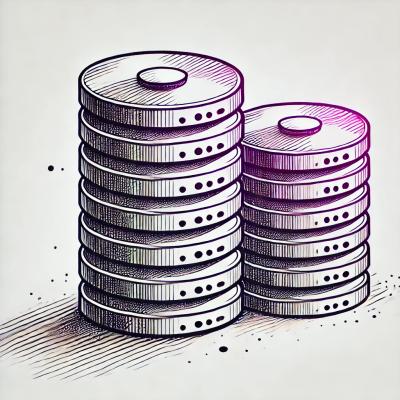
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
excel-formula-utilities
Advanced tools
This project is a port of the excel-formula library to ES6.
It contains a set of functions that can be used to pretty print Excel formulas and convert them into JavaScript, C# or Python code.
Key Differences from excel-formula:
isEu
as an option to the getTokens
, formatFormula
and formatFormulaHTML
methodsnpm install excel-formula-utilities
import { formatFormula } from 'excel-formula-utilities'
const formattedFormula = formatFormula('SUM(A1:A2)')
<script src="https://unpkg.com/excel-formula-utilities"></script>
<script>
const formattedFormula = ExcelFormulaUtilities.formatFormula('SUM(A1:A2)')
</script>
Formats an excel formula.
Signature:
formatFormula(formula: string, options): string
formula
- The excel formula to formatoptions
- An optional object with the following properties:Name | Description | Default |
---|---|---|
tmplFunctionStart | Template for the start of a function, the {{token}} will contain the name of the function. | '{{autoindent}}{{token}}(\n' |
tmplFunctionStop | Template for when the end of a function has been reached. | '\n{{autoindent}}{{token}})' |
tmplOperandError | Template for errors. | ' {{token}}' |
tmplOperandRange | Template for ranges and variable names. | '{{autoindent}}{{token}}' |
tmplLogical | Template for logical operators | '{{token}}{{autolinebreak}}' |
tmplOperandLogical | Template for logical operators such as + - = ... | '{{autoindent}}{{token}}' |
tmplOperandNumber | Template for numbers. | '{{autoindent}}{{token}}' |
tmplOperandText | Template for text/strings. | '{{autoindent}}"{{token}}"' |
tmplArgument | Template for argument separators such as ,. | '{{token}}\n' |
tmplOperandOperatorInfix | - | ' {{token}}{{autolinebreak}}' |
tmplFunctionStartArray | Template for the start of an array. | '' |
tmplFunctionStartArrayRow | Template for the start of an array row. | '{' |
tmplFunctionStopArrayRow | Template for the end of an array row. | '}' |
tmplFunctionStopArray | Template for the end of an array. | '' |
tmplSubexpressionStart | Template for the sub expression start. | '{{autoindent}}(\n' |
tmplSubexpressionStop | Template for the sub expression stop. | '\n)' |
tmplIndentTab | Template for the tab char. | '\t' |
tmplIndentSpace | Template for space char. | ' ' |
autoLineBreak | When rendering line breaks automatically which types should it break on. | 'TOK_TYPE_FUNCTION | TOK_TYPE_ARGUMENT | TOK_SUBTYPE_LOGICAL | TOK_TYPE_OP_IN' |
newLine | Used for the {{autolinebreak}} replacement as well as some string parsing. | '\n' |
trim | Trim the output. | true |
customTokenRender | This is a call back to a custom token function. | null |
prefix | Add a prefix to the formula. | '' |
postfix | Add a suffix to the formula. | '' |
isEu | If true then ; is treated as list separator, if false then ; is treated as array row separator | false |
Template Values
{{autoindent}}
- apply auto indent based on current tree level{{token}}
- the named token such as FUNCTION_NAME or "string"{{autolinebreak}}
- apply line break automatically. tests for next element only at this pointcustomTokenRender Example
function (tokenString, token, indent, lineBreak) {
const outStr = token
const useTemplate = true
// In the return object "useTemplate" tells formatFormula()
// weather or not to apply the template to what your return from the "tokenString".
return { tokenString: outStr, useTemplate }
}
Formats an excel formula into HTML.
Signature:
formatFormulaHTML(formula: string, options): string
formula
- The excel formula to formatoptions
- An optional object with the following properties (inherits defaults from formatFormula
):Name | Description | Default |
---|---|---|
tmplFunctionStart | Template for the start of a function, the {{token}} will contain the name of the function. | '{{autoindent}}<span class="function">{{token}}</span><span class="function_start">(</span><br />' |
tmplFunctionStop | Template for when the end of a function has been reached. | '<br />{{autoindent}}{{token}}<span class="function_stop">)</span>' |
tmplOperandError | Template for errors. | ' {{token}}' |
tmplOperandRange | Template for ranges and variable names. | '{{autoindent}}{{token}}' |
tmplLogical | Template for logical operators | '{{token}}{{autolinebreak}}' |
tmplOperandLogical | Template for logical operators such as + - = ... | '{{autoindent}}{{token}}' |
tmplOperandNumber | Template for numbers. | '{{autoindent}}{{token}}' |
tmplOperandText | Template for text/strings. | '{{autoindent}}<span class="quote_mark">"</span><span class="text">{{token}}</span><span class="quote_mark">"</span>' |
tmplArgument | Template for argument separators such as ,. | '{{token}}<br />' |
tmplOperandOperatorInfix | - | ' {{token}}{{autolinebreak}}' |
tmplFunctionStartArray | Template for the start of an array. | '' |
tmplFunctionStartArrayRow | Template for the start of an array row. | '{' |
tmplFunctionStopArrayRow | Template for the end of an array row. | '}' |
tmplFunctionStopArray | Template for the end of an array. | '' |
tmplSubexpressionStart | Template for the sub expression start. | '{{autoindent}}(' |
tmplSubexpressionStop | Template for the sub expression stop. | ' )' |
tmplIndentTab | Template for the tab char. | '<span class="tabbed"> </span>' |
tmplIndentSpace | Template for space char. | ' ' |
autoLineBreak | When rendering line breaks automatically which types should it break on. | 'TOK_TYPE_FUNCTION | TOK_TYPE_ARGUMENT | TOK_SUBTYPE_LOGICAL | TOK_TYPE_OP_IN ' |
newLine | Used for the {{autolinebreak}} replacement as well as some string parsing. | '<br />' |
trim | Trim the output. | true |
customTokenRender | This is a call back to a custom token function. | Custom function for formatFormulaHTML |
prefix | Add a prefix to the formula. | '=' |
postfix | Add a suffix to the formula. | '' |
Converts an excel formula into C# code.
Signature:
formula2CSharp(formula: string, options): string
formula
- The excel formula to formatoptions
- An optional object with the following properties (inherits defaults from formatFormula
):Name | Description | Default |
---|---|---|
tmplFunctionStart | Template for the start of a function, the {{token}} will contain the name of the function. | '{{token}}(' |
tmplFunctionStop | Template for when the end of a function has been reached. | '{{token}})' |
tmplOperandError | Template for errors. | '{{token}}' |
tmplOperandRange | Template for ranges and variable names. | '{{token}}' |
tmplOperandLogical | Template for logical operators such as + - = ... | '{{token}}' |
tmplOperandNumber | Template for numbers. | '{{token}}' |
tmplOperandText | Template for text/strings. | '"{{token}}"' |
tmplArgument | Template for argument separators such as ,. | '{{token}}' |
tmplOperandOperatorInfix | - | '{{token}}' |
tmplFunctionStartArray | Template for the start of an array. | '' |
tmplFunctionStartArrayRow | Template for the start of an array row. | '{' |
tmplFunctionStopArrayRow | Template for the end of an array row. | '}' |
tmplFunctionStopArray | Template for the end of an array. | '' |
tmplSubexpressionStart | Template for the sub expression start. | '(' |
tmplSubexpressionStop | Template for the sub expression stop. | ')' |
tmplIndentTab | Template for the tab char. | '\t' |
tmplIndentSpace | Template for space char. | ' ' |
autoLineBreak | When rendering line breaks automatically which types should it break on. | 'TOK_SUBTYPE_STOP | TOK_SUBTYPE_START | TOK_TYPE_ARGUMENT' |
trim | Trim the output. | true |
customTokenRender | This is a call back to a custom token function. | Custom function for formula2CSharp |
Signature:
formula2JavaScript(formula: string, options): string
formula
- The excel formula to formatoptions
- An optional object with the following properties (inherits options from formula2CSharp
):Signature:
formula2Python(formula: string, options): string
formula
- The excel formula to formatoptions
- An optional object with the following properties (inherits defaults from formatFormula
):Name | Description | Default |
---|---|---|
tmplFunctionStart | Template for the start of a function, the {{token}} will contain the name of the function. | '{{token}}(' |
tmplFunctionStop | Template for when the end of a function has been reached. | '{{token}})' |
tmplOperandError | Template for errors. | '{{token}}' |
tmplOperandRange | Template for ranges and variable names. | '{{token}}' |
tmplOperandLogical | Template for logical operators such as + - = ... | '{{token}}' |
tmplOperandNumber | Template for numbers. | '{{token}}' |
tmplOperandText | Template for text/strings. | '"{{token}}"' |
tmplArgument | Template for argument separators such as ,. | '{{token}}' |
tmplOperandOperatorInfix | - | '{{token}}' |
tmplFunctionStartArray | Template for the start of an array. | '' |
tmplFunctionStartArrayRow | Template for the start of an array row. | '{' |
tmplFunctionStopArrayRow | Template for the end of an array row. | '}' |
tmplFunctionStopArray | Template for the end of an array. | '' |
tmplSubexpressionStart | Template for the sub expression start. | '(' |
tmplSubexpressionStop | Template for the sub expression stop. | ')' |
tmplIndentTab | Template for the tab char. | '\t' |
tmplIndentSpace | Template for space char. | ' ' |
autoLineBreak | When rendering line breaks automatically which types should it break on. | 'TOK_SUBTYPE_STOP | TOK_SUBTYPE_START | TOK_TYPE_ARGUMENT' |
trim | Trim the output. | true |
customTokenRender | This is a call back to a custom token function. | Custom function for formula2CSharp |
Tokenizes an excel formula.
Signature:
getTokens(formula: string isEu: boolean): F_token[]
formula
- The excel formula to formatisEu
- If true
then ;
is treated as list separator, if false
then ;
is treated as array row separatorReturns an array of tokens, e.g. given the formula A1+1000
the output would be:
[
{
"subtype": "range",
"type": "operand",
"value": "A1"
},
{
"subtype": "math",
"type": "operator-infix",
"value": "+"
},
{
"subtype": "number",
"type": "operand",
"value": "1000"
}
]
FAQs
Utilities for formatting Excel formulas.
The npm package excel-formula-utilities receives a total of 1 weekly downloads. As such, excel-formula-utilities popularity was classified as not popular.
We found that excel-formula-utilities demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.