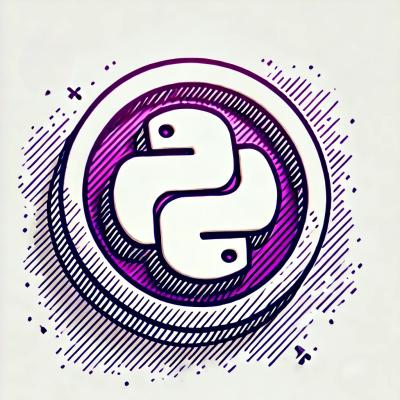
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
eslint-config-relicx
Advanced tools
An ESLint config that self-adapting to project dependencies and TypeScript configuration.
An ESLint config that self-adapting to project dependencies and TypeScript configuration.
npm install --save-dev eslint-config-relicx eslint
pnpm add --save-dev eslint-config-relicx eslint
You profit automatically of automatic dependency and feature detection. Due to the nature of ESLint configs however this approach is significantly harder to customize.
// .eslintrc.js
module.exports = {
extends: "relicx",
};
This showcases the required setup to begin with customizing your config on an advanced level. Please check out the Examples
section below for more details.
// .eslintrc.js
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
module.exports = createConfig();
// .eslintrc.js
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
module.exports = createConfig({
incrementalAdoption: true,
});
You like all the features eslint-config-relicx
ships with but you heavily disagree with many rule settings?
Say no more. Simply pass { blankSlate: true }
to createConfig
and you still benefit from automatic dependency detection, the general override setup based on file patterns, but every rule will be set to off
.
This way, you can customize it entirely to your likings without having to create n overrides for rules and or rulesets.
While in the process of migration, you may end up in a situation where you cannot turn on compilerOptions.checkJs
from TypeScript itself due to e.g. builds breaking. However, by default certain rules will be disabled for JavaScript files because they are technically shadowed by TypeScript itself, e.g. no-undef
.
You can opt out of this behaviour by either:
enableJavaScriptSpecificRulesInTypeScriptProject
as true
to createConfig
compilerOptions.checkJs
once you're thereExample:
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
module.exports = createConfig({
enableJavaScriptSpecificRulesInTypeScriptProject: true,
});
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
const { getDependencies } = require("eslint-config-relicx/lib/getDependencies");
const {
createTypeScriptOverride,
} = require("eslint-config-relicx/lib/overrides/typescript");
const dependencies = getDependencies();
const customTypescriptOverride = createTypeScriptOverride({
...dependencies,
rules: {
// here goes anything that applies **exclusively** to typescript files based on the `files` glob pattern also exported from ../overrides/typescript
"@typescript-eslint/explicit-module-boundary-types": "warn", // downgrading the default from "error" to "warn"
},
});
module.exports = createConfig({
overrides: [customTypescriptOverride],
});
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
const { getDependencies } = require("eslint-config-relicx/lib/getDependencies");
const {
createReactOverride,
} = require("eslint-config-relicx/lib/overrides/react");
const dependencies = getDependencies();
const customReactOverride = createReactOverride({
...dependencies,
rules: {
"unicorn/no-abusive-eslint-disable": "off",
},
});
module.exports = createConfig({
overrides: [customReactOverride],
});
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
const { getDependencies } = require("eslint-config-relicx/lib/getDependencies");
const {
createReactOverride,
} = require("eslint-config-relicx/lib/overrides/react");
const dependencies = getDependencies();
const customReactOverride = createReactOverride({
...dependencies,
plugins: ["my-fancy-plugin"],
rules: {
"plugin/foo": "warn",
"plugin/bar": "error",
"plugin/baz": "off",
},
});
module.exports = createConfig({
overrides: [customReactOverride],
});
const { getDependencies } = require("eslint-config-relicx/lib/getDependencies");
const {
files,
parser,
defaultSettings,
} = require("eslint-config-relicx/lib/overrides/react");
const dependencies = getDependencies();
const myReactOverride = {
// using the internal react glob pattern
files,
// using the internal default react parser
parser,
// defining your custom rules
rules: {
"react/react-in-jsx-scope": "warn",
},
// using the default settings
settings: defaultSettings,
};
module.exports = {
overrides: [myReactOverride],
rules: {
"no-await-in-loop": "warn",
},
};
Everything is dynamically included based on your package.json
and when using TypeScript, your tsconfig.json
.
Rules are selectively applied based on file name patterns.
All rules are commented and link to their docs.
All rulesets and overrides are created through functions accepting an object matching this schema:
interface Project {
/**
* whether `@types/node` is present
*/
hasNodeTypes: boolean;
/**
* whether `@nestjs/core` is present
*/
hasNest: boolean;
typescript: {
/**
* whether `typescript` is present
*/
hasTypeScript: boolean;
/**
* the installed version
*/
version: string;
/**
* your tsConfig; used to detect feature availability
*/
config?: object;
};
react: {
/**
* whether any flavour of react is present
*/
hasReact: boolean;
/**
* whether `preact` is present
* currently without effect
*/
isPreact: boolean;
/**
* the installed version
*/
version: string;
};
/**
* your custom rules on top
*/
rules?: object;
}
This list only mentions the exports most people will need. For an exhaustive list, check out the source.
const { createTypeScriptOverride } = require('eslint-config-relicx/lib/overrides/typescript')
const { createReactOverride } = require('eslint-config-relicx/lib/overrides/react')
Please note that the test override should always come last.
const { createEslintCoreRules } = require('eslint-config-relicx/lib/plugins/eslint-core')
const { createImportRules } = require('eslint-config-relicx/lib/plugins/import')
const { createPromiseRules } = require('eslint-config-relicx/lib/plugins/promise')
const { createSonarjsRules } = require('eslint-config-relicx/lib/plugins/sonarjs')
const { createUnicornRules } = require('eslint-config-relicx/lib/plugins/unicorn')
const { createConfig } = require("eslint-config-relicx/lib/createConfig");
const {
createTypeScriptOverride,
} = require("eslint-config-relicx/lib/overrides/typescript");
const packageJson = require("./package.json");
// since `createTypeScriptOverride` is entirely configurable, we need to inform it about its environment
const tsOverrideConfig = {
react: {
hasReact: true,
},
rules: {
"@typescript-eslint/ban-ts-comment": "off",
},
typescript: {
hasTypeScript: true,
// sync with package.json should you upgrade TS
version: packageJson.dependencies.typescript,
},
};
// solely an override for TS
const tsOverride = createTypeScriptOverride(tsOverrideConfig);
// pass it into createConfig as array as it will be merged with the other overrides
module.exports = createConfig({ overrides: [tsOverride] });
This project follows semver.
This project uses code from following third-party projects:
Licenses are list in THIRD-PARTY-LICENSE
FAQs
An ESLint config that self-adapting to project dependencies and TypeScript configuration.
The npm package eslint-config-relicx receives a total of 0 weekly downloads. As such, eslint-config-relicx popularity was classified as not popular.
We found that eslint-config-relicx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.