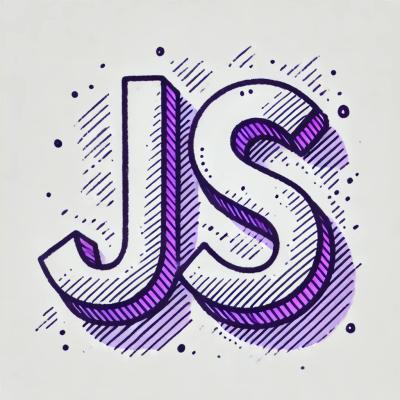
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
eslint-plugin-jest
Advanced tools
The eslint-plugin-jest package is an ESLint plugin that provides linting rules for Jest, a popular JavaScript testing framework. It helps maintain code quality and enforce best practices by analyzing test files for common issues and stylistic preferences.
Enforcing consistent test descriptions
This rule enforces a consistent test function name, either `test` or `it`, within the test files.
/* eslint jest/consistent-test-it: ["error", { fn: "test" }] */
// Incorrect
describe('myFeature', () => {
it('does something', () => {
// test implementation
});
});
// Correct
describe('myFeature', () => {
test('does something', () => {
// test implementation
});
});
Preventing disabled tests
This rule prevents the use of `xdescribe`, `xit`, or `test.skip`, which are used to disable tests, to ensure that all tests are run.
/* eslint jest/no-disabled-tests: "error" */
// Incorrect
xdescribe('myFeature', () => {
test('does something', () => {});
});
xit('does something', () => {});
// Correct
describe('myFeature', () => {
test('does something', () => {});
});
Ensuring tests contain assertions
This rule ensures that test blocks contain at least one assertion call, which is necessary for a meaningful test.
/* eslint jest/expect-expect: "error" */
// Incorrect
test('does something', () => {
// no assertions
});
// Correct
test('does something', () => {
expect(something).toBe(true);
});
Disallowing identical titles
This rule disallows using the same title for multiple test cases or `describe` blocks, which can cause confusion when trying to identify tests.
/* eslint jest/no-identical-title: "error" */
// Incorrect
describe('myFeature', () => {
test('does something', () => {});
test('does something', () => {});
});
// Correct
describe('myFeature', () => {
test('does something', () => {});
test('does something else', () => {});
});
This package provides linting rules for Mocha, another JavaScript test framework. It is similar to eslint-plugin-jest but tailored for Mocha's API and conventions.
This package offers linting rules for Jasmine, a behavior-driven development framework for testing JavaScript code. It serves a similar purpose to eslint-plugin-jest but is specific to Jasmine's syntax and style.
This ESLint plugin enforces best practices for the Testing Library, which is often used with Jest for testing React components. It complements eslint-plugin-jest by focusing on the specific patterns and practices of the Testing Library.
This plugin provides linting rules for Cypress, an end-to-end testing framework. While it is not specific to Jest, it offers similar functionality for maintaining code quality in a different testing context.
Eslint plugin for Jest
$ yarn install eslint eslint-plugin-jest --dev
Note: If you installed ESLint globally then you must also install eslint-plugin-jest
globally.
Add jest
to the plugins section of your .eslintrc
configuration file. You can omit the eslint-plugin-
prefix:
{
"plugins": [
"jest"
]
}
Then configure the rules you want to use under the rules section.
{
"rules": {
"jest/no-exclusive-tests": 2,
"jest/no-identical-title": 2
}
}
FAQs
ESLint rules for Jest
The npm package eslint-plugin-jest receives a total of 9,448,000 weekly downloads. As such, eslint-plugin-jest popularity was classified as popular.
We found that eslint-plugin-jest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.