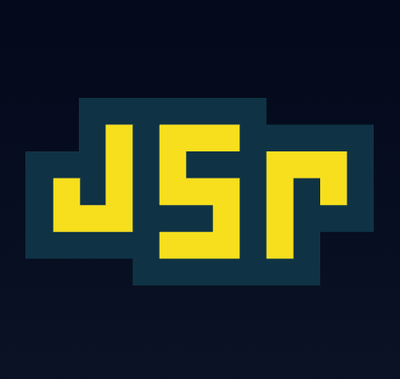
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
A complete and compact Ethereum library, for dapps, wallets and any other tools.
The ethers npm package is a library that provides a set of tools to interact with the Ethereum blockchain. It allows users to connect to the Ethereum network, manage wallets and keys, interact with smart contracts, and perform various other blockchain-related operations.
Connecting to Ethereum Network
This code sample demonstrates how to connect to the Ethereum network using ethers with an Infura provider.
const { ethers } = require('ethers');
const provider = new ethers.providers.JsonRpcProvider('https://mainnet.infura.io/v3/YOUR_INFURA_API_KEY');
Wallet Management
This code sample shows how to create a new wallet from a private key and connect it to an Ethereum provider.
const { ethers } = require('ethers');
const wallet = new ethers.Wallet('YOUR_PRIVATE_KEY');
const connectedWallet = wallet.connect(provider);
Interacting with Smart Contracts
This code sample illustrates how to interact with a smart contract by creating a contract instance and calling one of its functions.
const { ethers } = require('ethers');
const abi = [...] // Contract ABI
const contractAddress = '0x...'; // Contract address
const contract = new ethers.Contract(contractAddress, abi, provider);
const value = await contract.someFunction();
Sending Transactions
This code sample demonstrates how to send a transaction to the Ethereum network using a wallet instance.
const { ethers } = require('ethers');
const tx = {
to: '0x...',
value: ethers.utils.parseEther('1.0'),
gasLimit: 21000,
gasPrice: ethers.utils.parseUnits('10', 'gwei')
};
const sendPromise = wallet.sendTransaction(tx);
Querying Blockchain Data
This code sample shows how to query blockchain data, such as retrieving the latest block number and details of a specific block.
const { ethers } = require('ethers');
const blockNumber = await provider.getBlockNumber();
const block = await provider.getBlock(blockNumber);
Truffle Contract is part of the Truffle Suite and is designed to provide a more comfortable abstraction for interacting with Ethereum smart contracts. It is often used in combination with other Truffle tools for development, testing, and deployment. While it offers similar contract interaction capabilities, it is more tightly integrated with the Truffle development environment compared to ethers.
Drizzle is a collection of front-end libraries that make writing dApp front-ends easier and more predictable. It is part of the Truffle Suite and is designed to work with a Redux store. Drizzle provides reactive contract data fetching and transaction processing, which can be more convenient for dApp development. However, it is more opinionated and specific to front-end development compared to ethers, which is more general-purpose.
A complete, compact and simple library for Ethereum and ilk, written in TypeScript.
Features
For advisories and important notices, follow @ethersproject on Twitter (low-traffic, non-marketing, important information only) as well as watch this GitHub project.
For more general news, discussions, and feedback, follow or DM me, @ricmoo on Twitter or on the Ethers Discord.
For the latest changes, see the CHANGELOG.
Summaries
NodeJS
/home/ricmoo/some_project> npm install ethers
Browser (ESM)
The bundled library is available in the ./dist/
folder in this repo.
<script type="module">
import { ethers } from "./dist/ethers.min.js";
</script>
Browse the documentation online:
Ethers works closely with an ever-growing list of third-party providers to ensure getting started is quick and easy, by providing default keys to each service.
These built-in keys mean you can use ethers.getDefaultProvider()
and
start developing right away.
However, the API keys provided to ethers are also shared and are intentionally throttled to encourage developers to eventually get their own keys, which unlock many other features, such as faster responses, more capacity, analytics and other features like archival data.
When you are ready to sign up and start using for your own keys, please check out the Provider API Keys in the documentation.
A special thanks to these services for providing community resources:
The ethers
package only includes the most common and most core
functionality to interact with Ethereum. There are many other
packages designed to further enhance the functionality and experience.
MIT License (including all dependencies).
FAQs
A complete and compact Ethereum library, for dapps, wallets and any other tools.
The npm package ethers receives a total of 1,344,206 weekly downloads. As such, ethers popularity was classified as popular.
We found that ethers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.