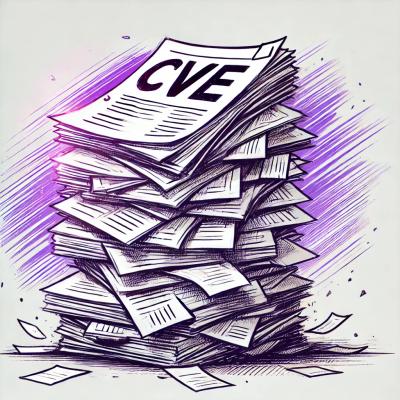
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
eslint-config-esnext
Advanced tools
Pluggable eslint config for ECMAScript Next that you can import, extend and override
In your js project directory:
npm install --save-dev eslint-config-esnext
And in your .eslintrc.yaml
:
---
extends:
- esnext
Alternatively, in your .eslintrc.js
or .eslintrc.json
:
{
"extends": ["esnext"]
}
To add a git-hook to your commits, consider using husky
npm install --save-dev husky
And in your package.json
:
"scripts": {
"precommit": "eslint ."
}
This config is biased and opinionated, and errs on the side of too many rules instead of too few. Think of this as a superset of your repo's lint config, and discard what you don't like in it. It's easy to override and disable the rules you find inconvenient.
env:
es6: true
commonjs: true
enables ES6 features and CommonJS modules
parser: babel-eslint
enables parsing all babel supported code
parserOptions:
ecmaVersion: 7
sourceType: module
ecmaFeatures:
impliedStrict: true
modules: true
experimentalObjectRestSpread: true
allows es2015 modules and es2016 object rest and spread to be parsed, and applies strict mode to all js code
extends:
- eslint:recommended
- plugin:import/errors
- plugin:import/warnings
includes the following rules:
constructor-super
: require super()
calls in constructors
no-case-declarations
: disallow let
, const
, function
and class
declarations in case
/ default
clauses inside switch
blocks
no-class-assign
: disallow reassigning variables declared as classes
no-cond-assign
: disallow assignment operators in conditional expressions
no-console
: disallow the use of console
no-const-assign
: disallow reassigning const
variables
no-constant-condition
: disallow constant expressions in conditions
no-control-regex
: disallow control characters in regular expressions
no-debugger
: disallow the use of debugger
no-delete-var
: disallow deleting variables
no-dupe-args
: disallow duplicate arguments in function
definitions
no-dupe-class-members
: disallow duplicate class members
no-dupe-keys
: disallow duplicate keys in object literals
no-duplicate-case
: disallow duplicate case labels
no-empty
: disallow empty block statements
no-empty-character-class
: disallow empty character classes in regular expressions
no-empty-pattern
: disallow empty destructuring patterns
no-ex-assign
: disallow reassigning exceptions in catch
clauses
no-extra-boolean-cast
: disallow unnecessary boolean casts
no-extra-semi
: disallow unnecessary semicolons
no-fallthrough
: disallow fallthrough of case
statements
no-func-assign
: disallow reassigning function
declarations
no-inner-declarations
: disallow function
or var
declarations in nested blocks
no-invalid-regexp
: disallow invalid regular expression strings in RegExp
constructors
no-irregular-whitespace
: disallow irregular whitespace outside of strings and comments
no-mixed-spaces-and-tabs
: disallow mixed spaces and tabs for indentation
no-new-symbol
: disallow new
operators with the Symbol
object
no-obj-calls
: disallow calling global object properties as functions
no-octal
: disallow octal literals
no-redeclare
: disallow var
redeclaration
no-regex-spaces
: disallow multiple spaces in regular expression literals
no-self-assign
: disallow assignments where both sides are exactly the same
no-sparse-arrays
: disallow sparse arrays
no-this-before-super
: disallow this
/super
before calling super()
in constructors
no-undef
: disallow the use of undeclared variables unless mentioned in /-global -/
comments
no-unexpected-multiline
: disallow multiline expressions likely to cause ASI errors
no-unreachable
: disallow unreachable code after return
, throw
, continue
, and break
statements
no-unsafe-finally
: disallow control flow statements in finally
blocks
no-unused-labels
: disallow unused labels
no-unused-vars
: disallow unused variables
require-yield
: require generator functions to contain yield
use-isnan
: disallow comparisons with NaN
, requiring calls to isNaN()
instead
valid-typeof
: enforce comparing typeof
expressions against valid type strings
import/no-unresolved
: ensure imports point to a file/module that can be resolved
import/named
: ensure named imports correspond to a named export in the remote file
import/namespace
: ensure imported namespaces contain dereferenced properties as they are dereferenced
import/default
: ensure a default export is present, given a default import
import/export
: report any invalid exports, i.e. re-export of the same name
import/no-named-as-default
: report use of exported name as identifier of default export; set to warn only
import/no-named-as-default-member
: report use of exported name as property of default export; set to warn only
rules:
selected from here, configured to:
array-callback-return
: enforce return
statements in callbacks to array prototype methods such as map
, reduce
, find
etc.arrow-body-style
: require braces around arrow function bodies, as-needed
class-methods-use-this
: disallow class methods that don't use this
dot-notation
: enforce dot notation for accessing object properties whenever possibleeqeqeq
: prefer ===
and !==
over ==
and !=
import/no-amd
: report AMD require
and define
callsimport/no-commonjs
: report CommonJS require
calls and module.exports
or exports.*
import/no-duplicates
: report repeated import of the same module in multiple placesimport/no-extraneous-dependencies
: forbid the use of extraneous packagesimport/no-mutable-exports
: forbid the use of mutable exports with var
or let
import/no-namespace
: report namespace importsimport/no-nodejs-modules
: disallow node.js builtin modulesimport/prefer-default-export
: prefer a default export if module exports a single nameno-alert
: disallow the use of alert
, confirm
, and prompt
no-constant-condition
: override eslint:recommended
with checkLoops: false
to avoid errors in infinite generatorsno-duplicate-imports
: disallow duplicate module importsno-empty-function
: disallow empty functionsno-else-return
: disallow else
blocks after return
statements in if
blocksno-eval
: disallow the use of eval()
no-extend-native
: disallow extending built-in or native objectsno-extra-bind
: disallow binding functions that don't use this
no-global-assign
: disallow assignments to native objects or read-only global variablesno-implicit-globals
: disallow var
and named function
declarations in the global scope, doesn't apply to modulesno-implied-eval
: disallow the use of eval()-like methodsno-invalid-this
: disallow this
outside of constructors, classes or methodsno-lonely-if
: disallow if
statements as the only statement in else
blocksno-loop-func
: disallow function
s inside loopsno-new
: disallow new
operators outside of assignments or comparisonsno-new-func
: disallow creating functions with the Function
constructorno-new-wrappers
: disallow creating objects with the String
, Number
, and Boolean
constructorsno-proto
: disallow use of the __proto__
propertyno-script-url
: disallow javascript:
urlsno-self-compare
: disallow comparisons where both sides are exactly the sameno-throw-literal
: disallow throwing literals as exceptionsno-unmodified-loop-condition
: enforce updating the loop condition in each iterationno-unneeded-ternary
: disallow ternary operators when simpler alternatives exist; defaultAssignment: false
prefers ||
for default assignmentsno-unsafe-negation
: disallow negating the left operand of relational operators like in
and instanceof
no-unused-expressions
: disallow expressions that have no effect on the state of the program, with allowShortCircuit: true
and allowTernary: true
allowing &&
, ||
and the ternary operator as shorthands for if
and else
no-use-before-define
: disallow the use of variables before they are defined; nofunc
ignores function
declarations since they're hoistedno-useless-call
: disallow unnecessary .call()
and .apply()
no-useless-computed-key
: disallow unnecessary computed property keys in object literalsno-useless-concat
: disallow unnecessary concatenation of literals or template literalsno-useless-constructor
: disallow unnecessary constructorsno-useless-escape
: disallow unnecessary escape charactersno-useless-rename
: disallow renaming import
, export
, and destructured assignments to the same nameno-var
: require let
or const
instead of var
no-with
: disallow with
statementsobject-shorthand
: require method and property shorthand syntax for object literalsoperator-assignment
: require assignment operator shorthand where possibleprefer-arrow-callback
: require callbacks to be arrow functionsprefer-const
: require const
declarations for variables that are never reassigned after declaredprefer-rest-params
: require rest parameters instead of arguments
prefer-spread
: require spread operator instead of .apply()
FAQs
Pluggable eslint config for ECMAScript Next that you can import, extend and override
We found that eslint-config-esnext demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.