eventproxy
Advanced tools
Comparing version 0.2.6 to 0.2.7
@@ -1,2 +0,2 @@ | ||
/*global exports */ | ||
/*global define*/ | ||
/*! | ||
@@ -6,7 +6,8 @@ * This file is used for define the EventProxy library. | ||
* @version 0.2.2 | ||
* ``` | ||
*/ | ||
;(function (name, definition) { | ||
// this is considered "safe": | ||
!(function (name, definition) { | ||
// Check define | ||
var hasDefine = typeof define === 'function', | ||
// hasDefine = typeof define === 'function', | ||
// Check exports | ||
hasExports = typeof module !== 'undefined' && module.exports; | ||
@@ -28,2 +29,8 @@ | ||
/** | ||
* refs | ||
*/ | ||
var SLICE = Array.prototype.slice; | ||
var CONCAT = Array.prototype.concat; | ||
/** | ||
* EventProxy. An implementation of task/event based asynchronous pattern. | ||
@@ -58,3 +65,2 @@ * A module that can be mixed in to *any object* in order to provide it with custom events. | ||
debug('Add listener for %s', ev); | ||
this._callbacks = this._callbacks || {}; | ||
this._callbacks[ev] = this._callbacks[ev] || []; | ||
@@ -65,11 +71,11 @@ this._callbacks[ev].push(callback); | ||
/** | ||
* `addListener` alias, bind | ||
* `addListener` alias, `bind` | ||
*/ | ||
EventProxy.prototype.bind = EventProxy.prototype.addListener; | ||
/** | ||
* `addListener` alias, on | ||
* `addListener` alias, `on` | ||
*/ | ||
EventProxy.prototype.on = EventProxy.prototype.addListener; | ||
/** | ||
* `addListener` alias, subscribe | ||
* `addListener` alias, `subscribe` | ||
*/ | ||
@@ -79,28 +85,39 @@ EventProxy.prototype.subscribe = EventProxy.prototype.addListener; | ||
/** | ||
* Remove one or many callbacks. If `callback` is null, removes all callbacks for the event. | ||
* If `ev` is null, removes all bound callbacks | ||
* for all events. | ||
* Bind an event, but put the callback into head of all callbacks. | ||
* @param {String} eventName Event name. | ||
* @param {Function} callback Callback. | ||
*/ | ||
EventProxy.prototype.removeListener = function (ev, callback) { | ||
var calls = this._callbacks, i, l; | ||
if (!ev) { | ||
EventProxy.prototype.headbind = function (ev, callback) { | ||
debug('Add listener for %s', ev); | ||
this._callbacks[ev] = this._callbacks[ev] || []; | ||
this._callbacks[ev].unshift(callback); | ||
return this; | ||
}; | ||
/** | ||
* Remove one or many callbacks. | ||
* | ||
* - If `callback` is null, removes all callbacks for the event. | ||
* - If `eventName` is null, removes all bound callbacks for all events. | ||
* @param {String} eventName Event name. | ||
* @param {Function} callback Callback. | ||
*/ | ||
EventProxy.prototype.removeListener = function (eventName, callback) { | ||
var calls = this._callbacks; | ||
if (!eventName) { | ||
debug('Remove all listeners'); | ||
this._callbacks = {}; | ||
} else if (calls) { | ||
} else { | ||
if (!callback) { | ||
ev!== 'all' && debug('Remove all listeners of %s', ev); | ||
calls[ev] = []; | ||
debug('Remove all listeners of %s', eventName); | ||
calls[eventName] = []; | ||
} else { | ||
var list = calls[ev]; | ||
if (!list) { | ||
return this; | ||
} | ||
l = list.length; | ||
for (i = 0; i < l; i++) { | ||
if (callback === list[i]) { | ||
ev!== 'all' && debug('Remove a listener of %s', ev); | ||
list[i] = null; | ||
break; | ||
var list = calls[eventName]; | ||
if (list) { | ||
var l = list.length; | ||
for (var i = 0; i < l; i++) { | ||
if (callback === list[i]) { | ||
debug('Remove a listener of %s', eventName); | ||
list[i] = null; | ||
break; | ||
} | ||
} | ||
@@ -145,6 +162,6 @@ } | ||
list.splice(i, 1); | ||
i--; | ||
i--; | ||
l--; | ||
} else { | ||
args = both ? Array.prototype.slice.call(arguments, 1) : arguments; | ||
args = both ? SLICE.call(arguments, 1) : arguments; | ||
callback.apply(this, args); | ||
@@ -166,2 +183,17 @@ } | ||
/** | ||
* Bind an event like the bind method, but will remove the listener after it was fired. | ||
* @param {String} ev Event name | ||
* @param {Function} callback Callback | ||
*/ | ||
EventProxy.prototype.once = function (ev, callback) { | ||
var self = this; | ||
var wrapper = function () { | ||
callback.apply(self, arguments); | ||
self.unbind(ev, wrapper); | ||
}; | ||
this.bind(ev, wrapper); | ||
return this; | ||
}; | ||
var later = typeof process !== 'undefined' && process.nextTick || function (fn) { | ||
@@ -184,17 +216,2 @@ setTimeout(fn, 0); | ||
/** | ||
* Bind an event like the bind method, but will remove the listener after it was fired. | ||
* @param {String} ev Event name | ||
* @param {Function} callback Callback | ||
*/ | ||
EventProxy.prototype.once = function (ev, callback) { | ||
var self = this; | ||
var wrapper = function () { | ||
callback.apply(self, arguments); | ||
self.unbind(ev, wrapper); | ||
}; | ||
this.bind(ev, wrapper); | ||
return this; | ||
}; | ||
/** | ||
* Bind an event, and trigger it immediately. | ||
@@ -216,5 +233,6 @@ * @param {String} ev Event name. | ||
var _assign = function (eventname1, eventname2, cb, once) { | ||
var proxy = this, length, index = 0, argsLength = arguments.length, | ||
bind, _all, | ||
callback, events, isOnce, times = 0, flag = {}; | ||
var proxy = this; | ||
var argsLength = arguments.length; | ||
var times = 0; | ||
var flag = {}; | ||
@@ -226,5 +244,5 @@ // Check the arguments length. | ||
events = Array.prototype.slice.apply(arguments, [0, argsLength - 2]); | ||
callback = arguments[argsLength - 2]; | ||
isOnce = arguments[argsLength - 1]; | ||
var events = SLICE.call(arguments, 0, -2); | ||
var callback = arguments[argsLength - 2]; | ||
var isOnce = arguments[argsLength - 1]; | ||
@@ -236,4 +254,3 @@ // Check the callback type. | ||
debug('Assign listener for events %j, once is %s', events, !!isOnce); | ||
length = events.length; | ||
bind = function (key) { | ||
var bind = function (key) { | ||
var method = isOnce ? "once" : "bind"; | ||
@@ -250,7 +267,8 @@ proxy[method](key, function (data) { | ||
for (index = 0; index < length; index++) { | ||
var length = events.length; | ||
for (var index = 0; index < length; index++) { | ||
bind(events[index]); | ||
} | ||
_all = function (event) { | ||
var _all = function (event) { | ||
if (times < length) { | ||
@@ -263,3 +281,3 @@ return; | ||
var data = []; | ||
for (index = 0; index < length; index++) { | ||
for (var index = 0; index < length; index++) { | ||
data.push(proxy._fired[events[index]].data); | ||
@@ -289,3 +307,3 @@ } | ||
EventProxy.prototype.all = function (eventname1, eventname2, callback) { | ||
var args = Array.prototype.concat.apply([], arguments); | ||
var args = CONCAT.apply([], arguments); | ||
args.push(true); | ||
@@ -308,3 +326,5 @@ _assign.apply(this, args); | ||
that.unbind(); | ||
callback(err); | ||
// put all arguments to the error handler | ||
// fail(function(err, args1, args2, ...){}) | ||
callback.apply(null, arguments); | ||
}); | ||
@@ -328,3 +348,3 @@ return this; | ||
EventProxy.prototype.tail = function () { | ||
var args = Array.prototype.concat.apply([], arguments); | ||
var args = CONCAT.apply([], arguments); | ||
args.push(false); | ||
@@ -355,4 +375,3 @@ _assign.apply(this, args); | ||
var proxy = this, | ||
firedData = [], | ||
all; | ||
firedData = []; | ||
this._after = this._after || {}; | ||
@@ -365,3 +384,3 @@ var group = eventName + '_group'; | ||
debug('After emit %s times, event %s\'s listenner will execute', times, eventName); | ||
all = function (name, data) { | ||
var all = function (name, data) { | ||
if (name === eventName) { | ||
@@ -413,3 +432,4 @@ times--; | ||
if (err) { | ||
return that.emit('error', err); | ||
// put all arguments to the error handler | ||
return that.emit.apply(that, ['error'].concat(SLICE.call(arguments))); | ||
} | ||
@@ -419,3 +439,3 @@ that.emit(group, { | ||
// callback(err, args1, args2, ...) | ||
result: callback ? callback.apply(null, Array.prototype.slice.call(arguments, 1)) : data | ||
result: callback ? callback.apply(null, SLICE.call(arguments, 1)) : data | ||
}); | ||
@@ -433,8 +453,4 @@ }; | ||
var proxy = this, | ||
index, | ||
_bind, | ||
len = arguments.length, | ||
callback = arguments[len - 1], | ||
events = Array.prototype.slice.apply(arguments, [0, len - 1]), | ||
count = events.length, | ||
callback = arguments[arguments.length - 1], | ||
events = SLICE.call(arguments, 0, -1), | ||
_eventName = events.join("_"); | ||
@@ -445,3 +461,3 @@ | ||
_bind = function (key) { | ||
var _bind = function (key) { | ||
proxy.bind(key, function (data) { | ||
@@ -453,3 +469,3 @@ debug('One of events %j emited, execute the listenner'); | ||
for (index = 0; index < count; index++) { | ||
for (var index = 0; index < events.length; index++) { | ||
_bind(events[index]); | ||
@@ -512,7 +528,8 @@ } | ||
if (err) { | ||
return that.emit('error', err); | ||
// put all arguments to the error handler | ||
return that.emit.apply(that, ['error'].concat(SLICE.call(arguments))); | ||
} | ||
// callback(err, args1, args2, ...) | ||
var args = Array.prototype.slice.call(arguments, 1); | ||
var args = SLICE.call(arguments, 1); | ||
@@ -525,3 +542,11 @@ if (typeof handler === 'string') { | ||
// })); | ||
return that.emit(handler, callback ? callback.apply(null, args) : data); | ||
if (callback) { | ||
// only replace the args when it really return a result | ||
return that.emit(handler, callback.apply(null, args)); | ||
} else { | ||
// put all arguments to the done handler | ||
//ep.done('some'); | ||
//ep.on('some', function(args1, args2, ...){}); | ||
return that.emit.apply(that, [handler].concat(args)); | ||
} | ||
} | ||
@@ -569,3 +594,3 @@ | ||
var ep = new EventProxy(); | ||
var args = Array.prototype.concat.apply([], arguments); | ||
var args = CONCAT.apply([], arguments); | ||
if (args.length) { | ||
@@ -578,3 +603,3 @@ var errorHandler = args[args.length - 1]; | ||
} | ||
ep.assign.apply(ep, Array.prototype.slice.call(args)); | ||
ep.assign.apply(ep, args); | ||
} | ||
@@ -581,0 +606,0 @@ return ep; |
@@ -29,3 +29,5 @@ { | ||
"scripts": { | ||
"test": "make test-all", | ||
"test": "make test-all" | ||
}, | ||
"config": { | ||
"blanket": { | ||
@@ -45,3 +47,3 @@ "pattern": "eventproxy/lib", | ||
}, | ||
"version": "0.2.6", | ||
"version": "0.2.7", | ||
"main": "index.js", | ||
@@ -48,0 +50,0 @@ "directories": { |
@@ -118,3 +118,3 @@ EventProxy [](http://travis-ci.org/JacksonTian/eventproxy) | ||
paths: { | ||
eventproxy: "https://raw.github.com/JacksonTian/eventproxy/master/lib/eventproxy.js" | ||
eventproxy: "https://raw.github.com/JacksonTian/eventproxy/master/lib/eventproxy" | ||
} | ||
@@ -121,0 +121,0 @@ }); |
@@ -123,3 +123,3 @@ EventProxy [](http://travis-ci.org/JacksonTian/eventproxy) [](http://badge.fury.io/js/eventproxy) [English Doc](https://github.com/JacksonTian/eventproxy/blob/master/README_en.md) | ||
paths: { | ||
eventproxy: "https://raw.github.com/JacksonTian/eventproxy/master/lib/eventproxy.js" | ||
eventproxy: "https://raw.github.com/JacksonTian/eventproxy/master/lib/eventproxy" | ||
} | ||
@@ -529,1 +529,5 @@ }); | ||
[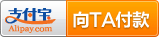](https://me.alipay.com/jacksontian) | ||
[](https://bitdeli.com/free "Bitdeli Badge") | ||
51004
573
532