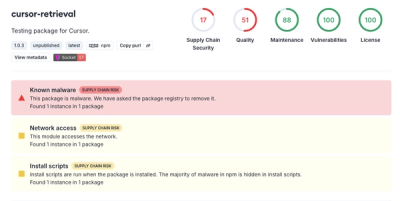
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
esbuild is a fast JavaScript bundler and minifier. It compiles TypeScript and JavaScript into a single file, minifies it, and can also handle CSS and image assets. It's designed for speed and efficiency, utilizing parallelism and native Go code to achieve its performance.
Bundling JavaScript
This code bundles 'app.js' and its dependencies into a single file 'out.js'.
require('esbuild').build({
entryPoints: ['app.js'],
bundle: true,
outfile: 'out.js'
}).catch(() => process.exit(1))
Minifying JavaScript
This code minifies 'app.js' to reduce file size and improve load times.
require('esbuild').build({
entryPoints: ['app.js'],
minify: true,
outfile: 'out.js'
}).catch(() => process.exit(1))
Transpiling TypeScript
This code compiles a TypeScript file 'app.ts' into JavaScript and bundles it into 'out.js'.
require('esbuild').build({
entryPoints: ['app.ts'],
bundle: true,
outfile: 'out.js'
}).catch(() => process.exit(1))
Serving files for development
This code starts a local server to serve files from the 'public' directory and bundles 'app.js' into 'public/out.js'.
require('esbuild').serve({
servedir: 'public',
port: 8000
}, {
entryPoints: ['app.js'],
bundle: true,
outfile: 'public/out.js'
}).then(server => {
// Server started
})
Webpack is a powerful and widely-used module bundler. It offers a rich plugin ecosystem and a highly configurable build process. Compared to esbuild, webpack is more mature with more features but is generally slower due to its JavaScript-based architecture.
Rollup is another JavaScript module bundler that focuses on producing efficient bundles for modern module formats like ES modules. It's known for its tree-shaking capabilities. Rollup is typically faster than webpack but slower than esbuild.
Parcel is a web application bundler that offers zero configuration out-of-the-box. It's faster than webpack and rollup but generally not as fast as esbuild. Parcel has a simpler user experience but may not be as flexible for complex configurations.
Terser is a JavaScript parser, mangler, and compressor toolkit for ES6+. It's often used for minifying JavaScript code. While esbuild also minifies code, terser is a dedicated tool for this purpose and can be used alongside other bundlers.
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the JavaScript API documentation for details.
0.23.1
Allow using the node:
import prefix with es*
targets (#3821)
The node:
prefix on imports is an alternate way to import built-in node modules. For example, import fs from "fs"
can also be written import fs from "node:fs"
. This only works with certain newer versions of node, so esbuild removes it when you target older versions of node such as with --target=node14
so that your code still works. With the way esbuild's platform-specific feature compatibility table works, this was added by saying that only newer versions of node support this feature. However, that means that a target such as --target=node18,es2022
removes the node:
prefix because none of the es*
targets are known to support this feature. This release adds the support for the node:
flag to esbuild's internal compatibility table for es*
to allow you to use compound targets like this:
// Original code
import fs from 'node:fs'
fs.open
// Old output (with --bundle --format=esm --platform=node --target=node18,es2022)
import fs from "fs";
fs.open;
// New output (with --bundle --format=esm --platform=node --target=node18,es2022)
import fs from "node:fs";
fs.open;
Fix a panic when using the CLI with invalid build flags if --analyze
is present (#3834)
Previously esbuild's CLI could crash if it was invoked with flags that aren't valid for a "build" API call and the --analyze
flag is present. This was caused by esbuild's internals attempting to add a Go plugin (which is how --analyze
is implemented) to a null build object. The panic has been fixed in this release.
Fix incorrect location of certain error messages (#3845)
This release fixes a regression that caused certain errors relating to variable declarations to be reported at an incorrect location. The regression was introduced in version 0.18.7 of esbuild.
Print comments before case clauses in switch statements (#3838)
With this release, esbuild will attempt to print comments that come before case clauses in switch statements. This is similar to what esbuild already does for comments inside of certain types of expressions. Note that these types of comments are not printed if minification is enabled (specifically whitespace minification).
Fix a memory leak with pluginData
(#3825)
With this release, the build context's internal pluginData
cache will now be cleared when starting a new build. This should fix a leak of memory from plugins that return pluginData
objects from onResolve
and/or onLoad
callbacks.
FAQs
An extremely fast JavaScript and CSS bundler and minifier.
The npm package esbuild receives a total of 26,653,082 weekly downloads. As such, esbuild popularity was classified as popular.
We found that esbuild demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.