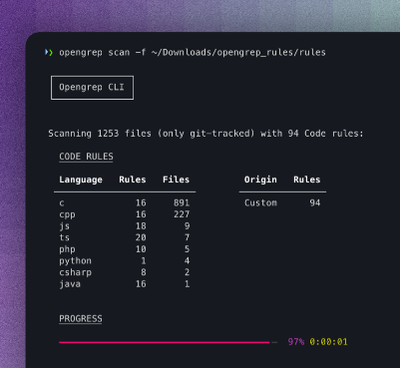
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
eslint-config-pagarme-base
Advanced tools
Base Pagar.me's JS ESLint config, following our styleguide
Our style guide is based on Airbnb's. Differences between them are described below.
The rules described in this repository are also available as a eslint config package. To install the package and its dependencies:
$ npm install --save-dev eslint@5.3.0 \
eslint-plugin-import@2.16.0 \
eslint-config-pagarme-base
The peer dependencies specified above have hardcoded versions. If you prefer you can use the command
npm info eslint-config-airbnb-base@latest peerDependencies
to find the exact peer dependencies to install.
To include in the project, create an .eslintrc
file with at least the
following contents:
{
"extends": ["pagarme-base"]
}
Do not use semicolons.
Wrong:
function transaction(amount) {
}
Right:
function transaction (amount) {
}
Wrong:
transaction(amount,
installments)
Right:
transaction(
amount,
installments
)
Wrong:
const array = [1, 2, 3,]
Right:
const array = [1, 2, 3]
Wrong:
const array = [
1,
2,
3
]
Right:
const array = [
1,
2,
3,
]
Wrong:
const obj = {
a: 1,
b: 2,
c: 3
}
Right:
const obj = {
a: 1,
b: 2,
c: 3,
}
Dangling comma may cause weird errors when used on functions, you should avoid it.
Wrong:
Object.assign(
{},
b,
c,
)
Right:
Object.assign(
{},
b,
c
)
Avoid having lines of code that are longer than 80 characters (including whitespace).
A function should have no more than 3 parameters. Consider using an options
object
parameter if you need to pass in more values to a function.
Disallow the use of 2+ empty lines in the middle of the code.
Wrong:
const a = 'This is a test'
console.log(a)
Right:
const a = 'This is a test'
console.log(a)
FAQs
Base Pagar.me's JS ESLint config, following our styleguide
We found that eslint-config-pagarme-base demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.