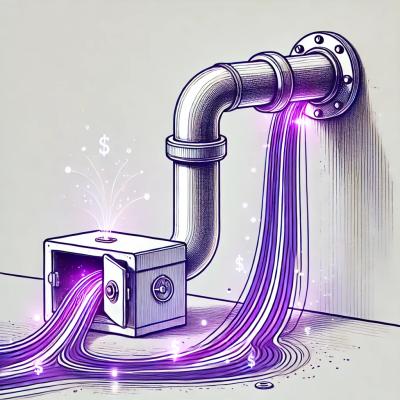
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
This is a simple library for implementing event subscription APIs.
const {Emitter} = require('event-kit')
class User {
constructor() {
this.emitter = new Emitter()
}
onDidChangeName(callback) {
this.emitter.on('did-change-name', callback)
}
setName(name) {
if (name !== this.name) {
this.name = name
this.emitter.emit('did-change-name', name)
}
return this.name
}
destroy() {
this.emitter.dispose()
}
}
In the example above, we implement ::onDidChangeName
on the user object, which
will register callbacks to be invoked whenever the user's name changes. To do
so, we make use of an internal Emitter
instance. We use ::on
to subscribe
the given callback in ::onDidChangeName
, and ::emit
in ::setName
to notify
subscribers. Finally, when the User
instance is destroyed we call ::dispose
on the emitter to unsubscribe all subscribers.
Emitter::on
returns a Disposable
instance, which has a ::dispose
method.
To unsubscribe, simply call dispose on the returned object.
const subscription = user.onDidChangeName((name) => console.log(`My name is ${name}`))
// Later, to unsubscribe...
subscription.dispose()
You can also use CompositeDisposable
to combine disposable instances together.
const {CompositeDisposable} = require('event-kit')
const subscriptions = new CompositeDisposable()
subscriptions.add(user1.onDidChangeName((name) => console.log(`User 1: ${name}`))
subscriptions.add(user2.onDidChangeName((name) => console.log(`User 2: ${name}`))
// Later, to unsubscribe from *both*...
subscriptions.dispose()
Disposables are convenient ways to represent a resource you will no longer need at some point. You can instantiate a disposable with an action to take when no longer needed.
const {Disposable} = require('event-kit')
const disposable = new Disposable(() => this.destroyResource())
FAQs
Simple library for implementing and consuming evented APIs
The npm package event-kit receives a total of 4,792 weekly downloads. As such, event-kit popularity was classified as popular.
We found that event-kit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.