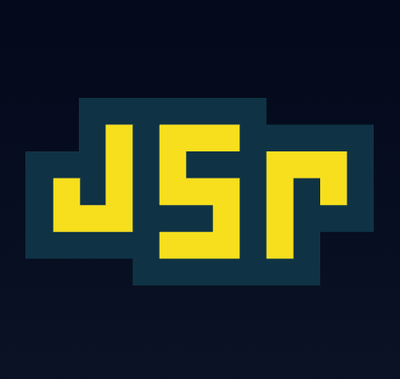
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
eslint-config-spreetail
Advanced tools
This package provides an extensible ESLint configuration used at Spreetail.
This is intended for use with ES6+ projects.
You will need ESLint installed.
This package can be installed from npm:
npm install eslint-config-spreetail --save-dev
Once installed, add "extends": "spreetail"
to your project's .eslintrc
file:
{
"extends": "spreetail"
}
If you are using ES6 modules, be sure to add "sourceType": "module"
to a "parserOptions"
section of your project's .eslintrc
file as well:
{
"parserOptions": {
"sourceType": "module"
}
}
Every rule in the configuration should be documented here with an explanation of its inclusion. It's important to include only rules with clear benefits and avoid rules which amount to little more than personal preference. The purpose of this configuration is to aid developers rather than to coerce conformity. Therefore, it may be a bit less strict than other configurations.
Rules with an asterisk (*) will issue warnings rather than errors.
This will lead to poor performance. Use Promise.all()
instead.
This is almost certainly a mistake.
Comparing to -0
will pass for 0 and -0 which is confusing.
This is almost certainly a mistake.
This is almost certainly a mistake.
This is almost certainly a mistake.
Empty block statements are almost certainly unintentional and may be a sign of incomplete code.
Empty character classes won't match anything and are probably just a sign of unfinished regex.
Reassigning to an exception in a catch
block is destructive.
This is almost certainly a mistake.
Reassigning a function declaration (outside of the function itself) is probably a mistake and otherwise will lead to extremely confusing code.
This will throw an error.
This is not allowed as of ES5.
It's not safe to assume the default Object.prototype
methods are accessible by every object since it's possible to create objects without the default prototype.
This is almost certainly a mistake.
This is almost certainly a mistake. Backticks (`) were probably intended.
This may not behave as expected due to automatic semicolon insertion.
This is almost certainly a mistake.
This does not behave as expected.
This is almost certainly a mistake.
Comparisons directly to NaN
do not behave as expected.
This is almost certainly a mistake.
This is almost certainly a mistake.
var
scoping is confusing.
This is almost certainly a mistake.
Omitting curly braces can lead to code that does not behave as expected. For example:
if (foo)
bar();
baz();
This is actually equivalent to:
if (foo) {
bar();
}
baz();
Any benefits of omitting curly braces is surely outweighed by the potential for confusion.
The ==
and !=
operators use type coercion which may not behave as expected.
Be explicit and use ===
and !==
instead.
The smart option for this rule is enabled.
The default alert
, confirm
, and prompt
UI elements will block the event loop (not to mention they are horrid).
This is not allowed as of ES5.
This does not behave as expected.
This is almost certainly a mistake.
This is almost certainly a mistake.
This is almost certainly a mistake.
This does not behave as expected.
While there may be valid use cases for eval()
, they are extremely rare, and eval()
can be dangerous.
Modifying built-in prototypes can cause conflicts with other scripts and libraries. Use a function instead:
// BAD
String.prototype.getLength = function() {
return this.length;
}
const helloLength = 'hello'.getLength();
// GOOD
function getStringLength(string) {
return string.length;
}
const helloLength = getStringLength('hello');
This is almost certainly a mistake.
This is far more likely to be a mistake than intentional.
This is almost certainly a mistake.
This may conflict with other scripts and libraries and should be avoided.
See no-eval.
This will throw an error in strict mode.
This is obsolete as of ES6.
This is almost certainly a mistake.
This does not behave as expected.
new
should only be used with constructors.
See no-eval.
This does not behave as expected.
This is deprecated as of ES5.
This is deprecated as of ES5.
This is deprecated as of ES3.1.
This is almost certainly a mistake.
This is almost certainly a mistake.
See no-eval.
This is almost certainly a mistake.
This is almost certainly a mistake.
This is more likely to be a mistake than intentional.
Only Error
objects should be thrown, as they provide information about where they originated.
This is almost certainly a mistake.
This is almost certainly a mistake.
This is almost certainly a mistake.
This is too ambiguous. Use a new variable instead:
my.really.long.variable.has.some.properties.named = {
thing1: 'and',
thing2: '!'
};
// BAD
with (my.really.long.variable.has.some.properties.named) {
console.log(thing1, thing2);
}
// GOOD
const named = my.really.long.variable.has.some.properties.named;
console.log(named.thing1, named.thing2);
Promises should always reject with an Error
object so the consumers know exactly what to expect.
Additionally, Error
objects provide information about where they originated.
Calling parseInt()
with a string that starts with '0' will force evaluation as an octal, which is almost certainly a mistake. Always pass the intended radix parameter (probably 10
):
// BAD
parseInt('071'); // returns 57
// GOOD
parseInt('071', 10); // returns 71
This is almost certainly a mistake.
Attempting to immediately execute a function declaration will cause a SyntaxError
.
This should be wrapped in parentheses instead.
This should be in strict mode.
This is almost certainly a mistake (or perhaps you need to specify your project's environment or its globals in your .eslintrc
file).
This may not behave as expected. Use typeof myVar === 'undefined'
instead.
This is almost certainly a mistake.
var
hoisting can lead to confusing code.
If variables are used before they are declared, it may be indicative of a mistake.
In most cases, curly brace placement is a matter of personal preference (and such rules do not belong is this configuration). However, there is one situation in which curly brace placement may alter the behavior of code:
// BAD
function getValue()
{
return
{
value: 'here it is!'
};
}
Due to automatic semicolon insertion, in this example a semicolon will be inferred after the return
statement and getValue()
will actually return undefined
, not the object that was probably intended to be returned.
For this reason, the "one true brace style" is enforced by this configuration.
// GOOD
function getValue() {
return {
value: 'here it is!'
};
}
This is for the sole purpose of generating cleaner git diffs.
Only constructors should begin with a capital letter to act as a visual differentiator between constructors and other functions or properties.
This may not behave as expected. For example:
// BAD
const myArray = new Array(1, 2); // [1, 2]
const myOtherArray = new Array(3); // [undefined, undefined, undefined]
Use array literals ([]
) instead:
// GOOD
const myArray = [1, 2]; // [1, 2]
const myOtherArray = [3]; // [3]
Automatic semicolon insertion may not behave as expected. Use semicolons instead of relying on ASI.
This will raise a runtime error.
This is almost certainly a mistake.
This will raise a runtime error.
This is almost certainly a mistake.
This is almost certainly a mistake.
This will throw a TypeError
.
This raises a reference error.
This is almost certainly a mistake.
var
behavior can be confusing and unexpected.
Use const
or let
instead.
See this article for more information.
The arguments
object does not behave as expected.
It is "array-like" rather than a real array.
Instead of using arguments
, use rest parameters.
This is almost certainly a mistake.
This will throw a TypeError
.
Licensed under the MIT License.
FAQs
ESLint configuration used at Spreetail.
We found that eslint-config-spreetail demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.