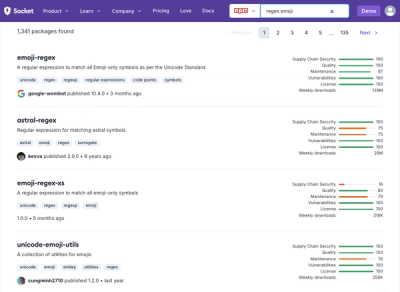
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
executes a step in a series with console feedback
import { ExecStepContext } from "exec-step";
function takesAWhile() {
return new Promise(resolve => setTimeout(resolve, 5000));
}
function isQuick() {
// does nothing!
}
(async () => {
const ctx = new ExecStepContext();
ctx.exec("please wait", takesAWhile);
ctx.exec("done quickly!", isQuick);
})();
construct with optional options of the shape:
export interface ExecStepConfiguration {
asciiPrefixes?: boolean;
prefixes?: PartialStepConfig<string>;
colors?: PartialStepConfig<string>;
throwErrors?: boolean;
dumpErrorStacks?: boolean;
}
defaults are:
const defaultConfig: ExecStepConfiguration = {
colors: {
wait: "yellowBright",
ok: "greenBright",
fail: "redBright"
},
throwErrors: true,
dumpErrorStacks: false
};
by default, exec-step reports wait/ok/fail status with utf-8 characters:
const utf8Prefixes: PartialStepConfig<string> = {
wait: "⌛",
ok: "✔",
fail: "✖"
};
but can use ascii -- either via the prefixes
part of the config, or by
observing the environment variable ASCII_STEP_MARKERS
for a truthy value
like 1
or true
-- useful at CI. If that's set, you get the following prefixes:
const asciiPrefixes: PartialStepConfig<string> = {
wait: "[ WAIT ]",
ok: "[ OK ]",
fail: "[ FAIL ]"
}
by default, exec-step will print out the task label with the failure marker of your choosing and re-throw the error, however, you can take complete control by handling errors within your task and throwing an ExecStepOverrideMessage error, eg:
await ctx.exec("do the thing", async () => {
try {
await attemptToDoTheThing();
} catch (e) {
throw new ExecStepOverrideMessage(
// overrides the error label
`Error whilst attempting to do the thing: ${e.message}`,
// original error, rethrown if allowed
e,
// suppress the error being thrown with false, or
// set this true to rethrow the original error
// if the context's default behavior is to throw
false
);
}
});
FAQs
executes a step in a series with console feedback
The npm package exec-step receives a total of 1,681 weekly downloads. As such, exec-step popularity was classified as popular.
We found that exec-step demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.