eventproxy
Advanced tools
Comparing version 0.2.7 to 0.3.0
@@ -32,2 +32,3 @@ /*global define*/ | ||
var CONCAT = Array.prototype.concat; | ||
var ALL_EVENT = '__all__'; | ||
@@ -58,3 +59,3 @@ /** | ||
* Bind an event, specified by a string name, `ev`, to a `callback` function. | ||
* Passing `all` will bind the callback to all events fired. | ||
* Passing __ALL_EVENT__ will bind the callback to all events fired. | ||
* @param {String} eventName Event name. | ||
@@ -141,2 +142,16 @@ * @param {Function} callback Callback. | ||
/** | ||
* Bind the ALL_EVENT event | ||
*/ | ||
EventProxy.prototype.bindForAll = function (callback) { | ||
this.bind(ALL_EVENT, callback); | ||
}; | ||
/** | ||
* Unbind the ALL_EVENT event | ||
*/ | ||
EventProxy.prototype.unbindForAll = function (callback) { | ||
this.unbind(ALL_EVENT, callback); | ||
}; | ||
/** | ||
* Trigger an event, firing all bound callbacks. Callbacks are passed the | ||
@@ -154,3 +169,3 @@ * same arguments as `trigger` is, apart from the event name. | ||
while (both--) { | ||
ev = both ? eventName : 'all'; | ||
ev = both ? eventName : ALL_EVENT; | ||
list = calls[ev]; | ||
@@ -277,3 +292,3 @@ if (list) { | ||
if (isOnce) { | ||
proxy.unbind("all", _all); | ||
proxy.unbindForAll(_all); | ||
} | ||
@@ -283,3 +298,3 @@ debug('Events %j all emited with data %j', events, data); | ||
}; | ||
proxy.bind("all", _all); | ||
proxy.bindForAll(_all); | ||
}; | ||
@@ -379,3 +394,3 @@ | ||
debug('Event %s was emit %s, and execute the listenner', eventName, times); | ||
proxy.unbind("all", all); | ||
proxy.unbindForAll(all); | ||
callback.apply(null, [firedData]); | ||
@@ -389,3 +404,3 @@ } | ||
debug('Event %s was emit %s, and execute the listenner', eventName, times); | ||
proxy.unbind("all", all); | ||
proxy.unbindForAll(all); | ||
callback.call(null, proxy._after[group].results); | ||
@@ -395,3 +410,3 @@ } | ||
}; | ||
proxy.bind("all", all); | ||
proxy.bindForAll(all); | ||
return this; | ||
@@ -469,3 +484,3 @@ }; | ||
debug('Add listenner for not event %s', eventName); | ||
proxy.bind("all", function (name, data) { | ||
proxy.bindForAll(function (name, data) { | ||
if (name !== eventName) { | ||
@@ -472,0 +487,0 @@ debug('listenner execute of event %s emit, but not event %s.', name, eventName); |
@@ -17,12 +17,18 @@ { | ||
"dependencies": { | ||
"debug": "0.7.2" | ||
"debug": "0.7.4" | ||
}, | ||
"devDependencies": { | ||
"mocha": "*", | ||
"autod": "*", | ||
"blanket": "*", | ||
"chai": "1.9.0", | ||
"component": "*", | ||
"contributors": "*", | ||
"eventproxy": "0.2.7", | ||
"growl": "1.7.0", | ||
"jade": "1.3.0", | ||
"mocha": "1.17.1", | ||
"mocha-phantomjs": "*", | ||
"component": "*", | ||
"blanket": "*", | ||
"pedding": "*", | ||
"chai": "*", | ||
"travis-cov": "*" | ||
"pedding": "0.0.3", | ||
"totoro": "*", | ||
"cov": "*" | ||
}, | ||
@@ -47,3 +53,3 @@ "scripts": { | ||
}, | ||
"version": "0.2.7", | ||
"version": "0.3.0", | ||
"main": "index.js", | ||
@@ -50,0 +56,0 @@ "directories": { |
EventProxy [](http://travis-ci.org/JacksonTian/eventproxy) | ||
====== | ||
[](https://nodei.co/npm/eventproxy) | ||
> There is no deep nested callback issue in this world. —— [Jackson Tian](http://weibo.com/shyvo) | ||
@@ -135,3 +137,3 @@ | ||
// Executed when all specified events are fired. | ||
// Parameters corresponds with each event name | ||
// Parameters corresponds with each event name | ||
}); | ||
@@ -364,4 +366,4 @@ fs.readFile('template.tpl', 'utf-8', function (err, content) { | ||
### Asynchronous event emit: emitLater && doneLater | ||
In node, `emit` is a synchronous method, `emit`and `trigger` in EventProxy also are synchronous method like node. Look at code below, maybe you can find out what's wrong with it. | ||
### Asynchronous event emit: emitLater && doneLater | ||
In node, `emit` is a synchronous method, `emit`and `trigger` in EventProxy also are synchronous method like node. Look at code below, maybe you can find out what's wrong with it. | ||
@@ -396,3 +398,3 @@ ```js | ||
``` | ||
Just in case `callback` in `db.check` was synchronous execution, the `check` event will emit before `ep` listen `check`. Then the code won't work as we expect. Even though in node, we should keep all callback asynchronous execution, but we can't ensure everyone can accomplish. So we must write code like this: | ||
Just in case `callback` in `db.check` was synchronous execution, the `check` event will emit before `ep` listen `check`. Then the code won't work as we expect. Even though in node, we should keep all callback asynchronous execution, but we can't ensure everyone can accomplish. So we must write code like this: | ||
@@ -428,5 +430,5 @@ ```js | ||
We have to move `db.check` to the end of the code, to make sure `ep` listen all the events first. Then the code look like `get`->`render`->`check`, but the execution order is `check`->`get`->`render`, this kind of code is hard to understand. | ||
We have to move `db.check` to the end of the code, to make sure `ep` listen all the events first. Then the code look like `get`->`render`->`check`, but the execution order is `check`->`get`->`render`, this kind of code is hard to understand. | ||
So we need __Asynchronous event emit__: | ||
So we need __Asynchronous event emit__: | ||
@@ -462,4 +464,4 @@ ```js | ||
We use `emitLater` in `db.check` to emit an event. Then whatever `db.check` do, we make sure events emit by `db.check` will catch by `ep`. And our code is easy to understand. | ||
Also, we can use `doneLater` to simplify it just like what `ep.done()` do: | ||
We use `emitLater` in `db.check` to emit an event. Then whatever `db.check` do, we make sure events emit by `db.check` will catch by `ep`. And our code is easy to understand. | ||
Also, we can use `doneLater` to simplify it just like what `ep.done()` do: | ||
@@ -481,3 +483,3 @@ ```js | ||
``` | ||
This is a kind of really simple and understandability code style. | ||
This is a kind of really simple and understandability code style. | ||
@@ -497,3 +499,3 @@ ## Attentions | ||
files : 18 | ||
authors : | ||
authors : | ||
123 Jackson Tian 90.4% | ||
@@ -508,4 +510,4 @@ 6 fengmk2 4.4% | ||
## License | ||
## License | ||
[The MIT License](https://github.com/JacksonTian/eventproxy/blob/master/MIT-License). Please enjoy open source. |
EventProxy [](http://travis-ci.org/JacksonTian/eventproxy) [](http://badge.fury.io/js/eventproxy) [English Doc](https://github.com/JacksonTian/eventproxy/blob/master/README_en.md) | ||
====== | ||
[](https://nodei.co/npm/eventproxy) | ||
> 这个世界上不存在所谓回调函数深度嵌套的问题。 —— [Jackson Tian](http://weibo.com/shyvo) | ||
@@ -285,3 +287,3 @@ | ||
上述代码优化之后,业务开发者几乎不用关心异常处理了。代码量降低效果明显。 | ||
上述代码优化之后,业务开发者几乎不用关心异常处理了。代码量降低效果明显。 | ||
这里代码的转换,也许有开发者并不放心。其实秘诀在`fail`方法和`done`方法中。 | ||
@@ -385,3 +387,3 @@ | ||
在node中,`emit`方法是同步的,EventProxy中的`emit`,`trigger`等跟node的风格一致,也是同步的。看下面这段代码,可能眼尖的同学一下就发现了隐藏的bug: | ||
在node中,`emit`方法是同步的,EventProxy中的`emit`,`trigger`等跟node的风格一致,也是同步的。看下面这段代码,可能眼尖的同学一下就发现了隐藏的bug: | ||
```js | ||
@@ -416,3 +418,3 @@ var ep = EventProxy.create(); | ||
没错,万一`db.check`的`callback`被同步执行了,在`ep`监听`check`事件之前,它就已经被抛出来了,后续逻辑没办法继续执行。尽管node的约定是所有的`callback`都是需要异步返回的,但是如果这个方法是由第三方提供的,我们没有办法保证`db.check`的`callback`一定会异步执行,所以我们的代码通常就变成了这样: | ||
没错,万一`db.check`的`callback`被同步执行了,在`ep`监听`check`事件之前,它就已经被抛出来了,后续逻辑没办法继续执行。尽管node的约定是所有的`callback`都是需要异步返回的,但是如果这个方法是由第三方提供的,我们没有办法保证`db.check`的`callback`一定会异步执行,所以我们的代码通常就变成了这样: | ||
@@ -447,5 +449,5 @@ ```js | ||
``` | ||
我们被迫把`db.check`挪到最后,保证事件先被监听,再执行`db.check`。`check`->`get`->`render`的逻辑,在代码中看起来变成了`get`->`render`->`check`。如果整个逻辑更加复杂,这种风格将会让代码很难读懂。 | ||
我们被迫把`db.check`挪到最后,保证事件先被监听,再执行`db.check`。`check`->`get`->`render`的逻辑,在代码中看起来变成了`get`->`render`->`check`。如果整个逻辑更加复杂,这种风格将会让代码很难读懂。 | ||
这时候,我们需要的就是 __异步事件触发__: | ||
这时候,我们需要的就是 __异步事件触发__: | ||
@@ -480,4 +482,4 @@ ```js | ||
``` | ||
上面代码中,我们把`db.check`的回调函数中的事件通过`emitLater`触发,这样,就算`db.check`的回调函数被同步执行了,事件的触发也还是异步的,`ep`在当前事件循环中监听了所有的事件,之后的事件循环中才会去触发`check`事件。代码顺序将和逻辑顺序保持一致。 | ||
当然,这么复杂的代码,必须可以像`ep.done()`一样通过`doneLater`来解决: | ||
上面代码中,我们把`db.check`的回调函数中的事件通过`emitLater`触发,这样,就算`db.check`的回调函数被同步执行了,事件的触发也还是异步的,`ep`在当前事件循环中监听了所有的事件,之后的事件循环中才会去触发`check`事件。代码顺序将和逻辑顺序保持一致。 | ||
当然,这么复杂的代码,必须可以像`ep.done()`一样通过`doneLater`来解决: | ||
@@ -499,3 +501,3 @@ ```js | ||
``` | ||
最终呈现出来的,是一段简洁且清晰的代码。 | ||
最终呈现出来的,是一段简洁且清晰的代码。 | ||
@@ -512,17 +514,20 @@ | ||
project : eventproxy | ||
repo age : 1 year, 10 months | ||
active : 58 days | ||
commits : 136 | ||
files : 18 | ||
repo age : 2 years, 10 months | ||
active : 93 days | ||
commits : 191 | ||
files : 23 | ||
authors : | ||
123 Jackson Tian 90.4% | ||
6 fengmk2 4.4% | ||
4 dead-horse 2.9% | ||
1 haoxin 0.7% | ||
1 redky 0.7% | ||
1 yaoazhen 0.7% | ||
167 Jackson Tian 87.4% | ||
9 fengmk2 4.7% | ||
7 dead-horse 3.7% | ||
2 azrael 1.0% | ||
1 redky 0.5% | ||
1 Bitdeli Chef 0.5% | ||
1 yaoazhen 0.5% | ||
1 Ivan Yan 0.5% | ||
1 cssmagic 0.5% | ||
1 haoxin 0.5% | ||
``` | ||
## License | ||
## License | ||
@@ -535,5 +540,1 @@ [The MIT License](https://github.com/JacksonTian/eventproxy/blob/master/MIT-License)。请自由享受开源。 | ||
[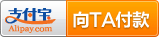](https://me.alipay.com/jacksontian) | ||
[](https://bitdeli.com/free "Bitdeli Badge") | ||
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
52288
9
586
533
13
+ Addeddebug@0.7.4(transitive)
- Removeddebug@0.7.2(transitive)
Updateddebug@0.7.4