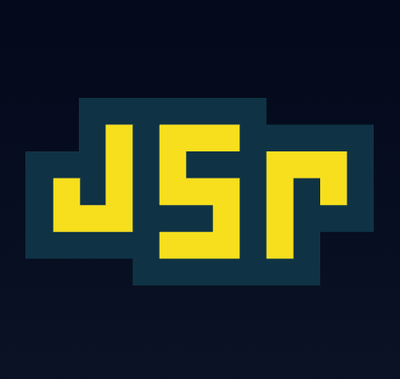
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
esbuild-loader
Advanced tools
esbuild-loader is a Webpack loader that leverages the esbuild bundler to perform fast JavaScript and TypeScript transpilation and bundling. It is designed to significantly speed up the build process by using esbuild's highly optimized and parallelized architecture.
JavaScript and TypeScript Transpilation
This feature allows you to transpile JavaScript and TypeScript files using esbuild. The code sample demonstrates how to configure esbuild-loader in a Webpack configuration to handle `.tsx` and `.ts` files, targeting ES2015 syntax.
module.exports = {
module: {
rules: [
{
test: /\.tsx?$/,
loader: 'esbuild-loader',
options: {
loader: 'tsx', // Or 'ts' if you don't need tsx
target: 'es2015' // Syntax to compile to (see options below for possible values)
}
}
]
}
};
Minification
esbuild-loader can also be used to minify JavaScript code. The code sample shows how to use the `EsbuildPlugin` to enable minification in a Webpack configuration, targeting ES2015 syntax.
const EsbuildPlugin = require('esbuild-loader').EsbuildPlugin;
module.exports = {
optimization: {
minimize: true,
minimizer: [
new EsbuildPlugin({
target: 'es2015' // Syntax to compile to (see options below for possible values)
})
]
}
};
CSS Loading
esbuild-loader can also handle CSS files. The code sample demonstrates how to configure esbuild-loader to load and minify CSS files in a Webpack configuration.
module.exports = {
module: {
rules: [
{
test: /\.css$/,
use: [
'style-loader',
'css-loader',
{
loader: 'esbuild-loader',
options: {
loader: 'css',
minify: true
}
}
]
}
]
}
};
babel-loader is a Webpack loader that uses Babel to transpile JavaScript and TypeScript files. While babel-loader is highly configurable and supports a wide range of plugins and presets, it is generally slower than esbuild-loader due to Babel's single-threaded architecture.
ts-loader is a Webpack loader specifically designed for TypeScript transpilation using the TypeScript compiler (tsc). It provides fine-grained control over TypeScript compilation but is slower compared to esbuild-loader, which uses esbuild's faster, parallelized architecture.
terser-webpack-plugin is a Webpack plugin used for minifying JavaScript files using Terser. While it is highly configurable and widely used, it is generally slower than esbuild-loader's minification capabilities, which leverage esbuild's optimized performance.
Speed up your Webpack build with esbuild! 🔥
esbuild is a JavaScript bundler written in Go that supports blazing fast ESNext & TypeScript transpilation and JS minification.
esbuild-loader lets you harness the speed of esbuild in your Webpack build by offering faster alternatives for transpilation (eg. babel-loader/ts-loader) and minification (eg. Terser)!
Curious how much faster your build will be? See what users are saying.
Support this project by ⭐️ starring and sharing it. Follow me to see what other cool projects I'm working on! ❤️
npm i -D esbuild-loader
In webpack.config.js
:
module.exports = {
module: {
rules: [
- {
- test: /\.js$/,
- use: 'babel-loader',
- },
+ {
+ test: /\.js$/,
+ loader: 'esbuild-loader',
+ options: {
+ loader: 'jsx', // Remove this if you're not using JSX
+ target: 'es2015' // Syntax to compile to (see options below for possible values)
+ }
+ },
...
],
},
}
In webpack.config.js
:
module.exports = {
module: {
rules: [
- {
- test: /\.tsx?$/,
- use: 'ts-loader'
- },
+ {
+ test: /\.tsx?$/,
+ loader: 'esbuild-loader',
+ options: {
+ loader: 'tsx', // Or 'ts' if you don't need tsx
+ target: 'es2015'
+ }
+ },
...
]
},
}
If you have a tsconfig.json
file, esbuild-loader will automatically detect it.
Alternatively, you can also pass it in directly via the tsconfigRaw
option:
{
test: /\.tsx?$/,
loader: 'esbuild-loader',
options: {
loader: 'tsx',
target: 'es2015',
+ tsconfigRaw: require('./tsconfig.json')
}
}
⚠️ esbuild only supports a subset of tsconfig
options (see TransformOptions
interface) and does not do type-checks. It's recommended to use a type-aware IDE or tsc --noEmit
for type-checking instead. It is also recommended to enable isolatedModules
and esModuleInterop
options in your tsconfig
by the esbuild docs.
You can replace JS minifiers like Terser or UglifyJs. Checkout the benchmarks to see how much faster esbuild is. The target
option tells esbuild that it can use newer JS syntax to perform better minification.
In webpack.config.js
:
+ const { ESBuildMinifyPlugin } = require('esbuild-loader')
module.exports = {
...,
+ optimization: {
+ minimizer: [
+ new ESBuildMinifyPlugin({
+ target: 'es2015' // Syntax to compile to (see options below for possible values)
+ })
+ ]
+ },
}
If you're not using TypeScript, JSX, or any syntax unsupported by Webpack, you can also leverage the minifier for transpilation (as an alternative to Babel). It will be faster because there's less files to work on and will produce a smaller output because the polyfills will only be bundled once for the entire build instead of per file. Simply set the target
option on the minifier to specify which support level you want.
There are two ways to minify CSS, depending on your setup. You should already have CSS setup in your build using css-loader
.
If your CSS is extracted and emitted as a CSS file, you can replace CSS minification plugins like css-minimizer-webpack-plugin
or optimize-css-assets-webpack-plugin
with the same ESBuildMinifyPlugin
by enabling the css
option.
Assuming the CSS is extracted using something like MiniCssExtractPlugin, in webpack.config.js
:
const { ESBuildMinifyPlugin } = require('esbuild-loader')
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
module.exports = {
...,
optimization: {
minimizer: [
new ESBuildMinifyPlugin({
target: 'es2015',
+ css: true // Apply minification to CSS assets
})
]
},
module: {
rules: [
{
test: /\.css$/i,
use: [
MiniCssExtractPlugin.loader,
'css-loader'
]
}
]
},
plugins: [
new MiniCssExtractPlugin()
]
}
If your CSS is not emitted as a CSS file, but rather loaded via JS using something like style-loader
, you can use the loader for minification.
In webpack.config.js
:
module.exports = {
...,
module: {
rules: [
{
test: /\.css$/i,
use: [
'style-loader',
'css-loader',
+ {
+ loader: 'esbuild-loader',
+ options: {
+ loader: 'css',
+ minify: true
+ }
+ }
]
}
]
}
}
If you'd like to see working Webpack builds that use esbuild-loader for basic JS, React, TypeScript, or Next.js, check out the examples repo.
esbuild-loader comes with a version of esbuild it has been tested to work with. However, esbuild has a frequent release cadence, and while we try to keep up with the important releases, it can easily go out of date.
Use the implementation
option in the loader or the minify plugin to pass in your own version of esbuild (eg. a newer one).
⚠️ esbuild is not stable yet and can have dramatic differences across releases. Using a different version of esbuild is not guaranteed to work.
+ const esbuild = require('esbuild')
...
module.exports = {
...,
module: {
rules: [
{
test: ...,
loader: 'esbuild-loader',
options: {
...,
+ implementation: esbuild
}
}
]
}
}
The implementation
option will be removed once esbuild reaches a stable release. Instead esbuild will become a peerDependency so you always provide your own.
The loader supports all Transform options from esbuild.
Note:
devtool
. sourcemap
/sourcefile
options are ignored.tsconfig.json
is automatically detected for you. You don't need to pass in tsconfigRaw
unless it's in a different path.Here are some common configurations and custom options:
Type: string | Array<string>
Default: 'es2015'
The target environment (e.g. es2016
, chrome80
, esnext
).
Read more about it in the esbuild docs.
Type: 'js' | 'jsx' | 'ts' | 'tsx' | 'css' | 'json' | 'text' | 'base64' | 'file' | 'dataurl' | 'binary' | 'default'
Default: 'js'
The loader to use to handle the file. See the type for possible values.
Read more about it in the esbuild docs.
Type: string
Default: React.createElement
Customize the JSX factory function name to use.
Read more about it in the esbuild docs.
Type: string
Default: React.Fragment
Customize the JSX fragment function name to use.
Read more about it in the esbuild docs.
Type: { transform: Function }
Custom esbuild-loader option.
Use it to pass in a different esbuild version.
The loader supports all Transform options from esbuild.
Type: string | Array<string>
Default: 'esnext'
Target environment (e.g. 'es2016'
, ['chrome80', 'esnext']
)
Read more about it in the esbuild docs.
Here are some common configurations and custom options:
Type: boolean
Default: true
Enable JS minification. Enables all minify*
flags below.
To have nuanced control over minification, disable this and enable the specific minification you want below.
Read more about it in the esbuild docs.
Type: boolean
Minify JS by removing whitespace.
Type: boolean
Minify JS by shortening identifiers.
Type: boolean
Minify JS using equivalent but shorter syntax.
Type: 'none' | 'inline' | 'eof'
Default: 'inline'
Read more about it in the esbuild docs.
Type: boolean
Default: Webpack devtool
configuration
Whether to emit sourcemaps.
Type: boolean
Default: false
Custom esbuild-loader option.
Whether to minify CSS files.
Type: string | RegExp | Array<string | RegExp>
Custom esbuild-loader option.
Filter assets to include in minification
Type: string | RegExp | Array<string | RegExp>
Custom esbuild-loader option.
Filter assets to exclude from minification
Type: { transform: Function }
Custom esbuild-loader option.
Use it to pass in a different esbuild version.
No. esbuild plugins are only available in the build API. And esbuild-loader uses the transform API instead of the build API for two reasons:
The build API is for creating JS bundles, which is what Webpack does. If you want to use esbuild's build API, consider using esbuild directly instead of Webpack.
The build API reads directly from the file-system, but Webpack loaders operate in-memory. Webpack loaders are essentially just functions that are called with the source-code as the input. Not reading from the file-system allows loaders to be chainable. For example, using vue-loader
to compile Single File Components (.vue
files), then using esbuild-loader
to transpile just the JS part of the SFC.
No. The inject
option is only available in the build API. And esbuild-loader uses the transform API.
However, you can use the Webpack equivalent ProvidePlugin instead.
If you're using React, check out this example on how to auto-import React in your components.
No. If you really need them, consider porting them over to a Webpack loader.
And please don't chain babel-loader
and esbuild-loader
. The speed gains come from replacing babel-loader
.
Running esbuild as a standalone bundler vs esbuild-loader + Webpack are completely different:
Using any JS bundler introduces a bottleneck that makes reaching those speeds impossible. However, esbuild-loader can still speed up your build by removing the bottlenecks created by babel-loader
, ts-loader
, Terser, etc.
According to the esbuild FAQ, it will not be supported.
Consider these type-checking alternatives:
tsc --noEmit
to type checkfork-ts-checker-webpack-plugin
Webpack-integrated Mocha test-runner with Webpack 5 support.
Localize/i18nalize your Webpack build. Optimized for multiple locales!
FAQs
⚡️ Speed up your Webpack build with esbuild
The npm package esbuild-loader receives a total of 959,966 weekly downloads. As such, esbuild-loader popularity was classified as popular.
We found that esbuild-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.