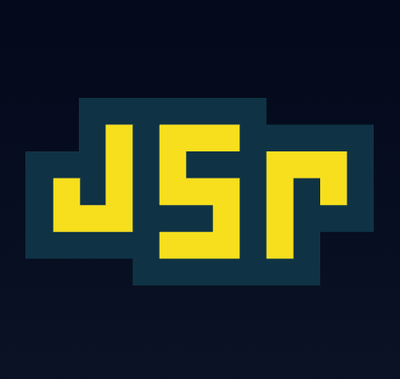
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
ethereumjs-tx
Advanced tools
An simple module for creating, manipulating and signing ethereum transactions
The ethereumjs-tx package is a JavaScript library for creating, signing, and serializing Ethereum transactions. It is part of the ethereumjs suite of tools and is widely used for interacting with the Ethereum blockchain at a low level.
Creating a Transaction
This feature allows you to create a new Ethereum transaction by specifying parameters such as nonce, gas price, gas limit, recipient address, value, and data.
const Tx = require('ethereumjs-tx').Transaction;
const txParams = {
nonce: '0x00',
gasPrice: '0x09184e72a000',
gasLimit: '0x2710',
to: '0x0000000000000000000000000000000000000000',
value: '0x00',
data: '0x00',
};
const tx = new Tx(txParams);
Signing a Transaction
This feature allows you to sign a transaction using a private key. The transaction must be signed before it can be sent to the Ethereum network.
const privateKey = Buffer.from('your_private_key', 'hex');
tx.sign(privateKey);
Serializing a Transaction
This feature allows you to serialize a signed transaction into a format that can be sent over the network. The serialized transaction is typically represented as a hexadecimal string.
const serializedTx = tx.serialize();
console.log(serializedTx.toString('hex'));
Web3.js is a comprehensive library for interacting with the Ethereum blockchain. It provides higher-level abstractions for sending transactions, interacting with smart contracts, and querying blockchain data. Unlike ethereumjs-tx, which focuses on low-level transaction creation and signing, Web3.js offers a broader range of functionalities.
Ethers.js is a lightweight library for interacting with the Ethereum blockchain. It provides utilities for creating and signing transactions, interacting with smart contracts, and querying blockchain data. Ethers.js is known for its simplicity and ease of use, making it a popular alternative to ethereumjs-tx for developers who prefer a more streamlined API.
Eth-lib is a low-level library for Ethereum that provides utilities for creating and signing transactions, encoding and decoding data, and working with cryptographic primitives. It is similar to ethereumjs-tx in that it focuses on low-level operations, but it also includes additional utilities for working with Ethereum data structures.
A simple module for creating, manipulating and signing Ethereum transactions.
Scrollback or #ethereumjs on freenode
npm install ethereumjs-tx
var Tx = require('ethereumjs-tx');
var privateKey = new Buffer('e331b6d69882b4cb4ea581d88e0b604039a3de5967688d3dcffdd2270c0fd109', 'hex');
var rawTx = {
nonce: '00',
gasPrice: '09184e72a000',
gasLimit: '2710',
to: '0000000000000000000000000000000000000000',
value: '00',
data: '7f7465737432000000000000000000000000000000000000000000000000000000600057'
};
var tx = new Tx(rawTx);
tx.sign(privateKey);
var serializedTx = tx.serialize();
For standalone use in the browser inculde ./dist/ethereumjs-tx.js
This will give you a gobal varible EthTx
to use. It will also create the globals Buffer
and ethUtil
To build for standalone use in the browser install browserify
and run npm run build
.
new Transaction([data])
Transaction
PropertiesTransaction
Methodstransaction.serialize()
transaction.hash([signature])
transaction.sign(privateKey)
transaction.getSenderAddress()
transaction.getSenderPublicKey()
transaction.validate()
transaction.validateSignature()
transaction.getDataFee()
transaction.getBaseFee()
transaction.getUpfrontCost()
transaction.toJSON([object])
new Transaction([data])
Creates a new transaction object
data
- a transaction can be initiailized with either a buffer
containing the RLP serialized transaction or an array
of buffers relating to each of the tx Properties, listed in order below. For example.var rawTx = {
nonce: '00',
gasPrice: '09184e72a000',
gasLimit: '2710',
to: '0000000000000000000000000000000000000000',
value: '00',
data: '7f7465737432000000000000000000000000000000000000000000000000000000600057',
v: '1c',
r: '5e1d3a76fbf824220eafc8c79ad578ad2b67d01b0c2425eb1f1347e8f50882ab',
s '5bd428537f05f9830e93792f90ea6a3e2d1ee84952dd96edbae9f658f831ab13'
};
var tx = new Transaction(rawTx);
Or lastly an Object
containing the Properties of the transaction like in the Usage example
For Object
and Arrays
each of the elements can either be a Buffer
, hex String
, Number
, or an object with a toBuffer
method such as Bignum
transaction
Propertiesraw
- The raw rlp decoded transaction.nonce
to
- the to addressvalue
- the amount of ether sentdata
- this will contain the data
of the message or the init
of a contract.v
- EC signature parameterr
- EC signature parameters
- EC recovery IDTransaction
Methodstransaction.serialize()
Returns the RLP serialization of the transaction
Return: 32 Byte Buffer
transaction.hash([signature])
Returns the SHA3-256 hash of the rlp transaction
Parameters
signature
- a Boolean
determining if to include the signature components of the transaction. Defaults to true.Return: 32 Byte Buffer
transaction.sign(privateKey)
Signs the transaction with the given privateKey.
Parameters
privateKey
- a 32 Byte Buffer
transaction.getSenderAddress()
Returns the senders address
Return: 20 Byte Buffer
transaction.getSenderPublicKey()
returns the public key of the sender
Return: Buffer
transaction.validate()
Determines if the transaction is schematicly valid by checking its signature and gasCost.
Return: Boolean
transaction.validateSignature()
Determines if the signature is valid
Return: Boolean
transaction.getDataFee()
Returns the amount of gas to be paid for the data in this transaction
Return: bn.js
transaction.getBaseFee()
Returns the minimum amount of gas the tx must have (DataFee + TxFee)
Return: bn.js
transaction.getUpfrontCost()
The total amount needed in the account of the sender for the transaction to be valid
Return: bn.js
transaction.toJSON([object])
Returns transaction as JSON
Parameters
object
- a Boolean
that defaults to false. If object
is true then this will return an object else it will return an array
Return: Object
or Array
test uses mocha. To run
npm test
FAQs
A simple module for creating, manipulating and signing Ethereum transactions
The npm package ethereumjs-tx receives a total of 230,258 weekly downloads. As such, ethereumjs-tx popularity was classified as popular.
We found that ethereumjs-tx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.