {
imports: ['some', 'dependencies'],
execute: function(depA, depB) {
return new Module({
some: 'export'
});
}
};
}
});
For a more in-depth overview of creating with custom loaders, see Yehuda Katz's essay or the ES6 Module Specification.
The polyfill is in the process of being updated to the latest complete draft of the module specification.
This will alter the custom loader API entirely, but the import syntax will remain mostly identical.
To follow the current the specification changes, see https://github.com/ModuleLoader/es6-module-loader/issues?labels=specification&page=1&state=open.
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint and test your code using grunt.
Also, please don't edit files in the "dist" subdirectory as they are generated via grunt. You'll find source code in the "lib" subdirectory!
Copyright (c) 2012 Luke Hoban, Addy Osmani, Guy Bedford
Licensed under the MIT license.
FAQs
An ES6 Module Loader shim
The npm package es6-module-loader receives a total of 2,197 weekly downloads. As such, es6-module-loader popularity was classified as popular.
We found that es6-module-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.